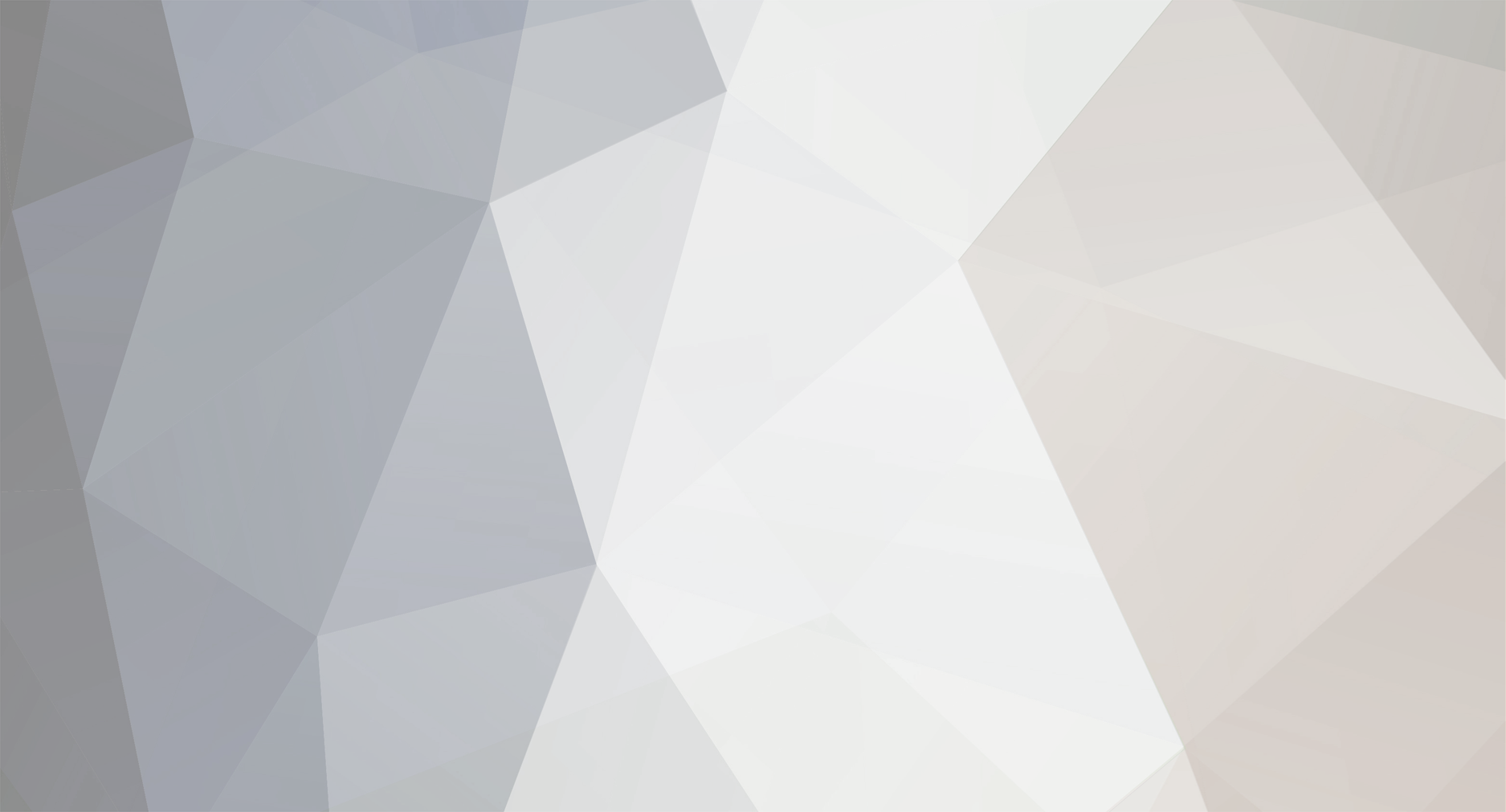
myonlake
Members-
Posts
2,312 -
Joined
-
Days Won
41
Everything posted by myonlake
-
I'm quite sure that's not your script because 1. it doesn't output anything, 2. it's not the full script, 3. g_Me, g_Rockets and those semicolons are a signature of a scripter I know. This is an old script. Please provide more information (and a link to the resource).
-
You have a two errors over there: not closing the function scope and using else when there is no if-scope to else (you shouldn't close the if-statement before the else). So simply move the end after the else scope, and remember to end the function as well. addEventHandler("onClientVehicleCollision",getRootElement(), -- function scope starts function (theHitElement) -- if-statement scope starts if theHitElement == nil then setVehicleDamageProof (source, true) else setVehicleDamageProof (source, false) end -- if-statement scope ends end -- function scope ends ) You can also do the same thing with less code: addEventHandler( "onClientVehicleCollision", root, function( theHitElement ) setVehicleDamageProof( source, not theHitElement ) end ) Do note, that doing any of this will make the vehicle damage proof to any damage from the world at any given time. So it'll not only be protected from jump damage, but also crashing into light poles and buildings and so on. Just so you know.
-
No.
-
Alright, I managed to figure it out for you. I also included the OOP version. So, I found out attachElements doesn't have the custom order parameter which allows you to set the order of the rotation. The expected rotation order would be ZYX, in OOP this is not the case however. I am not entirely sure why this is, it might be because of some simple rule but I just don't realize it right now... maybe it's written somewhere in the depths of the Wiki, but I cba to find it right now. I changed some of the parameters for the addVehicleCustomWheel function to allow tilt and scale upon call. I also added a command /customwheels so you can try it out by typing /customwheels [tilt] [scale] Anyway, have fun, either one works, whichever you like the best. I also cleaned up and commented the code just because people might be interested in this, so it's better if this is cleaned up for future reference. For you specifically, the fix would be to look into the calculateVehicleWheelRotation function, which shows how I've attached the custom wheel to the vehicle. Those contents should replace your attachElements, because attachElements doesn't work, you have to attach manually with position and rotation... Client-side -- Table containing vehicles that have custom wheels local createdCustomWheels = { } --[[ /customwheels [ number tilt, number scale ] Adds custom wheels to your currently occupied vehicle and optionally applies a tilt angle and wheel scale. ]] addCommandHandler( 'customwheels', function( _, tilt, scale ) -- Let's get our vehicle local vehicle = getPedOccupiedVehicle( localPlayer ) -- Oh, we have a vehicle! if ( vehicle ) then -- Let's give it a wheel now addVehicleCustomWheel( vehicle, 1075, tilt, scale ) end end ) -- Let's bind that command to F2 as requested by OP bindKey( 'f2', 'down', 'customwheels' ) --[[ void addVehicleCustomWheel ( vehicle vehicle, number model [ , number tilt, number scale ] ) Adds custom wheels to the vehicle. ]] function addVehicleCustomWheel( vehicle, model, tilt, scale ) -- If we've given the object's model number if ( tonumber( model ) ) then -- Let's delete any old ones for _, wheel in pairs( createdCustomWheels[ vehicle ] or { } ) do destroyElement( wheel.object ) end -- And let's nil it to be clear createdCustomWheels[ vehicle ] = nil -- Let's set some component names we want to customize local wheels = { 'wheel_lf_dummy', 'wheel_rf_dummy' } -- Let's iterate through those components for i = 1, #wheels do -- Let's hide the existing component setVehicleComponentVisible( vehicle, wheels[ i ], false ) -- Initialize our wheel table local wheel = { tilt = tonumber( tilt ) or 0, -- We'll default a non-number tilt angle to 0 name = wheels[ i ], -- Let's store the component name object = createObject( model, Vector3( ) ) -- Let's make a new wheel object } -- Let's set the vehicle as the wheel object's parent setElementParent( wheel.object, vehicle ) -- Let's make sure the wheel is not colliding with the vehicle setElementCollidableWith( wheel.object, vehicle, false ) -- Let's change the scale setObjectScale( wheel.object, tonumber( scale ) or 0.7 ) -- Let's update that original table wheels[ i ] = wheel end -- And let's store all of those wheels now createdCustomWheels[ vehicle ] = wheels end end --[[ void calculateVehicleWheelRotation ( vehicle vehicle, table wheel ) Let's calculate the wheel rotation for given wheel(s). ]] function calculateVehicleWheelRotation( vehicle, wheel ) -- If we have many wheels in the given argument if ( type( wheel ) == 'table' ) and ( #wheel > 0 ) then -- Let's iterate through them all for i = 1, #wheel do -- Let's call the method alone with just the iterator calculateVehicleWheelRotation( vehicle, wheel[ i ] ) end -- Let's stop here now return end -- If we have an object if ( wheel.object ) then -- Let's get the rotation vector of the original component local rotation = Vector3( getVehicleComponentRotation( vehicle, wheel.name, 'world' ) ) -- Let's set our tilt angle rotation.y = wheel.tilt -- Let's make sure the wheel is at the original component's position setElementPosition( wheel.object, Vector3( getVehicleComponentPosition( vehicle, wheel.name, 'world' ) ) ) -- Let's finally set the rotation (in ZYX order, important!) setElementRotation( wheel.object, rotation, "ZYX" ) end end -- Render-time! addEventHandler( 'onClientPreRender', root, function( ) -- Let's iterate through all the vehicles for vehicle, wheels in pairs( createdCustomWheels ) do -- If we have a vehicle, it's streamed in and it has wheels if ( vehicle ) and ( isElementStreamedIn( vehicle ) ) and ( #wheels > 0 ) then -- Let's calculate wheel rotation! calculateVehicleWheelRotation( vehicle, wheels ) end end end ) Client-side (OOP) -- Table containing vehicles that have custom wheels local createdCustomWheels = { } --[[ /customwheels [ number tilt, number scale ] Adds custom wheels to your currently occupied vehicle and optionally applies a tilt angle and wheel scale. ]] addCommandHandler( 'customwheels', function( _, tilt, scale ) -- Let's get our vehicle local vehicle = localPlayer:getOccupiedVehicle( ) -- Oh, we have a vehicle! if ( vehicle ) then -- Let's give it a wheel now vehicle:addCustomWheel( 1075, tilt, scale ) end end ) -- Let's bind that command to F2 as requested by OP bindKey( 'f2', 'down', 'customwheels' ) --[[ void Vehicle:addCustomWheel ( number model [ , number tilt, number scale ] ) Adds custom wheels to the vehicle. ]] function Vehicle:addCustomWheel( model, tilt, scale ) -- If we've given the object's model number if ( tonumber( model ) ) then -- Let's delete any old ones for _, wheel in pairs( createdCustomWheels[ self ] or { } ) do wheel.object:destroy( ) end -- And let's nil it to be clear createdCustomWheels[ self ] = nil -- Let's set some component names we want to customize local wheels = { 'wheel_lf_dummy', 'wheel_rf_dummy' } -- Let's iterate through those components for i = 1, #wheels do -- Let's hide the existing component self:setComponentVisible( wheels[ i ], false ) -- Initialize our wheel table local wheel = { tilt = tonumber( tilt ) or 0, -- We'll default a non-number tilt angle to 0 name = wheels[ i ], -- Let's store the component name object = Object( model, Vector3( ) ) -- Let's make a new wheel object } -- Let's set the vehicle as the wheel object's parent wheel.object:setParent( self ) -- Let's make sure the wheel is not colliding with the vehicle wheel.object:setCollidableWith( self, false ) -- Let's change the scale wheel.object:setScale( tonumber( scale ) or 0.7 ) -- Let's update that original table wheels[ i ] = wheel end -- And let's store all of those wheels now createdCustomWheels[ self ] = wheels end end --[[ void Vehicle:calculateWheelRotation ( table wheel ) Let's calculate the wheel rotation for given wheel(s). ]] function Vehicle:calculateWheelRotation( wheel ) -- If we have many wheels in the given argument if ( type( wheel ) == 'table' ) and ( #wheel > 0 ) then -- Let's iterate through them all for i = 1, #wheel do -- Let's call the method alone with just the iterator self:calculateWheelRotation( wheel[ i ] ) end -- Let's stop here now return end -- If we have an object if ( wheel.object ) then -- Let's get the rotation vector of the original component local rotation = Vector3( self:getComponentRotation( wheel.name, 'world' ) ) -- Let's set our tilt angle rotation.y = wheel.tilt -- Let's make sure the wheel is at the original component's position wheel.object:setPosition( self:getComponentPosition( wheel.name, 'world' ) ) -- Let's finally set the rotation wheel.object:setRotation( rotation ) end end -- Render-time! addEventHandler( 'onClientPreRender', root, function( ) -- Let's iterate through all the vehicles for vehicle, wheels in pairs( createdCustomWheels ) do -- If we have a vehicle, it's streamed in and it has wheels if ( vehicle ) and ( vehicle:isStreamedIn( ) ) and ( #wheels > 0 ) then -- Let's calculate wheel rotation! vehicle:calculateWheelRotation( wheels ) end end end )
-
English only here. Portuguese / Português: https://forum.multitheftauto.com/forum/97-portuguese-português/
-
I advise you to check out these topics to get you started with Lua: https://wiki.multitheftauto.com/wiki/Scripting_Introduction
-
Nothing. You just need to make sure you've defined veh[ source ]. What I did was I created a new vehicle when they entered the first marker, which defined veh[ source ] as that. Then the vehicle would stay as veh[ source ] on the 4th marker, and then it deletes the vehicle. I don't know where you want to define veh[ source ]. Is it spawned somewhere else in code, or do you want them to spawn a vehicle once they hit the first marker? If you want it to work even without a vehicle, then move everything else out of the if-statement except for the destroyElement.
-
Always when you define variables, you define them like this in Lua: variable = something -- timer = setTimer(...) etc. So, your timer is never defined in a variable, so calling a variable called timer would do nothing... Same problem with newState, where is it defined? How can it know what it is, if you never define what it is?
-
I don't know what your Money-function does, so I had to guess that you didn't complete that part. Anyway, what this does, is it sends the amount from client to the server, stores that number in a table and then once they hit the specified marker, they will get that amount and so on. I also added an onPlayerQuit event handler in case you want to avoid confusion. I also used setElementVisibleTo to show/hide the marker to the player, because setMarkerSize isn't reliable enough (and it's hacky code despite the fact that it might do the job). Client-side -- You had their "relative" parameter set to "true", that's why -- they didn't show... now they're set to "false" sett = guiCreateButton( 507, 278, 64, 37, "Set The Money", false ) money = guiCreateEdit( 448, 225, 166, 45, "", false ) -- When the button is clicked addEventHandler( "onClientGUIClick", sett, function( ) -- Get the contents of the edit box local amount = money and guiGetText( money ) -- Let's convert that amount to a number if we can amount = tonumber( amount ) and tonumber( math.floor( amount ) ) -- If the amount is a (realistic) number, we let them continue if ( amount ) then -- If the amount is greater than 0 if ( amount > 0 ) then -- We send the amount to the server triggerServerEvent( "SendMoney", localPlayer, amount ) else -- Otherwise let's output an error message outputChatBox( "Bad amount!" ) end -- Let's put our fixed number into the edit box too guiSetText( money, amount ) end end, false ) Server-side -- Let's initialize a new table to store pending amounts in local waitlist = { } -- Once we receive a SendMoney event from the client addEventHandler( "SendMoney", root, function( amount ) -- Let's convert the sent argument to a number again amount = tonumber( amount ) and math.floor( tonumber( amount ) ) -- If they are who they say they are, and the amount is a (realistic) number if ( source == client ) and ( amount ) and ( amount > 0 ) then -- Let's add the user and their amount to a table for storage waitlist[ client ] = amount -- Use this to show myMarker1 for the user setElementVisibleTo( myMarker1, client, true ) -- Output some confirmation outputChatBox( amount .. "$ set!", client ) else -- If myMarker1 is showing, hide it if ( isElementVisibleTo( myMarker1, client ) ) then setElementVisibleTo( myMarker1, client, false ) end end end ) -- Once someone hits myMarker1 addEventHandler( "onMarkerHit", myMarker1, function( hitElement, matchingDimension ) -- If our dimension matches if ( matchingDimension ) then -- Let's get the amount from the table local amount = waitlist[ hitElement ] -- If we have an amount stored in the table if ( amount ) then destroyElement( gold ) givePlayerMoney( hitElement, amount ) sendClientMessage( "- " .. getPlayerName( hitElement ) .. " money", root, 255, 23, 23, top, 15 ) outputChatBox( " ", root, 183, 92, 38 ) -- Let's hide the marker from the user setElementVisibleTo( source, hitElement, false ) -- Let's reset that pending amount from the table waitlist[ hitElement ] = nil end end end ) -- If someone quits the game, let's be sure to clear their pending amount addEventHandler( "onPlayerQuit", root, function( ) waitlist[ source ] = nil end ) Tested and working.
-
That happens because there is no veh[ source ] set for the player. I was able to make it work once I defined veh[ source ] with a vehicle, as an example. Here's a cleaned up version: Mk1 = createMarker( -33.35546875, -279.1201171875, 5.625 - 1, "cylinder", 1.1, 255, 255, 0, 255 ) Mk2 = createMarker( -92.0009765625, -307.244140625, 1.4296875 - 1, "cylinder", 7.5, 255, 0, 0 ) Mk3 = createMarker( 1070.2001953125, 1889.2646484375, 10.8203125 - 1, "cylinder", 7.5, 255, 0, 0 ) Mk4 = createMarker( -40.4404296875, -225.564453125, 5.4296875 - 1, "cylinder", 7.5, 255, 0, 0 ) B1 = createBlipAttachedTo( Mk2, 0 ) B2 = createBlipAttachedTo( Mk3, 41 ) B3 = createBlipAttachedTo( Mk4, 19 ) setElementVisibleTo( B1, root, false ) setElementVisibleTo( B2, root, false ) setElementVisibleTo( B3, root, false ) setElementVisibleTo( Mk2, root, false ) setElementVisibleTo( Mk3, root, false ) setElementVisibleTo( Mk4, root, false ) veh = { } function incio( source ) if ( isElementWithinMarker( source, Mk1 ) ) then if ( isElement( veh[ source ] ) ) then destroyElement( veh[ source ] ) end x, y, z = getElementPosition( source ) Trabalho = true setElementVisibleTo( B1, source, true ) setElementVisibleTo( Mk2, source, true ) outputChatBox( "#00ff00Vá carregar o furgão.", source, 0, 0, 0, true ) end end addEventHandler( "onMarkerHit", Mk1, incio ) function carrega( source ) if ( isElementWithinMarker( source, Mk2 ) ) then outputChatBox( "#00ff00Leve a carga do furgão até o local marcado no mapa para descarregar.", source, 0, 0, 0, true ) setElementVisibleTo( B1, source, false ) setElementVisibleTo( Mk2, source, false ) setElementVisibleTo( Mk3, source, true ) setElementVisibleTo( B2, source, true ) end end addEventHandler( "onMarkerHit", Mk2 ,carrega ) function retorna( source ) if ( isElementWithinMarker( source, Mk3 ) ) then outputChatBox( "Agora retorne a empresa com o furgão para receber seu pagamento.", source, 0, 0, 0, true ) setElementVisibleTo( Mk3, source, false ) setElementVisibleTo( B2, source, false ) setElementVisibleTo( Mk4, source, true ) setElementVisibleTo( B3, source, true ) end end addEventHandler( "onMarkerHit", Mk3, retorna ) function fim( source ) -- Never gets triggered, because the player has no vehicle... if ( isElement( veh[ source ] ) ) then destroyElement( veh[ source ] ) givePlayerMoney( source, 1000 ) setElementVisibleTo( B3, source, false ) setElementVisibleTo( B4, source, false ) -- There is no B4 blip in the script? This throws an error. end end addEventHandler( "onMarkerHit", Mk4, fim ) function sair( source ) if ( isElement( veh[ source ] ) ) then setElementVisibleTo( B1, source, false ) destroyElement( veh[ source ] ) outputChatBox( "#00ff00Você saiu do veiculo,desobedeceu as ordems do chefe e foi demitido.", source, 0, 0, 0, true ) end end addEventHandler( "onVehicleExit", root, sair )
-
Even shorter. -- bool Vector2:within ( Vector2 position, Vector2 size ) function Vector2:within( position, size ) local diff = ( self - position ) / size return diff.x >= 0 and diff.x <= 1 and diff.y >= 0 and diff.y <= 1 end local cursor = Vector2( getCursorPosition( ) ) * Vector2( guiGetScreenSize( ) ) local position, size = Vector2( 500, 500 ), Vector2( 50, 50 ) if ( isCursorShowing( ) ) and ( cursor:within( position, size ) ) then -- cursor (enabled with showCursor) is inside area end if ( getCursorPosition( ) ) and ( cursor:within( position, size ) ) then -- cursor is inside area end
-
This is an international forum, please write in English.
-
Exactly. In my opinion, the price could even be 2x as big as it is now. If people can't afford a 20 dollar marketing budget, then I don't know what they can afford.
-
I don't think MTA serials have any significant probability for a collision like that. I'd even go as far as to say that the guy who played played on multiple machines, aka multiboxing.
-
I made my own map conversion tool: http://convert.sites.paju.io/ You may also see the Resource:Race Wiki page which has a batch converter application that you can run on your PC. So if you want to make sure the conversion works, use that application as it probably does it correctly; I only used your map file as a reference when making that conversion tool site.
-
You're welcome!
-
Oh, right, forgot getCursorPosition returns a relative vector instead. This should do it: local screenWidth, screenHeight = guiGetScreenSize( ) function drawCursor( ) if ( isCursorShowing( ) ) then local cursorX, cursorY = getCursorPosition( ) dxDrawImage( cursorX * screenWidth, cursorY * screenHeight, 41, 41, 'images/cursor.png' ) end end addEventHandler( "onClientRender", root, drawCursor ) showCursor( true ) setCursorAlpha( 0 )
-
Use the warpPedIntoVehicle function to do that.
-
You can move the setCursorAlpha outside to where you use showCursor, because you are now setting the alpha all the time, whereas you only need to put it once. That script seems to be fine.
-
You need to put the getCursorPosition function inside the renderer as well (you want it to get the cursor vector all the time, not only in the beginning of the script).
-
Give me the map and I'll see what's wrong.
-
You can use this http://www.convertffs.com/ Choose MTA Race Object and MTA Race Spawnpoint, and then convert to MTA 1.0 Object and MTA 1.0 Vehicle.