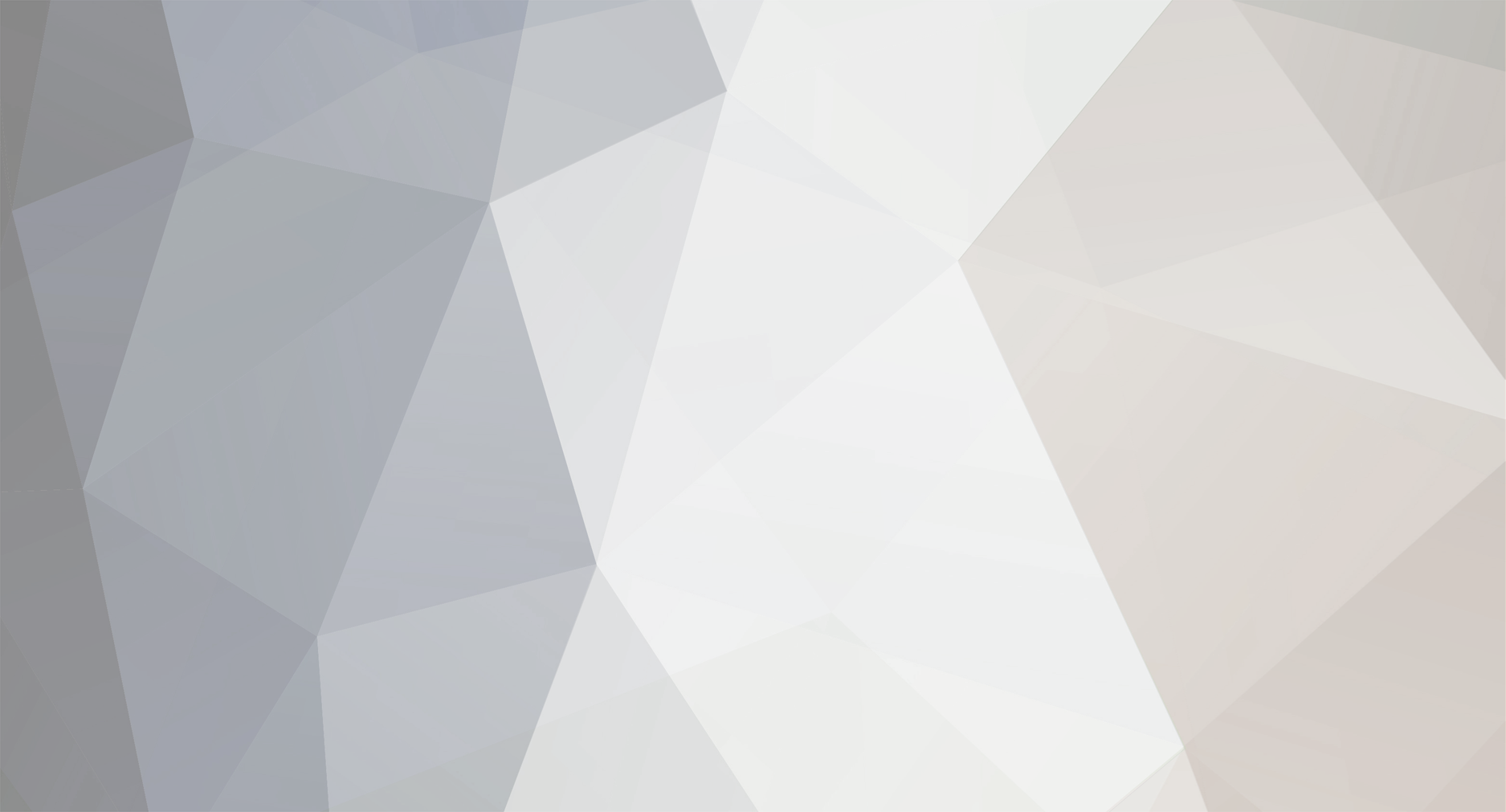
myonlake
Members-
Posts
2,312 -
Joined
-
Days Won
41
Everything posted by myonlake
-
Like Arran explained, here it is, done exactly like he said. addEventHandler("onPlayerLogin", getRootElement(), function(_, account) outputChatBox(getPlayerName(source).."=> You successfully logged in.",source) setElementData(source, "admin.level", getAccountData(account, "admin.level") or 0) end ) addEventHandler("onPlayerLogout", root, function(account) setElementHealth(source, 100) outputChatBox("You logged out", source) setAccountData(account, "admin.level", getElementData(source, "admin.level") or 0) removeElementData(source, "admin.level") end ) And yes, it does work. Not sure what you're doing wrong.
-
You can probably use this resource to get some idea how to do that: https://community.multitheftauto.com/index.php?p=resources&s=details&id=11851
-
It's not working because there is no such function as dxDraw3DText. You need to implement that yourself, or use that function linked to you in the above reply.
-
Sure, you can do that, but that kinda takes away the whole point of having functions in scripting languages in general. What if that element data key changes? You'll need to replace that everywhere. That's why we use functions. And instead of doing all that weird code, you can simply just write this and it'll do the same thing. if ( getElementData( thePlayer, 'gang' ) == 'yourGangName' ) then -- Your gate script end
-
Well, I don't have a fix for you. What I do have is a temporary solution to keep the script rolling. --[[for i, v in ipairs ( clothSlots ) do setAccountData(account,v,getElementData(source,v)) end]] Just comment out that part like so (and any other parts which use clothSlots).
-
You're welcome.
-
Well, the position was checked incorrectly, here is a better version with minimal changes done to the code: function Vector3:compare( comparison, precision ) if ( not precision ) then if ( self:getX( ) ~= comparison:getX( ) ) or ( self:getY( ) ~= comparison:getY( ) ) or ( self:getZ( ) ~= comparison:getZ( ) ) then return false end else if ( math.abs( self:getX( ) - comparison:getX( ) ) > precision ) or ( math.abs( self:getY( ) - comparison:getY( ) ) > precision ) or ( math.abs( self:getZ( ) - comparison:getZ( ) ) > precision ) then return false end end return true end function isElementMoving ( theElement ) if isElement ( theElement ) then local x, y, z = getElementVelocity( theElement ) return x ~= 0 or y ~= 0 or z ~= 0 end return false end function onPlayerTryUsinKit(player) if(player.type == "player") then isKit = player:getData("kit") if (isKit ~= false) then if not (isPedInVehicle(player)) then isMoving = isElementMoving(player) if (isMoving) then player:outputChat("Você precisa estar parado para usar o Kit Medico!", 255, 255, 0, false) else player:outputChat("Fique parado por 5 segundo para aplicar o Kit Medico", 255, 255, 0, false) player:setData("position", player:getPosition(),false) Timer(useKitMedic, 5000, 1, player) end end else player:outputChat("Você não tem Kit Medico para usar!", 255, 255, 0, false) end end end function useKitMedic(player) if(not player:getPosition():compare(player:getData('position'))) then player:outputChat("Falha ao usar o Kit Medico! Voce se moveu!", 255, 0, 0, false) else kitTimer = Timer(function(player) if(isElement(player)) then if(not player:getPosition():compare(player:getData('position'))) then player:outputChat("Falha ao usar o Kit Medico! Voce se moveu!", 255, 0, 0, false) kitTimer:destroy() else health = player:getHealth() player:setHealth(health + 5) player:outputChat('ok') end end end, 1000, 0, player) end end addEventHandler("onResourceStart", resourceRoot, function () local players = getElementsByType("player") for k,v in ipairs(players) do bindKey(v, "K", "down", onPlayerTryUsinKit) end end ) Here is a little bit more cleaned up version: --[[ Let's make a comparison method for Vector3 to make our job faster. bool Vector3:compare( Vector3 comparison [ , float precision ] ) ]] function Vector3:compare( comparison, precision ) -- If we're not using any specific precision, let's optimize -- the process and just check if they are not equal if ( not precision ) then if ( self:getX( ) ~= comparison:getX( ) ) or ( self:getY( ) ~= comparison:getY( ) ) or ( self:getZ( ) ~= comparison:getZ( ) ) then return false end else -- If we have some precision set, let's compare all absolute -- components to the precision if ( math.abs( self:getX( ) - comparison:getX( ) ) > precision ) or ( math.abs( self:getY( ) - comparison:getY( ) ) > precision ) or ( math.abs( self:getZ( ) - comparison:getZ( ) ) > precision ) then return false end end -- If all went fine, let's return the good news return true end --[[ Since MTA doesn't come with this method, let's make one. So we basically compare the velocity vector to the zeroed vector. bool Element:isMoving( void ) ]] function Element:isMoving( ) return not self:getVelocity( theElement ):compare( Vector3( 0, 0, 0 ) ) end --[[ Let's initialize a method for the player that starts the useKit process. void Player:tryKit( void ) ]] function Player:tryKit( ) -- If we're dead, we can't really do anything anyway. if ( self:isDead( ) ) then return end -- And let's make sure we actually need that medkit. if ( self:getHealth( ) < 100 ) then -- How many kits are left to use? local kits = self:getData( 'kit' ) -- If we have any kits available. if ( kits ) and ( tonumber( kits ) > 0 ) then -- And we're not in a car, because we need to pay attention to the road, of course (no Teslas in 90's). if ( not self:isInVehicle( ) ) then -- Let's make sure we're not moving. if ( self:isMoving( ) ) then self:outputChat( "Você precisa estar parado para usar o Kit Medico!", 255, 0, 0 ) else -- Once we're past all the checks, let's save our position (no-sync) and initialize the kit timer for 5000 ms self:outputChat( "Fique parado por 5 segundo para aplicar o Kit Medico", 255, 255, 0 ) self:setData( 'position', self:getPosition( ), false ) self.kitTimer = Timer( function( player ) -- Once that timer is past, we'll start healing ourselves! player:useKit( ) end, 5000, 1, self ) end end else self:outputChat( "Você não tem Kit Medico para usar!", 255, 0, 0 ) end else self:outputChat( "You already have max health.", 255, 0, 0 ) end end --[[ When we want to use a kit, we'll call this method. void Player:useKit( void ) ]] function Player:useKit( ) -- If we're dead, we can't really do anything anyway. if ( self:isDead( ) ) then return end -- Have we moved from our saved position? if ( not self:getPosition( ):compare( self:getData( 'position' ) ) ) then self:outputChat( "Falha ao usar o Kit Medico! Voce se moveu!", 255, 0, 0 ) else -- Let's get our current health. local health = self:getHealth( ) -- And let's make sure we actually need a fix. if ( health < 100 ) then -- Yep, let's hit that arm with some of that good stuff. self:setHealth( health + 5 ) -- Is there any need for more? if ( health + 5 < 100 ) then -- Sure there is, so let's keep on doing that every 1000 ms. self.kitTimer = Timer( function( player ) -- Eat, sleep, heal, repeat... or what was that again. player:useKit( ) end, 1000, 1, self ) end end end end --[[ Funny thing is as much as healing yourself while not on the server sounds good, we're not able to do that on the fly, so we'll just stop timers when you quit. ]] addEventHandler( 'onPlayerQuit', root, function( ) -- If there is a kitTimer initialized, we'll destroy it! if ( isTimer( source.kitTimer ) ) then source.kitTimer:destroy( ) end end ) --[[ When the party begins... ]] addEventHandler( 'onResourceStart', resourceRoot, function( ) -- We start by inviting all of our friends over. for _, player in ipairs( Element.getAllByType( 'player' ) ) do -- And then giving them some of those medkit juice drinks that everybody loves. bindKey( player, 'K', 'down', function( ) -- And so they can now taste them by pressing that good ol' K. Sound good? player:tryKit( ) end ) end end )
-
Well, I suppose you can then call this instead of that getElementData in the reply above. if ( not isGuestAccount( getPlayerAccount( thePlayer ) ) and ( isGangMember( 'yourGangName', getAccountName( getPlayerAccount( thePlayer ) ) ) ) then -- Your gate script end You will need to execute the gate script inside the DayZ resource, as otherwise it'll not be able to find the isGangMember function that is included in the resource.
-
As far as I can see, there is no table for clothSlots, which means the for loop will cause an error. I don't know if it's relevant (probably is, or at least was), but as a temporary fix, you can just comment out that for loop.
-
Where did you download the DayZ gamemode so I can have a look at it.
-
No. You'll need to make your own script to handle multiple server configurations and instances.
-
Please format your code. And refrain from using all-caps in the title.
-
So executing one line of code and two commands is too difficult? Boy, the smell of lazyness.
-
Unable to reproduce with a different type of test. Client-side test results 27.12.2016 18:09 > testEngineStateFalse > tests completed: 212 > failed tests: 6 > passed tests: 206 > failed ids: 449, 537, 538, 570, 590, 569 > passed ids: 602, 545, 496, 517, 401, 410, 518, 600, 527, 436, 589, 580, 419, 439, 533, 549, 526, 491, 474, 445, 467, 604, 426, 507, 547, 585, 405, 587, 409, 466, 550, 492, 566, 546, 540, 551, 421, 516, 529, 592, 553, 577, 488, 511, 497, 548, 563, 512, 476, 593, 447, 425, 519, 520, 460, 417, 469, 487, 513, 581, 510, 509, 522, 481, 461, 462, 448, 521, 468, 463, 586, 472, 473, 493, 595, 484, 430, 453, 452, 446, 454, 485, 552, 431, 438, 437, 574, 420, 525, 408, 416, 596, 433, 597, 427, 599, 490, 432, 528, 601, 407, 428, 544, 523, 470, 598, 499, 588, 609, 403, 498, 514, 524, 423, 532, 414, 578, 443, 486, 515, 406, 531, 573, 456, 455, 459, 543, 422, 583, 482, 478, 605, 554, 530, 418, 572, 582, 413, 440, 536, 575, 534, 567, 535, 576, 412, 402, 542, 603, 475, 441, 464, 501, 465, 564, 568, 557, 424, 471, 504, 495, 457, 539, 483, 508, 571, 500, 444, 556, 429, 411, 541, 559, 415, 561, 480, 560, 562, 506, 565, 451, 434, 558, 494, 555, 502, 477, 503, 579, 400, 404, 489, 505, 479, 442, 458, 606, 607, 610, 611, 584, 608, 435, 450, 591, 594 > failed types: Train Pastebin for client test code: https://pastebin.mtasa.com/304240389 [^] __________ Server-side test results 27.12.2016 18:21 > testEngineStateFalse > tests completed: 212 > failed tests: 6 > passed tests: 206 > failed ids: 449, 537, 538, 570, 590, 569 > passed ids: 602, 545, 496, 517, 401, 410, 518, 600, 527, 436, 589, 580, 419, 439, 533, 549, 526, 491, 474, 445, 467, 604, 426, 507, 547, 585, 405, 587, 409, 466, 550, 492, 566, 546, 540, 551, 421, 516, 529, 592, 553, 577, 488, 511, 497, 548, 563, 512, 476, 593, 447, 425, 519, 520, 460, 417, 469, 487, 513, 581, 510, 509, 522, 481, 461, 462, 448, 521, 468, 463, 586, 472, 473, 493, 595, 484, 430, 453, 452, 446, 454, 485, 552, 431, 438, 437, 574, 420, 525, 408, 416, 596, 433, 597, 427, 599, 490, 432, 528, 601, 407, 428, 544, 523, 470, 598, 499, 588, 609, 403, 498, 514, 524, 423, 532, 414, 578, 443, 486, 515, 406, 531, 573, 456, 455, 459, 543, 422, 583, 482, 478, 605, 554, 530, 418, 572, 582, 413, 440, 536, 575, 534, 567, 535, 576, 412, 402, 542, 603, 475, 441, 464, 501, 465, 564, 568, 557, 424, 471, 504, 495, 457, 539, 483, 508, 571, 500, 444, 556, 429, 411, 541, 559, 415, 561, 480, 560, 562, 506, 565, 451, 434, 558, 494, 555, 502, 477, 503, 579, 400, 404, 489, 505, 479, 442, 458, 606, > failed types: Train Pastebin for server test code: https://pastebin.mtasa.com/591127685 [^]
-
You can use those data points client-side. Here is an example /myvehicles which outputs your vehicle data on client-side. addCommandHandler( 'myvehicles', function( ) local vehicles = getElementData( localPlayer, "VS.Vehicles" ) if ( type( vehicles ) == 'table' ) and ( #vehicles > 0 ) then outputChatBox( "Your vehicles:" ) for index, data in ipairs( vehicles ) do outputChatBox( " Vehicle #" .. index .. " is a " .. getVehicleNameFromModel( data.id ) .. " and has a price of $" .. data.price .. "." ) end else outputChatBox( "You have no vehicles.", 255, 0, 0 ) end end ) Tables in Lua: http://lua-users.org/wiki/TablesTutorial For in Lua: http://lua-users.org/wiki/ForTutorial There is also a tutorial on tables here on MTA forums, I see:
-
You're welcome. In that cleaned up version I removed the JSON encoding, because in the end, you don't really need it, unless you're outputting or transferring the data somewhere - even then, you should be doing the encoding when you need it, and not just because you can on initialization. Otherwise you're always ending up decoding the data when you need to use it, which is a lot more work than just letting it be. I also made that vehicle spawn properly with the rotation of the player. I also cleaned up the unnecessary keeping of those counts when resetting the vehicles table, since the only case that happens is when you have no vehicles. You can of course change it back if you have some other code which does something that messes up the vehicles data...
-
You haven't initialized the index in the table, that's why it cannot set the values. You also had a pretty dangerous for loop over that which can potentially crash your server. No idea why you wanted to iterate through the vehicles. For both of the versions, I fixed the last outputChatBox that had no target player defined in the arguments, it's now set to player like the other ones. Here's your script with minimal changes: createdVehicle = {} function buyVehicle(player,id,price) local money = getPlayerMoney(player) if ( money >= price ) and ( not ( isGuestAccount(getPlayerAccount(player)) ) ) and ( not ( getElementData(player,"VS.Count") == 5 ) ) then local x, y, z = getElementPosition(player) if ( isElement(createdVehicle[player]) ) then destroyElement(createdVehicle[player]) end createdVehicle[player] = createVehicle(id,x,y-3,z) if ( createdVehicle[player] ) then if ( getElementData(player,"VS.Vehicles") == false ) then local aTable = {} local jsone = toJSON(aTable) setElementData(player,"VS.Vehicles",jsone) local i = getElementData(player,"VS.Count") if ( i == false ) then setElementData(player,"VS.Count",0) else setElementData(player,"VS.Count",tonumber(i)+1) end end local theTable = {fromJSON(getElementData(player,"VS.Vehicles"))} if ( type(theTable) == "table" ) and ( not ( theTable == false ) ) or ( not ( theTable == nil ) ) then local i = getElementData(player,"VS.Count")+1 theTable[i]={} theTable[i][1] = id --[[ HERE is the problem ]]-- theTable[i][2] = 1000 --[[ and here ]]-- theTable[i][3] = price/2 --[[ and here ]]-- setElementData(player,"VS.Vehicles",toJSON(theTable)) setElementData(player,"VS.Count",i) setElementData(createdVehicle[player],"Owner",player) setElementData(createdVehicle[player],"VS.Count",i) takePlayerMoney(player,price) outputChatBox("[Vehicle System] You have bought ".. getVehicleNameFromModel(id) .." for ".. price .."$ !",player,0,255,0) else outputChatBox("[Vehicle System] Error purchasing a car!",player,255,0,0) end end elseif ( isGuestAccount(getPlayerAccount(player)) ) then outputChatBox("[Vehicle System] Please login in order to buy a vehicle!",player,255,0,0) elseif ( money < price ) then outputChatBox("[Vehicle System] You don't have enough money to buy this car!",player,255,0,0) elseif ( getElementData(player,"VS.Count") == 5 ) then outputChatBox("[Vehicle System] You have reached the maximum amount of vehicles to purchase!",player,255,0,0) end end A little bit more cleaned up, if you want it: createdVehicle = { } function buyVehicle( player, id, price ) local money = getPlayerMoney( player ) if ( money >= price ) and ( not isGuestAccount( getPlayerAccount( player ) ) ) and ( not ( getElementData( player, "VS.Count" ) == 5 ) ) then if ( isElement( createdVehicle[ player ] ) ) then destroyElement( createdVehicle[ player ] ) end local x, y, z = getElementPosition( player ) local _, _, rz = getElementRotation( player ) createdVehicle[ player ] = createVehicle( id, Matrix( Vector3( x, y, z ), Vector3( 0, 0, rz ) ):transformPosition( Vector3( 3, 0, 1 ) ), 0, 0, rz ) if ( isElement( createdVehicle[ player ] ) ) then local vehicles = getElementData( player, "VS.Vehicles" ) if ( type( vehicles ) ~= 'table' ) then vehicles = { } setElementData( player, "VS.Vehicles", vehicles ) setElementData( player, "VS.Count", 0 ) end local index = getElementData( player, "VS.Count" ) + 1 vehicles[ index ] = { } vehicles[ index ].id = id vehicles[ index ].something = 1000 vehicles[ index ].price = price / 2 setElementData( player, "VS.Vehicles", vehicles ) setElementData( player, "VS.Count", index ) setElementData( createdVehicle[ player ], "Owner", player ) setElementData( createdVehicle[ player ], "VS.Count", index ) takePlayerMoney( player, price ) outputChatBox( "[Vehicle System] You have bought " .. getVehicleNameFromModel( id ) .. " for $" .. price .. "!", player, 0, 255, 0 ) end elseif ( isGuestAccount( getPlayerAccount( player ) ) ) then outputChatBox( "[Vehicle System] Please login in order to buy a vehicle!", player, 255, 0, 0 ) elseif ( money < price ) then outputChatBox( "[Vehicle System] You don't have enough money to buy this car!", player, 255, 0, 0 ) elseif ( getElementData( player, "VS.Count" ) == 5 ) then outputChatBox( "[Vehicle System] You have reached the maximum amount of vehicles to purchase!", player, 255, 0, 0 ) end end
-
All sounds have a group and index within that group. If you want to disable all sounds inside a group, you can do that. You can also disable a single sound inside a group. bool setWorldSoundEnabled( int group, [ int index = -1, ] bool enable ) That's the syntax for the function. What Marty was trying to say, is that you can find the group and index of the weapon shots by enabling the development mode client-side, for example with the script provided to you by Marty, and then using /debugscript 3 and /showsound 1 to find out which groups and indexes are triggered when shooting. You should be able to do this quite easily yourself, so you might as well go and try it out now.
-
I see now, I just didn't expect anyone to do that in the first place.
-
The first CREATE TABLE query is invalid, it has a typo "varchat", while it should be "varchar", and it's also missing an ending bracket. CREATE TABLE IF NOT EXISTS vehicles(id int(11), model int(3), x decimal(10,6), y decimal(10.6), z decimal(10.6), rotx decimal(10.6), roty decimal(10,6), rotz decimal(10.6), engine int(1), locked int(1), lights int(1), hp float, color1 varchar(50), color2 varchar(50), plate text, owner text, interior int(5), dimension int(5), upgrades varchar(150), headLights varchar(30), variant1 int(3), variant2 int(3)) That probably sorts it out.