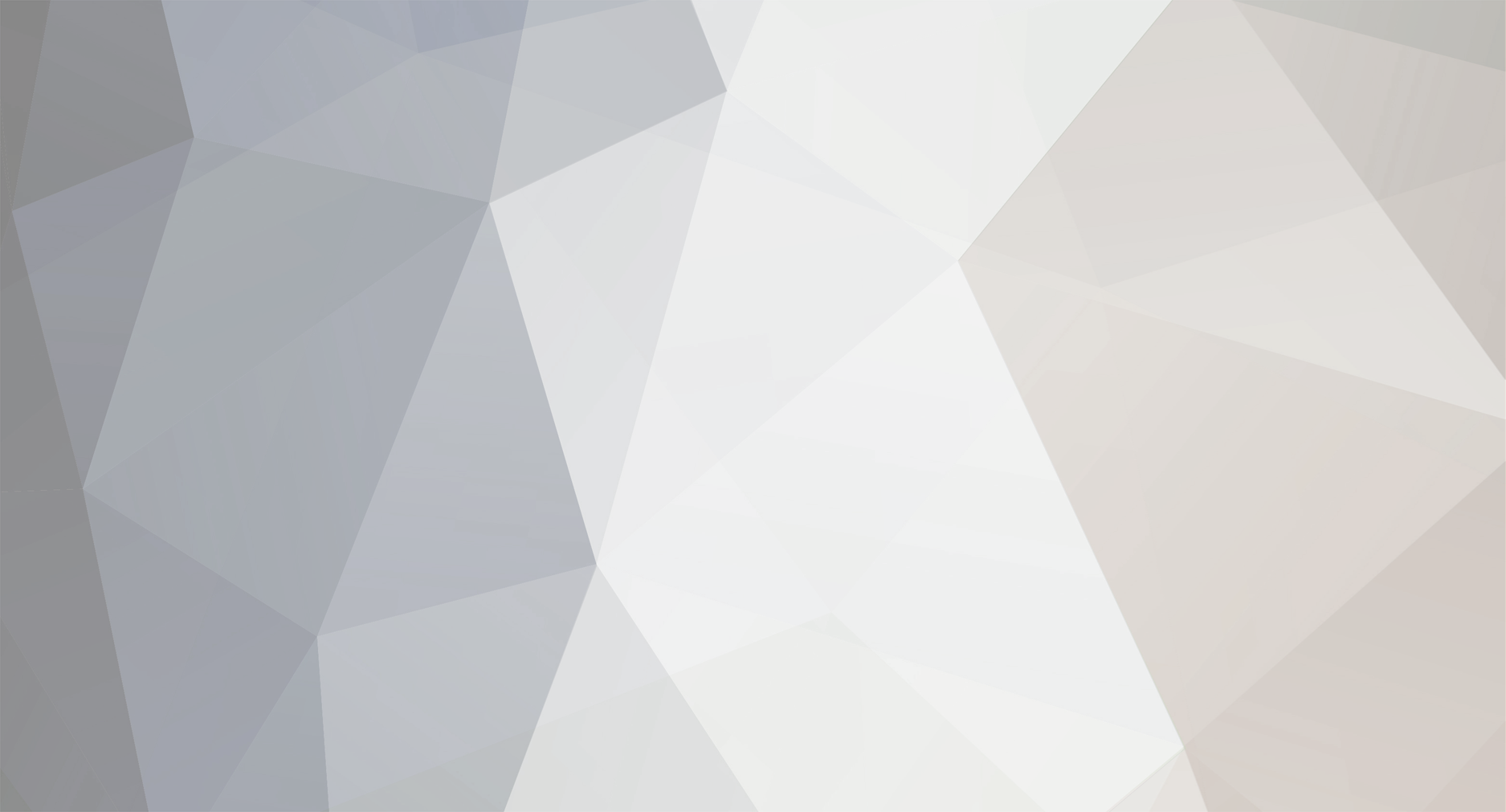
myonlake
Members-
Posts
2,312 -
Joined
-
Days Won
41
Everything posted by myonlake
-
Seems it does save the position but the camera is not faded in. Add the following line of code after setCameraTarget. fadeCamera(source, true) Full script would now look like this: local randomSpawnTable = { { x = 270.92654418945, y = -1986.3665771484, z = 797.52966308594, rotation = 0, model = 312, interior = 0, dimension = 0 }, { x = 270.92654418945, y = -1986.3665771484, z = 797.52966308594 }, { x = 270.92654418945, y = -1986.3665771484, z = 797.52966308594 } } -- Once the player logs in, let's spawn them to their saved position or a random spawnpoint addEventHandler("onPlayerLogin", root, function(_, account) -- Get the saved x, y, z position from the account data local x, y, z = getAccountData(account, "posX"), getAccountData(account, "posY"), getAccountData(account, "posZ") -- Get the saved rotation from the account data and default to 0 local rotation = getAccountData(account, "rotation") or 0 -- Get the saved model (skin) from the account data and default to 312 local model = getAccountData(account, "model") or 312 -- Get the saved interior from the account data and default to 0 local interior = getAccountData(account, "interior") or 0 -- Get the saved dimension from the account data and default to 0 local dimension = getAccountData(account, "dimension") or 0 -- If account data was missing any of the coordinates if (not x) or (not y) or (not z) then -- Get a random spawnpoint from the spawnpoint table local randomSpawnPoint = randomSpawnTable[math.random(#randomSpawnTable)] -- Overwrite coordinates and other details with spawnpoint details or fall back to the data above x, y, z = randomSpawnPoint.x or 0, randomSpawnPoint.y or 0, randomSpawnPoint.z or 3 rotation = randomSpawnPoint.rotation or rotation model = randomSpawnPoint.model or model interior = randomSpawnPoint.interior or interior dimension = randomSpawnPoint.dimension or dimension end -- Spawn the player to the location spawnPlayer(source, x, y, z, rotation, model, interior, dimension) -- Give weapons giveWeapon(source, 46) giveWeapon(source, 9) giveWeapon(source, 25, 5000) giveWeapon(source, 28, 5000) giveWeapon(source, 31, 5000) giveWeapon(source, 22, 5000, true) -- Reset their camera target and fade the camera in setCameraTarget(source) fadeCamera(source, true) end) -- Let's create a new savePlayer function for general use function savePlayer(player, account) -- Make sure we have passed in an element if (isElement(player)) then -- Get the player's account account = account or getPlayerAccount(player) -- If the player has an account if (account) then -- Get the player element position local x, y, z = getElementPosition(player) -- Get the player element rotation local _, _, rotation = getElementRotation(player) -- Set the account data setAccountData(account, "posX", x) setAccountData(account, "posY", y) setAccountData(account, "posZ", z) setAccountData(account, "rotation", rotation) setAccountData(account, "model", getElementModel(player)) setAccountData(account, "interior", getElementInterior(player)) setAccountData(account, "dimension", getElementDimension(player)) return true end end return false end -- Once the player logs out, let's save the player to their old account addEventHandler("onPlayerLogout", root, function(account) savePlayer(source, account) end) -- Once the player disconnects, let's log them out (which triggers savePlayer) addEventHandler("onPlayerQuit", root, function() logOut(source) end)
-
Make sure you load the file in meta.xml too. <file src="hello.png" />
-
Yes? Well, you need to spawn the player somewhere if they don't have any old position on their account? That script works fine for spawning the player to a random spawnpoint and then saves their position and spawns them to their old location when they come back another day and log in to the server.
-
You need to start the admin resource to get the flag image.
-
I knew this was going to be a problem sooner than later with the resource. The scoreboard resource uses the admin resource to get the player's country (and flag images). At least the latest version does. Do you have the admin resource somewhere in the resources folder?
-
And don't doublepost.
- 2 replies
-
- a temp
- a boolean value
-
(and 4 more)
Tagged with:
-
[QUESTION] setElementRotation and getCameraMatrix.
myonlake replied to Exilepilot's topic in Scripting
Sorry, perhaps findRotation3D helps you in this case better. Wait, so you're essentially just wanting the camera to point forward? -
[QUESTION] setElementRotation and getCameraMatrix.
myonlake replied to Exilepilot's topic in Scripting
You can use the findRotation function to do that. -
You could use the following functions to make such a feature. -- To create a command handler "tpme" and "tpto" addCommandHandler -- Create an ipairs loop using these functions getAlivePlayers -- To get all alive players, for /tpme getElementsByType -- To get all elements of type "player", for /tpto getPlayerName -- To get the iterated player's name and make a partial match with the name entered to the command arguments -- Upon match getElementPosition -- To get your/found player's position getElementInterior -- To get your/found player's interior getElementDimension -- To get your/found player's dimension setElementPosition -- To teleport you/found player to the coordinates setElementInterior -- To teleport you/found player to the interior setElementDimension -- To teleport you/found player to the dimension
-
Which version of the dxscoreboard are you using at the moment? There have been various changes to the dxscoreboard (now integrated into the default scoreboard resource) and you can download the latest version from MTA's official mtasa-resources GitHub repository at https://github.com/multitheftauto/mtasa-resources. The scoreboard resource can be found at https://github.com/multitheftauto/mtasa-resources/tree/master/[gameplay]/scoreboard.
- 1 reply
-
- 1
-
-
Do you mean the chatbox T or Y menu opens? That's MTA default functionality. You can disable the chatbox with showChat.
-
I worked on an idea like this a long time ago, managed to create synchronized sectors and mobs with some basic skills and mobility. Good luck for this project, I don't think I have time to participate though.
-
Use account data to store player element position and then spawn the player element at that saved position upon login. setAccountData getAccountData Such as... local randomSpawnTable = { { x = 270.92654418945, y = -1986.3665771484, z = 797.52966308594, rotation = 0, model = 312, interior = 0, dimension = 0 }, { x = 270.92654418945, y = -1986.3665771484, z = 797.52966308594 }, { x = 270.92654418945, y = -1986.3665771484, z = 797.52966308594 } } -- Once the player logs in, let's spawn them to their saved position or a random spawnpoint addEventHandler("onPlayerLogin", root, function(_, account) -- Get the saved x, y, z position from the account data local x, y, z = getAccountData(account, "posX"), getAccountData(account, "posY"), getAccountData(account, "posZ") -- Get the saved rotation from the account data and default to 0 local rotation = getAccountData(account, "rotation") or 0 -- Get the saved model (skin) from the account data and default to 312 local model = getAccountData(account, "model") or 312 -- Get the saved interior from the account data and default to 0 local interior = getAccountData(account, "interior") or 0 -- Get the saved dimension from the account data and default to 0 local dimension = getAccountData(account, "dimension") or 0 -- If account data was missing any of the coordinates if (not x) or (not y) or (not z) then -- Get a random spawnpoint from the spawnpoint table local randomSpawnPoint = randomSpawnTable[math.random(#randomSpawnTable)] -- Overwrite coordinates and other details with spawnpoint details or fall back to the data above x, y, z = randomSpawnPoint.x or 0, randomSpawnPoint.y or 0, randomSpawnPoint.z or 3 rotation = randomSpawnPoint.rotation or rotation model = randomSpawnPoint.model or model interior = randomSpawnPoint.interior or interior dimension = randomSpawnPoint.dimension or dimension end -- Spawn the player to the location spawnPlayer(source, x, y, z, rotation, model, interior, dimension) -- Reset their camera target setCameraTarget(source) -- Give weapons giveWeapon(source, 46) giveWeapon(source, 9) giveWeapon(source, 25, 5000) giveWeapon(source, 28, 5000) giveWeapon(source, 31, 5000) giveWeapon(source, 22, 5000, true) end) -- Let's create a new savePlayer function for general use function savePlayer(player, account) -- Make sure we have passed in an element if (isElement(player)) then -- Get the player's account account = account or getPlayerAccount(player) -- If the player has an account if (account) then -- Get the player element position local x, y, z = getElementPosition(player) -- Get the player element rotation local _, _, rotation = getElementRotation(player) -- Set the account data setAccountData(account, "posX", x) setAccountData(account, "posY", y) setAccountData(account, "posZ", z) setAccountData(account, "rotation", rotation) setAccountData(account, "model", getElementModel(player)) setAccountData(account, "interior", getElementInterior(player)) setAccountData(account, "dimension", getElementDimension(player)) return true end end return false end -- Once the player logs out, let's save the player to their old account addEventHandler("onPlayerLogout", root, function(account) savePlayer(source, account) end) -- Once the player disconnects, let's log them out (which triggers savePlayer) addEventHandler("onPlayerQuit", root, function() logOut(source) end)
-
I don't know what you mean exactly, and I don't know how to help since I don't have the full code. Also, create a new topic for that question. Let's keep this on topic.
-
Then attach it to the finish marker? And set the rewardAmount to math.random(500) if that's what you want. If you are using the base race resource, then you can just use the following snippet. -- Add an event handler for onPlayerFinish addEventHandler("onPlayerFinish", root, function(rank, time) -- Calculate some reward amount down from 500 local rewardAmount = 500 / rank -- Give the calculated reward amount to the player givePlayerMoney(source, rewardAmount) end) Why don't people search on Google or Wiki first? https://wiki.multitheftauto.com/wiki/Resource:Race
-
Click open the "Browse Data" tab in the window. Seriously... try to learn to use the software and not ask the most basic questions.
-
You can perhaps use a hit counter to calculate some progressive reward amount, goes to infinity in this example. -- Create a marker local marker = createMarker(0, 0, 3) -- Add an event handler for onPlayerMarkerHit on the marker addEventHandler("onPlayerMarkerHit", marker, function(markerHit, matchingDimension) -- If the player element is in the same dimension as the marker if (matchingDimension) then -- Get the hit count (or default to 0) from the marker local markerHitCount = tonumber(getElementData(markerHit, "hitCount") or 0) -- Calculate some reward amount down from 500 local rewardAmount = 500 / (markerHitCount + 1) -- Give the calculated reward amount to the player givePlayerMoney(source, rewardAmount) -- Increment the hit count of the marker setElementData(markerHit, "hitCount", markerHitCount + 1) end end) To reset the hit counter, just do the following: removeElementData(marker, "hitCount")
-
Like explained in that other topic by Solidsnake14, you can use it to read and write data to sqlite databases, like registry.db and internal.db, which are explained in the Database article I linked in my previous reply. GitHub repository: https://github.com/sqlitebrowser/sqlitebrowser Downloads: https://github.com/sqlitebrowser/sqlitebrowser/releases
-
Read more on the wiki Database article. Another question has already been posted about this:
-
You're welcome!
-
I need to see more code in order to be able to point out the problem.
-
Something like this should do the trick. -- Start by setting the interiorId variable to the player element's current interior ID local interiorId = getElementInterior(localPlayer) addEventHandler("onClientRender", root, function() -- The the player element's current interior ID local currentInteriorId = getElementInterior(localPlayer) -- Compare if the last saved interior ID has changed by the current player element interior ID if (interiorId ~= currentInteriorId) then -- Trigger a client-side event "onClientPlayerInteriorChanged" -- addEventHandler("onClientPlayerInteriorChanged", root, function(oldInteriorId, newInteriorId) --[[ your code ]] end) triggerEvent("onClientPlayerInteriorChanged", localPlayer, interiorId, currentInteriorId) -- Save the new interior ID to the variable interiorId = currentInteriorId end end) Tested and working using the following snippet: addEvent("onClientPlayerInteriorChanged") addEventHandler("onClientPlayerInteriorChanged", localPlayer, function(oldInteriorId, newInteriorId) -- Bound to only the localPlayer element in this example outputChatBox(oldInteriorId .. " => " .. newInteriorId) end) addCommandHandler("int", function(_, interiorId) setElementInterior(localPlayer, math.max(0, math.min(255, tonumber(interiorId) or 0))) end) You can implement this further by doing a full scan over a table of elements, such as all players, and triggering that event whenever someone changes their interior. Right now it only checks the local player.
-
There's a couple ways you can do this. a) A render loop and see if the interior has changed in a frame by storing the current interior ID into a variable outside of the render loop, and then next frame checking if it has changed, triggering an event, and then overwriting it with the new interior ID. Heavy way of doing a check, and it's only client-side, hence insecure. Just works doesn't matter which resource sets the interior. b) Wrap the setElementInterior function with your own function and trigger an event or something when it's called. Perhaps more lightweight compared to a render loop. Can be implemented both client and server-side (with server-side validation, more secure). Requires you to implement this wrapper function everywhere in your code, so it's a bit of a pain if you use third-party scripts a lot.