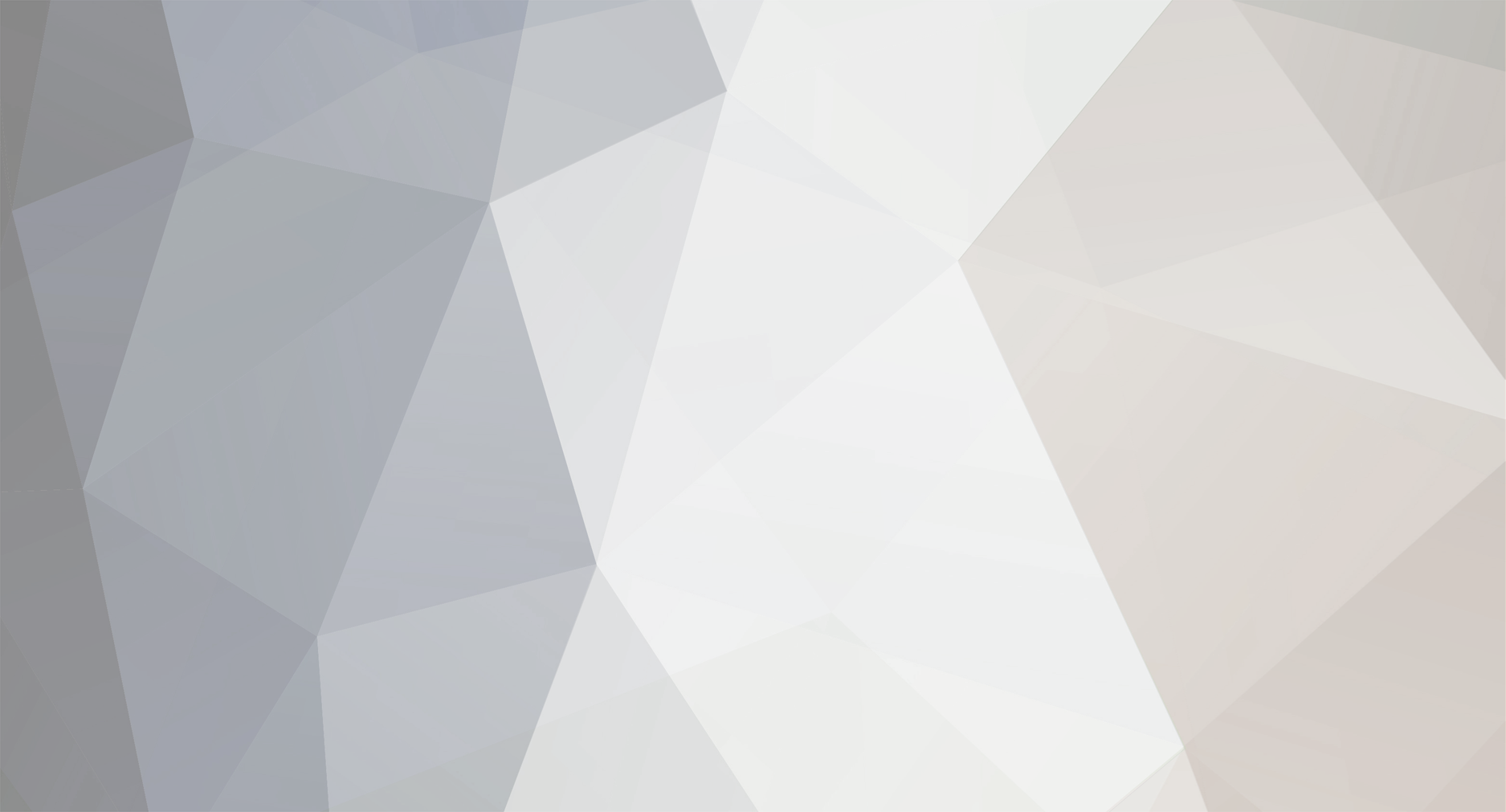
myonlake
Members-
Posts
2,312 -
Joined
-
Days Won
40
Everything posted by myonlake
-
getElementsByType returns a table containing all elements for a specific type. In that script it looks for all pedestrians and draws a dxDrawText for each of them.
-
Right there. local peds = getElementsByType( "ped" ) for _,ped in ipairs( peds ) do -- ... end It is used to repeat the contents of that loop to each and every pedestrian on the server. It is useful for nametags, scoreboards, and such.
-
Also in addition, keep in mind that the multi-dimensional table example is not very good. For-looping through each dimension is not efficient and you should totally go for another approach on that.
-
Well, here is a small example on how a numeric for loop works. for index=0,10 do print( "This number is " .. index .. "." ) end It repeats the contents of that for loop 10 times, as 0,10 means from 0 to 10. That for loop will print the following: This number is 0. This number is 1. This number is 2. This number is 3. This number is 4. This number is 5. This number is 6. This number is 7. This number is 8. This number is 9. This number is 10. Now, there is also a generic for loop, which is based on doing something to each and every division of a table. An example of a generic for loop could be the following: local animals = { cat = " says meow!", dog = " says woof!", car = " says wroom wroom!" } for key,value in pairs( animals ) do print( key .. value ) end It repeats the contents of that for loop as well, but this time it doesn't do it between numbers, but between the amount of table divisions (for cat, dog and car). So this will print the following: car says wroom wroom! dog says woof! cat says meow! Now, if you had a table inside of a table division, you would have to make another for loop for that table, like the following: local animals = { cats = { male = { "Robert", "Daniel" }, female = { "Holly", "Britney" } }, dogs = { male = { "Jack", "Patrick" }, female = { "Emma", "Caitlin" } } } for category,genders in pairs( animals ) do print( "My " .. category .. ":" ) for gender,animal in pairs( genders ) do for _,name in ipairs( animal ) do print( " " .. name .. " is a " .. gender ) end end end And that would print the following: My dogs: Jack is a male Patrick is a male Emma is a female Caitlin is a female My cats: Robert is a male Daniel is a male Holly is a female Britney is a female When you're handling generic for loops with just a number key (0, 1, 2...) and they don't change or get deleted, you should be using index-value pairs (ipairs) instead of key-value pairs (pairs). The big difference being, that index-value relays on a properly formed, numerical list of contents - while key-value relays on just simply everything without any type of forming or so. It is good to know the difference and when to use either. The main rule is that you use pairs for string keys, and ipairs for numerical keys - again, there are exceptions, such as if the key is suddenly removed, which will mess up the order and will return a nil, or a partial table without the rest. Hopefully this helped you out.
-
Exactly. Place your other chat functions into the event after the cancelEvent( ) function.
-
No it won't, element datas are really powerfull. Indeed. The main reason for that is that element data isn't going thru the Lua VM, but the core, so it should be considered as a more native feature. Depends on the usage though, sometimes tables are far better than element data.
-
Actually I don't think it is possible. I remembered wrong; you can't unbind MTA internal commands. However, you can do the following, like Anubhav mentioned above: Server-side addEventHandler( "onPlayerChat", root, function( message, messageType ) if ( messageType == 1 ) then cancelEvent( ) -- Do your own stuff end end )
-
Yes, it is possible to unbind the say key and bind it with another one.
-
I wonder what do you mean by "new mathematical calculations"? I recall all schools in this world teach people trigonometric functions, but okay. And they also teach how to calculate spheres, so yep... But, you're welcome.
-
To even begin using the function, you need to render it like so. local position = { startX = 0, -- x coordinate of the start position startY = 0, -- y coordinate of the start position startZ = 4, -- z coordinate of the start position endX = 10, -- x coordinate of the end position endY = 10, -- y coordinate of the end position endZ = 10 -- z coordinate of the end position } addEventHandler( "onClientHUDRender", root, function( ) dxDrawLine3D( position.startX, position.startY, position.startZ, position.endX, position.endY, position.endZ, tocolor( 245, 20, 20, 180 ), 3.25 ) end ) That marker is made with mathematical calculations to make it a circle. Simply just read more about trigonometric functions and test the formula examples, which you can find by searching on Google. The one you are especially looking for is the unit circle.
-
That's made with dxDrawLine3D. You have to use that in combination with mathematical calculations to make it work. I suppose you could change the texture too, not sure.
-
As explained by the Wiki site: I posted an example for an issue someone was having, and it's using getTickCount. local final_wait_time = 5000 -- Amount of ticks the last message should be displayed for local phrases = { { 0, "The sun is shining right now" }, { 5000, "And so I think it'll be a great day today" } } local visible, tick = false local function toggleRendering( ) tick = getTickCount( ) local fn = ( visible and removeEventHandler or addEventHandler ) fn( "onClientRender", root, renderDisplay ) visible = not visible end local screen_width, screen_height = guiGetScreenSize( ) function renderDisplay( ) if ( not visible ) or ( not phrases ) or ( #phrases == 0 ) then return end for index,data in ipairs( phrases ) do if ( getTickCount( ) - tick >= data[ 1 ] ) and ( not phrases[ index + 1 ] and ( getTickCount( ) - tick < phrases[ index ][ 1 ] + final_wait_time ) or ( phrases[ index + 1 ] and getTickCount( ) - tick < phrases[ index + 1 ][ 1 ] ) ) then local text_width, text_height = dxGetTextWidth( data[ 2 ] ), dxGetFontHeight( ) dxDrawRectangle( ( screen_width - text_width ) / 2 - 15, ( screen_height - text_height ) / 2 - 15, text_width + 30, text_height + 30, tocolor( 0, 0, 0, 200 ) ) dxDrawText( data[ 2 ], ( screen_width - text_width ) / 2, ( screen_height - text_height ) / 2, ( screen_width - text_width ) / 2 + text_width, ( screen_height - text_height ) / 2 + text_height, tocolor( 255, 255, 255, 255 ) ) end end end addEventHandler( "onClientResourceStart", resourceRoot, function( ) toggleRendering( ) end ) It basically displays text phrases, and each one has a small delay calculated with getTickCount. So, to make it a little bit clearer: addEventHandler( "onClientResourceStart", resourceRoot, function( ) local tick = getTickCount( ) addEventHandler( "onClientRender", root, function( ) -- If 5000 ms (5 seconds) has passed since resource was loaded for the client, then... if ( getTickCount( ) - tick >= 5000 ) then -- ... do something end end ) end )
-
Do not create the vehicle client-side if you're not nearby the vehicle. It will cause the vehicle to drop under the ground and it will bug out. Just create the vehicle server-side.
-
Tables are the answer. However, keep in mind that these two are located in two different areas of the application.
-
And what exactly is this Cit marker and what does it do?
-
You'd need to have multiple servers hooked together. That's just not possible right now.
-
I don't see how it makes things easier.
-
You are welcome.
-
So it works or not? And it's fine to use timers, don't get me wrong. It's just about where and how you plan on using them. It's like having two coffee machines, one is very fast, but makes bad coffee, while the other one is slow and makes good coffee; depends on where you plan on using them, so usually at work they might buy a cheap and fast machine to make loads of coffee though bad taste, and at home they might do the opposite. Not the best example really, but you know. Using getTickCount is quite logical after all. At first back a long time ago I thought it's just not possible to understand, but later found out why: because it's too easy to understand, and ever since I've used often in smaller scripts.
-
Usually when handling massive amount of data and delaying it, it'd be better to use timers, as timers are on the main application level within the cpp files. Tick count on the other hand goes through the Lua VM, and so that is inefficient for massive data and repeated usage. However, as we are using small data and not necessarily repeating it endlessly, I'd stick with tick count as it's also easier to modify in a way. I am not totally "banning" timer usage on this, but I'd highly recommend using ticks, as I find it just way more logical for a small script. That's up to you and your usage though...
-
I suppose I got out of logic in the morning. At the moment it's waiting double the amount it starts from, which doesn't really work out. Change the * 2 part to + 5000, that should fix the issue. @Lin: All you have to do is edit the code to reset such stuff. That was just an example script, and I find no reason to use timers in this system.
-
No, the interior count is 255, you mixed those two