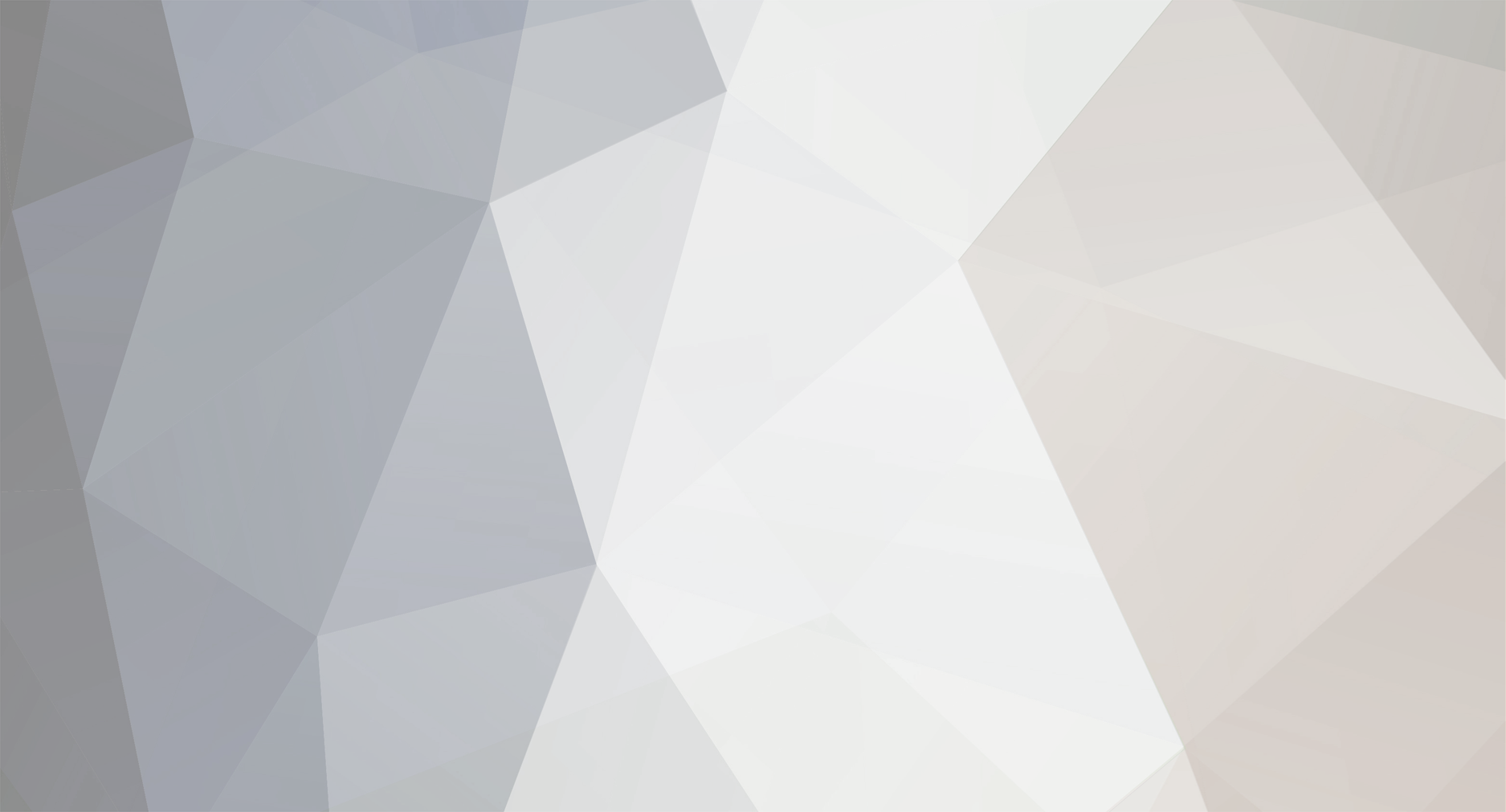
myonlake
Members-
Posts
2,312 -
Joined
-
Days Won
40
Everything posted by myonlake
-
Pretty much like this; https://wiki.multitheftauto.com/wiki/EngineImportTXD and the sub-functions below that, combined with https://wiki.multitheftauto.com/wiki/Cl ... ponent_IDs.
-
Please, do not use a timer for the second text, that's just a waste of good functions that are made for a reason. Use getTickCount instead, and make a table for your texts. local final_wait_time = 5000 -- Amount of ticks the last message should be displayed for local phrases = { { 0, "The sun is shining right now" }, { 5000, "And so I think it'll be a great day today" } } local visible, tick = false local function toggleRendering( ) tick = getTickCount( ) local fn = ( visible and removeEventHandler or addEventHandler ) fn( "onClientRender", root, renderDisplay ) visible = not visible end local screen_width, screen_height = guiGetScreenSize( ) function renderDisplay( ) if ( not visible ) or ( not phrases ) or ( #phrases == 0 ) then return end for index,data in ipairs( phrases ) do if ( getTickCount( ) - tick >= data[ 1 ] ) and ( not phrases[ index + 1 ] and ( getTickCount( ) - tick < phrases[ index ][ 1 ] + final_wait_time ) or ( phrases[ index + 1 ] and getTickCount( ) - tick < phrases[ index + 1 ][ 1 ] ) ) then local text_width, text_height = dxGetTextWidth( data[ 2 ] ), dxGetFontHeight( ) dxDrawRectangle( ( screen_width - text_width ) / 2 - 15, ( screen_height - text_height ) / 2 - 15, text_width + 30, text_height + 30, tocolor( 0, 0, 0, 200 ) ) dxDrawText( data[ 2 ], ( screen_width - text_width ) / 2, ( screen_height - text_height ) / 2, ( screen_width - text_width ) / 2 + text_width, ( screen_height - text_height ) / 2 + text_height, tocolor( 255, 255, 255, 255 ) ) end end end addEventHandler( "onClientResourceStart", resourceRoot, function( ) toggleRendering( ) end )
-
That's awkward. Are you sure you put the client-side code client-side? It shouldn't give such error.
-
Ugh, no. local account = ( getPlayerAccount( p ) and not isGuestAccount( getPlayerAccount( p ) ) ) and getPlayerAccount( p ) or false local account_name = account and getAccountName( account ) or "Unknown"
-
Do you mind checking /debugscript 3 for possible errors. Let me know what it says.
-
Replace the hedit/server/core.lua with this one: http://pastebin.mtasa.com/773420943. You can change the allowed ACL groups on line 65-67. Add a new or statement after the ones there if you want to add another one. If you want to change the group names in the script, then just switch Admin, Moderator or Super Moderator to whatever you use.
-
Yes, but I am not talking about custom sounds, which you are using. I am talking about the same horn sound without any need to download sound files.
-
I would've went for another approach to enable the default GTA horn. Check for how long the client pressed the key, if it was above a certain tick count, then just let them do the horn. I am not sure if this works though, as you have to toggle the control state and I guess MTA doesn't toggle controls in real-time if key is already being pressed before the key is available again... but it would be worth a shot.
-
There were quite many mistakes in the code. You had some unnecessary repeated if statements and some variables that weren't necessarily have to be initiated (try to keep the amount of variables low, for memory reasons really). Also, don't use the local player as an event source, as it won't work like that. I haven't tested the code as I am not able to do that right now, but let me know of any errors in debug unless you figure them out yourself, in fact, I hope you'll at least try to do that. Server-side local pending_vehicles = { } local skinmechanic = { [ 50 ] = true } addEvent( getResourceName( resource ) .. ":accepted", true ) addEventHandler( getResourceName( resource ) .. ":accepted", root, function( ) local vehicle = getPedOccupiedVehicle( client ) if ( not vehicle ) or ( getPedOccupiedVehicleSeat( client ) ~= 1 ) or ( not skinmechanic[ getElementModel( client ) ] ) then return end local driver = getVehicleController( vehicle ) if ( driver ) and ( getElementType( driver ) == "player" ) then if ( getElementHealth( vehicle ) < 800 ) then fadeCamera( source, false, 1.0 ) setTimer( function( vehicle, source ) if ( isElement( vehicle ) ) and ( getElementHealth( vehicle ) < 800 ) then givePlayerMoney( source, 3000 ) fixVehicle( vehicle ) end if ( pending_vehicles[ source ] ) then pending_vehicles[ source ] = nil end fadeCamera( source, true, 1.0 ) end, 2500, 1, vehicle, source ) else outputChatBox( "Error: The vehicle is already in good condition.", source, 255, 0, 0, false ) end end end ) local vehicle_types = { [ "Automobile" ] = true, [ "Helicopter" ] = true, [ "Monster Truck" ] = true } addEventHandler( "onPlayerVehicleEnter", root, function( vehicle, seat, jacked ) if ( jacked ) or ( not skinmechanic[ getElementModel( source ) ] ) or ( not vehicle_types[ getVehicleType( vehicle ) ] ) or ( seat ~= 1 ) then return end local driver = getVehicleController( vehicle ) if ( driver ) and ( getElementType( driver ) == "player" ) then playSoundFrontEnd( source, 40 ) pending_vehicles[ source ] = driver triggerClientEvent( driver, getResourceName( resource ) .. ":display", source ) end end ) addEventHandler( "onPlayerVehicleStartExit", root, function( vehicle ) if ( not skinmechanic[ getElementModel( source ) ] ) or ( not vehicle_types[ getVehicleType( vehicle ) ] ) then return end if ( pending_vehicles[ source ] ) and ( isElement( pending_vehicles[ source ] ) ) then triggerClientEvent( pending_vehicles[ source ], getResourceName( resource ) .. ":display", source, false ) pending_vehicles[ source ] = nil end end ) Client-side local theMechanic local GUIEditor = { button = { }, window = { }, label = { } } function toggleMechanicDisplay( forced_state ) if ( ( not forced_state ) and ( isElement( GUIEditor.window[ 1 ] ) ) ) or ( isElement( GUIEditor.window[ 1 ] ) ) then destroyElement( GUIEditor.window[ 1 ] ) showCursor( false ) return end GUIEditor.window[ 1 ] = guiCreateWindow( 526, 287, 334, 142, "Mechanic", false ) guiWindowSetSizable( GUIEditor.window[ 1 ], false ) GUIEditor.label[ 1 ] = guiCreateLabel( 60, 42, 209, 17, "Do you accept to get your car repaired?", false, GUIEditor.window[ 1 ] ) GUIEditor.button[ 1 ] = guiCreateButton( 9, 92, 150, 40, "Accept", false, GUIEditor.window[ 1 ] ) GUIEditor.button[ 2 ] = guiCreateButton( 169, 91, 155, 41, "Close", false, GUIEditor.window[ 1 ] ) addEventHandler( "onClientGUIClick", GUIEditor.button[ 1 ], function( ) if ( isElement( theMechanic ) ) then triggerServerEvent( getResourceName( resource ) .. ":accepted", theMechanic ) end toggleMechanicDisplay( ) end ) addEventHandler( "onClientGUIClick", GUIEditor.button[ 2 ], function( ) toggleMechanicDisplay( ) end ) showCursor( true ) end addEvent( getResourceName( resource ) .. ":display", true ) addEventHandler( getResourceName( resource ) .. ":display", root, function( forced_state ) if ( isElement( source ) ) and ( getElementType( source ) == "player" ) then theMechanic = source toggleMechanicDisplay( forced_state or true ) else outputDebugString( "An alien tried to abduct you...", 2 ) end end )
-
And why are you guys mixing thePlayer, player and localPlayer together? Just use one player element for the same one...
-
You should do yourself a favor and go read a little bit more about Lua scripting: viewtopic.php?f=148&t=40809.
-
Use the mathematical algorithm inside the print function, for example like the following: -- The current amount of experience the player has local current_experience = 0 -- Ascending order with numerical keys containing the experience points for each level local experience_levels = { 100, 250, 500, 1000, 1500, 2000, 2750, 3250, 4000, 5000, 6250, 7500, 8750, 10000 } -- A function to get the level from experience points local function getLevelFromExperience( experience ) if ( type( experience ) ~= "number" ) then return false, 1 end -- Loop through all experience levels for level,_experience in ipairs( experience_levels ) do -- If there is another level after the current level if ( experience_levels[ level + 1 ] ) then -- If the current experience is less than the next level's experience if ( experience < experience_levels[ level + 1 ] ) then -- Return this level return level end else -- If no other level was found, then return the current level return level end end return false, 2 end -- A function to calculate and return the percentage to next level local function getPercentageToNextLevel( experience ) if ( type( experience ) ~= "number" ) then return false, 1 end -- Check if the current experience doesn't exceed the maximum experience points if ( experience ~= experience_levels[ #experience_levels ] ) then -- Get the current level local level = getLevelFromExperience( experience ) or 1 -- Get the amount of experience required till next level local experience_to_level = experience - experience_levels[ level ] -- Reset the next level experience properly local fixed_experience = experience_levels[ level + 1 ] - experience_levels[ level ] -- Calculate and return the percentage between the levels return math.ceil( 100 - ( experience_to_level / fixed_experience * 100 ) ) end return 0 end -- Get the current width and height of the screen local screen_width, screen_height = guiGetScreenSize( ) -- Initialize a renderer to draw the level data on screen addEventHandler( "onClientRender", resourceRoot, function( ) -- Get the current level local level = getLevelFromExperience( current_experience ) or 1 -- If the experience is less than the maximum amount, then increment the current experience for demonstration if ( current_experience ~= experience_levels[ #experience_levels ] ) then current_experience = current_experience + 2 * level end -- Initialize a string containing the data local text = "Current level: " .. level .. " | Current experience: " .. current_experience .. " (Progress till next level: " .. getPercentageToNextLevel( current_experience ) .. "%)" -- Draw a shadow under the actual text dxDrawText( text, screen_width - dxGetTextWidth( text, 2.0 ) - 14, screen_height - dxGetFontHeight( 2.0 ) - 14, screen_width, screen_height, tocolor( 0, 0, 0, 125 ), 2.0, "default", "left", "top" ) -- Draw the actual text containing the level data dxDrawText( text, screen_width - dxGetTextWidth( text, 2.0 ) - 15, screen_height - dxGetFontHeight( 2.0 ) - 15, screen_width, screen_height, tocolor( 255, 255, 255, 225 ), 2.0, "default", "left", "top" ) end ) Note that the code is client-side as it has a renderer, but you can use the rest of the code server-side as there are no limitations there. I have not tested the text rendering however as I cannot try it right now, but the math and everything works as I tried it on the Lua engine. I also extended the mathematical algorithm so, that there is no issues with the levels no longer.
-
It's just basic math, really. -- The current amount of experience local current_exp = 3000 -- The next level's experience local next_exp = 4000 -- Calculate the percentage till next level local percentage = math.ceil( 100 - ( current_exp / next_exp * 100 ) ) print( percentage ) -- Prints 25 Note, that you should extend the mathematical algorithm further, as at the moment you are not able to calculate more than one level with this code (it will start from 50 percent from level 2 on).
-
There are many ways to do this, and one way is to use pregReplace in combination with guiGetText and guiSetText. You can trigger the function with the onClientGUIChanged event.
-
Did you use the code Solidsnake or Citizen posted above? It won't work alone, if you're using the admin panel, so that's why you need to use those codes in combination with my code.
-
Are you sure you need to update the direction all the time? You can just make it render everything client-side and update some element data with the direction if necessary.
-
Make sure you use the element data script also instead of event triggers.
-
In this case I don't think that matters, as I doubt no one is going to mute a player before they've loaded everything, if they do, then they should consider revamping their initialization techniques. Yeah, and I think I am not responsible for making that happen, as it's just an example of how the system could work. The rest is up to him, as he didn't request anything else than to display the muted state. And he's also able to export that function from another resource, so I doubt the resource is going to stay stopped for a long time, which would potentially cause these issues. This is why I brought up two different approaches on how to make it work. These are the only ways to make it work (unless you want to make it go through a web server or something stupid), so.
-
Dream on, the performance will drop after a amount of players(take mini-mission as example, true collapse), I am not going to discus this with you what is better. After you send it, you can even so use element data without synchronization. So that isn't truly a super benefit at all. To be honest, I have never seen a big performance drop with element data, especially because when you define something client-side, it'll always be triggered for the client instead of the server, which in this case is very very efficient, as it doesn't have to check the element data through the server each time. I know we've gone through this discussion many times before, but I just don't care to believe it's a bad choice to use element data until a developer proves me totally wrong in that. This is quite lightweight data as well, so it won't synchronize too much information and that's exactly why element data is useful. Unsure how he wanted the mute to be displayed on screen, so I made it in two different ways on both, element data and event triggers. Without element data None of these use element data as you dislike it so much. Server-side local _setPlayerMuted = setPlayerMuted function setPlayerMuted( player, state ) if ( isElement( player ) ) and ( getElementType( player ) == "player" ) and ( type( state ) == "boolean" ) then if ( _setPlayerMuted( player, state ) ) then triggerClientEvent( player, getResourceName( resource ) .. ":mute:sync", player, state ) return true end end return false end Approach #1 Display both muted and unmuted state on the screen as "Mute: Yes/No". The event is never removed. Client-side local is_muted local screen_width, screen_height = guiGetScreenSize( ) addEventHandler( "onClientRender", root, function( ) local mute_text = "Mute: " .. ( is_muted and "Yes" or "No" ) local mute_width, mute_height = dxGetTextWidth( mute_text, 2.0, "default" ), dxGetFontHeight( 2.0, "default" ) dxDrawText( mute_text, screen_width - mute_width - 24, ( screen_height - mute_height ) / 2 + 1, screen_width, screen_height, tocolor( 0, 0, 0, 150 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) dxDrawText( mute_text, screen_width - mute_width - 25, ( screen_height - mute_height ) / 2, screen_width, screen_height, tocolor( 255, is_muted and 0 or 255, is_muted and 0 or 255, 230 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) end ) addEvent( getResourceName( resource ) .. ":mute:sync", true ) addEventHandler( getResourceName( resource ) .. ":mute:sync", root, function( state ) is_muted = state end ) Approach #2 Display only muted state as "You are muted!" and remove the renderer when no longer muted. Client-side local is_muted local screen_width, screen_height = guiGetScreenSize( ) local function drawMuteDisplay( ) if ( not is_muted ) then return end local mute_text = "You are muted!" local mute_width, mute_height = dxGetTextWidth( mute_text, 2.0, "default" ), dxGetFontHeight( 2.0, "default" ) dxDrawText( mute_text, screen_width - mute_width - 24, ( screen_height - mute_height ) / 2 + 1, screen_width, screen_height, tocolor( 0, 0, 0, 150 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) dxDrawText( mute_text, screen_width - mute_width - 25, ( screen_height - mute_height ) / 2, screen_width, screen_height, tocolor( 255, 0, 0, 230 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) end addEvent( getResourceName( resource ) .. ":mute:sync", true ) addEventHandler( getResourceName( resource ) .. ":mute:sync", root, function( state ) local fn = ( is_muted ~= state and ( is_muted and removeEventHandler or addEventHandler ) or nil ) is_muted = state if ( fn ) then fn( "onClientRender", root, drawMuteDisplay ) end end ) With element data And these use element data instead of event triggers. Server-side local _setPlayerMuted = setPlayerMuted function setPlayerMuted( player, state ) if ( isElement( player ) ) and ( getElementType( player ) == "player" ) and ( type( state ) == "boolean" ) then if ( _setPlayerMuted( player, state ) ) then if ( state ) then setElementData( player, getResourceName( resource ) .. ":muted", true, true ) else if ( getElementData( player, getResourceName( resource ) .. ":muted" ) ) then removeElementData( player, getResourceName( resource ) .. ":muted" ) end end return true end end return false end Approach #1 Display both muted and unmuted state on the screen as "Mute: Yes/No". The event is never removed. Client-side local screen_width, screen_height = guiGetScreenSize( ) addEventHandler( "onClientRender", root, function( ) local is_muted = getElementData( localPlayer, getResourceName( resource ) .. ":muted" ) local mute_text = "Mute: " .. ( is_muted and "Yes" or "No" ) local mute_width, mute_height = dxGetTextWidth( mute_text, 2.0, "default" ), dxGetFontHeight( 2.0, "default" ) dxDrawText( mute_text, screen_width - mute_width - 24, ( screen_height - mute_height ) / 2 + 1, screen_width, screen_height, tocolor( 0, 0, 0, 150 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) dxDrawText( mute_text, screen_width - mute_width - 25, ( screen_height - mute_height ) / 2, screen_width, screen_height, tocolor( 255, is_muted and 0 or 255, is_muted and 0 or 255, 230 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) end ) Approach #2 Display only muted state as "You are muted!" and cancel rendering if not muted. Client-side local screen_width, screen_height = guiGetScreenSize( ) addEventHandler( "onClientRender", root, function( ) local is_muted = getElementData( localPlayer, getResourceName( resource ) .. ":muted" ) if ( not is_muted ) then return end local mute_text = "You are muted!" local mute_width, mute_height = dxGetTextWidth( mute_text, 2.0, "default" ), dxGetFontHeight( 2.0, "default" ) dxDrawText( mute_text, screen_width - mute_width - 24, ( screen_height - mute_height ) / 2 + 1, screen_width, screen_height, tocolor( 0, 0, 0, 150 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) dxDrawText( mute_text, screen_width - mute_width - 25, ( screen_height - mute_height ) / 2, screen_width, screen_height, tocolor( 255, 0, 0, 230 ), 2.0, "default", "left", "top", true, false, post_gui, false, false ) end ) Additionally I went on and tested all parts of the code and it seems to be working flawlessly. Hopefully this helped you.
-
Use tonumber( ) like I did above.
-
The following functions should do the trick. -- Server-side setPlayerMuted -- To set the player muted in the first place setElementData -- When player is set muted, then set the element data, which is accessible client-side -- Client-side getElementData -- Get the muted state of the player guiGetScreenSize -- To get the client's screen resolution and position the text accordingly dxDrawText -- To draw the muted state received above And use onClientRender to render the text on the screen.
-
Use tonumber( ) to make it a number value. local marker = createMarker( 2483.900390625, -1666.7001953125, 13.3, "cylinder", 1, 255, 2, 0 ) local drugCount = 0 addCommandHandler( "drugs", function( cmd, amount ) local amount = tonumber( amount ) if ( amount ) and ( amount > 0 ) then local currentDrugs = tonumber( getElementData( localPlayer, "drugs" ) ) or 0 drugCount = currentDrugs + amount setElementData( localPlayer, "drugs", drugCount ) else outputChatBox( "SYNTAX: /" .. cmd .. " [amount]", 220, 185, 20, false ) end end ) addEventHandler( "onClientRender", root, function( ) dxDrawText( tostring( drugCount ), 507, 466, 786, 569, tocolor( 255, 255, 255, 255 ), 1.00, "default", "left", "top", false, false, true, false, false ) end )
-
As long as you have cache set to false, you'll do just fine. There is an exception though, which requires more than enough knowledge in memory addressing and access locations, which isn't really 5-minute-book type of thing. Just encrypt the code and make some small piece of code in that file that checks whether the script is ran on the real server, check for the server information for example, something that recognizes misuse. It doesn't even have to be server name, but if a variable isn't initialized then just rape the whole client till they have to Alt-F4.
-
You cannot have videos on Multi Theft Auto at this time. The only thing you can do is render all frames individually by taking a video and splitting it into thousands of images. That's the only way to make it work, as you cannot have a video codec running on your server, as it has to be running on the client, and server cannot really do anything to the client remotely so it's just not going to happen. I'd like to refer you over to client-side drawing functions.