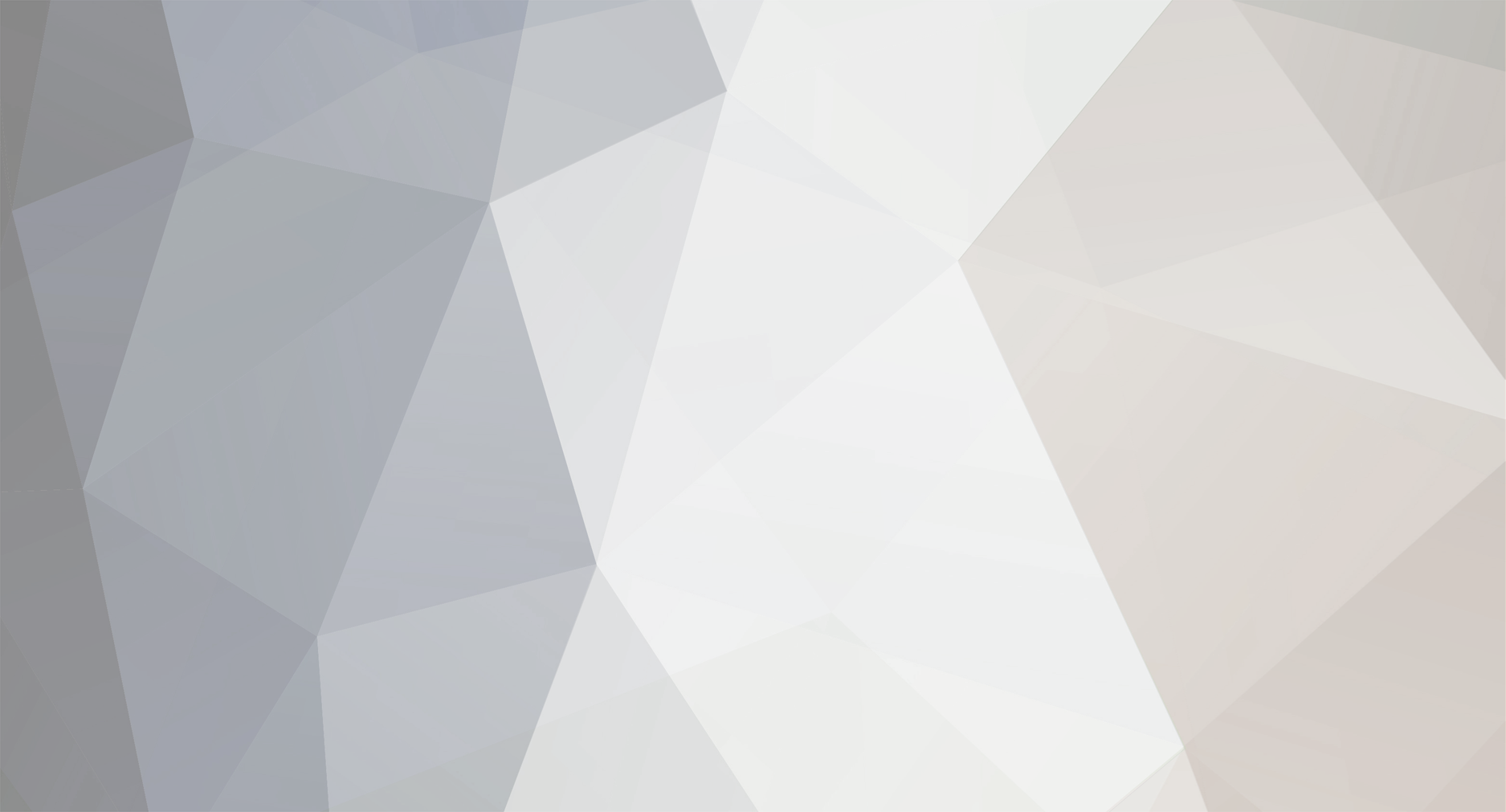
Galactix
Members-
Posts
121 -
Joined
-
Last visited
-
Days Won
2
Everything posted by Galactix
-
addCommandHandler('setskin',function(source,_,player,skinid) local find = findPlayer( player ) if find then local playerAccount = getPlayerAccount(source) local accName = getAccountName ( playerAccount ) if isObjectInACLGroup ("user."..accName, aclGetGroup ( "Admin" ) ) then setElementModel ( find, skinid ) outputChatBox("Your skin was changed to "..skinid.." by the admin "..accName.."!", find) else outputChatBox("You are not allowed to use this command!", source, 255,0,0) end else outputChatBox("Player not found!", source, 255,0,0) end end) And you get the error because the player you want to change the ACL is not online. Don't forget to add a little "Thanks" for the help
-
function findPlayer( namepart ) local player = getPlayerFromName( namepart ) if player then return player end for _,player in pairs( getElementsByType 'player' ) do if string.find( string.gsub( getPlayerName( player ):lower( ),"#%x%x%x%x%x%x", "" ), namepart:lower( ), 1, true ) then return player end end return false end addCommandHandler('setacl',function(source,_,player,aclgroup) local find = findPlayer( player ) if find then local playerAccount = getPlayerAccount(find) local accName = getAccountName ( playerAccount ) if isObjectInACLGroup ("user."..accName, aclGetGroup ( "Admin" ) ) then aclGroupAddObject (aclGetGroup(""..aclgroup..""), "user."..accName) outputChatBox("Your ACL group has been changed to "..aclgroup.."!", find) else outputChatBox("You are not allowed to use this command!", source, 255,0,0) end else outputChatBox("Player not found!", source, 255,0,0) end end)
-
You also need to keep the findPlayer function in the script for it to work.
-
The function is directly in the commandHandler. I just tested the command on my server and it works perfectly, but the resource has to have admin rights.
-
addCommandHandler('setacl',function(source,_,player,aclgroup) local find = findPlayer( player ) if find then local playerAccount = getPlayerAccount(find) local accName = getAccountName ( playerAccount ) if isObjectInACLGroup ("user."..accName, aclGetGroup ( "Owner" ) ) then aclGroupAddObject (aclGetGroup(""..aclgroup..""), "user."..accName) outputChatBox("Your ACL group has been changed to "..aclgroup.."!", source) else outputChatBox("You are not allowed to use this command!", source, 255,0,0) end end end) Also, make sure that the resource you are using has admin rights.
-
addCommandHandler('setacl',function(source,_,player,aclgroup) local find = findPlayer( player ) if find then local playerAccount = getPlayerAccount(find) local accName = getAccountName ( playerAccount ) if isObjectInACLGroup ("user."..accName, aclGetGroup ( "Admin" ) ) then aclGroupAddObject (aclGetGroup(""..aclgroup..""), "user."..accName) outputChatBox("Your ACL group has been changed to "..aclgroup.."!", player) else outputChatBox("You are not allowed to use this command!", player, 255,0,0) end end end)
-
addCommandHandler('setacl',function(source,_,player,aclgroup) local find = findPlayer( player ) if find then local playerAccount = getPlayerAccount(player) aclGroupAddObject (aclGetGroup(""..aclgroup..""), "user."..playerAccount) outputChatBox("The account "..playerAccount.." has been successfully added to the "..aclgroup.." group!", player) end end)
-
Hello, my script is giving out the error "Expected element, got nil" but I tried everything and cannot manage to fix it function findPlayer( namepart ) local player = getPlayerFromName( namepart ) if player then return player end for _,player in pairs( getElementsByType 'player' ) do if string.find( string.gsub( getPlayerName( player ):lower( ),"#%x%x%x%x%x%x", "" ), namepart:lower( ), 1, true ) then return player end end return false end addCommandHandler( 'heal', function( source,_,player ) local find = findPlayer( player ) if find then setElementData(find, "healStatus", true) outputChatBox("The doctor is willing to heal you. Do you accept?", find) else outputChatBox("Player not found!", source, 255, 0, 0) end end) function acceptHeal() local requestStatus = getElementData(player, "healStatus") if requestStatus == true then setElementData(player, "healStatus" , false) -- delete it! setElementHealth(player, 100) outputChatBox("You have been healed by the doctor.", player) else outputChatBox("You have no pending request.", player) end end addCommandHandler("aheal", acceptHeal)
-
Hello, I made a script that makes player able to make a request to heal others but I couldn't get my script to work properly. function findPlayer( namepart ) local player = getPlayerFromName( namepart ) if player then return player end for _,player in pairs( getElementsByType 'player' ) do if string.find( string.gsub( getPlayerName( player ):lower( ),"#%x%x%x%x%x%x", "" ), namepart:lower( ), 1, true ) then return player end end return false end addCommandHandler( 'heal', function( source,_,player ) local find = findPlayer( player ) if find then setElementData(find,"healrequest", "pending") outputChatBox("The doctor is willing to heal you. Do you accept?", find) else outputChatBox("Player not found!", source, 255, 0, 0) end end) function acceptHeal() local requestStatus = getElementData(source, "healrequest") if (requestStatus == "pending") then setElementData(source,"healrequest", "unpending") setElementHealth(source, 100) outputChatBox("You have been healed by the doctor.", player) else outputChatBox("You have no pending request.", player) end end addCommandHandler("aheal", acceptHeal) the part with if requestStatus doesn't work, it just outputs all the time that the player has no pending request. How could I fix that and also make players unable to heal themselves?
-
Sorry, I will make sure to do so in the future!
-
function getPlayerFromPartialName(name) local name = name and name:gsub("#%x%x%x%x%x%x", ""):lower() or nil if name then for _, player in ipairs(getElementsByType("player")) do local name_ = getPlayerName(player):gsub("#%x%x%x%x%x%x", ""):lower() if name_:find(name, 1, true) then return player end end end end addCommandHandler( 'heal', function( source,_,player ) local find = getPlayerFromPartialName( player ) if find then setElementData(player,"healrequest", pending) outputChatBox("The doctor is willing to heal you. Do you accept?", player) else outputChatBox("Player not found!", source, 255, 0, 0) end end) function acceptHeal() local requestStatus = getElementData(source, "healrequest") if (requestStatus = "pending") then setElementData(player,"healrequest", unpending) setElementHealth(player, 100) outputChatBox("You have been healed by the doctor.", source) else outputChatBox("You have no pending request.") end end addCommandHandler("aheal", acceptHeal) @MRThinker
-
function findPlayer( namepart ) local player = getPlayerFromName( namepart ) if player then return player end for _,player in pairs( getElementsByType 'player' ) do if string.find( string.gsub( getPlayerName( player ):lower( ),"#%x%x%x%x%x%x", "" ), namepart:lower( ), 1, true ) then return player end end return false end addCommandHandler('setacl',function(source,_,player,aclgroup) local find = findPlayer( player ) if find then local playerAccount = getPlayerAccount(player) aclGroupAddObject (aclGetGroup(""..aclgroup..""), "user."..playerAccount) end end)
-
Please avoid making multiple threads for the same problem to get more visibility, it’s disrespectful for the other users that need help as much as you do. I will give you the script soon.
-
In the meta.xml of the scoreboard resource change ? name="*forceHideTeams" value="false" to ? name="*forceHideTeams" value="true"
-
Nope. It just says that the resource isn't started if I don't start the extrahealth script but when I turn it on it just doesn't throw any error related to it.
-
Use this serverside function adminColor() if isPlayerInACL(source, "Admin") then setPlayerNametagColor (source, 255, 0, 0) end addEventHandler ("onPlayerLogin", getRootElement(), adminColor)
-
function playerLogin (thePreviousAccount, theCurrentAccount, autoLogin) if not (isGuestAccount (getPlayerAccount (source))) then local accountSkinData = getAccountData (theCurrentAccount, "skinid") spawnPlayer (source, 0, 0, 1, 0, playerSkin, 0, 0) end end addEventHandler ("onPlayerLogin", getRootElement(), playerLogin) function onQuit (quitType, reason, responsibleElement) if not (isGuestAccount (getPlayerAccount (source))) then account = getPlayerAccount (source) if (account) then setAccountData (account, "skinid", tostring (getPedSkin (source))) end end end addEventHandler ("onPlayerQuit", getRootElement(), onQuit)
-
Try to use: setWaterLevel(-5000)
-
Use the /stop [resourcename] command and also you have to remove the resources that you don't want at server start in the mtaserver.conf
-
Just change this: getElementPosition(peds[i]) to this:
-
Well, using your script doesn't color nametags and doesn't cancel any hexcolor coded nametag without giving any error. I have however found this on the wiki for the outputChatBox function which works for the nametag color on players without hexacode colors: function colouredChat ( message, theType ) if theType == 0 then --if its normal chat (not /me or teamchat) then cancelEvent() --prevent MTA from outputting chat message = string.gsub(message, "#%x%x%x%x%x%x", "") --remove any hex tags in a player's chat to prevent custom colours by using lua's string.gsub local r,g,b = getPlayerNametagColor ( source ) --get the player's nametag colour local chatterName = getPlayerName ( source ) --get his name --output a message with the name as his nametag colour, and the rest in white. outputChatBox ( chatterName..":#FFFFFF "..message, getRootElement(), r, g, b, true ) end end addEventHandler("onPlayerChat", getRootElement(), colouredChat) except that the player using nicknames containing hexcolor codes aren't affected by it, why?