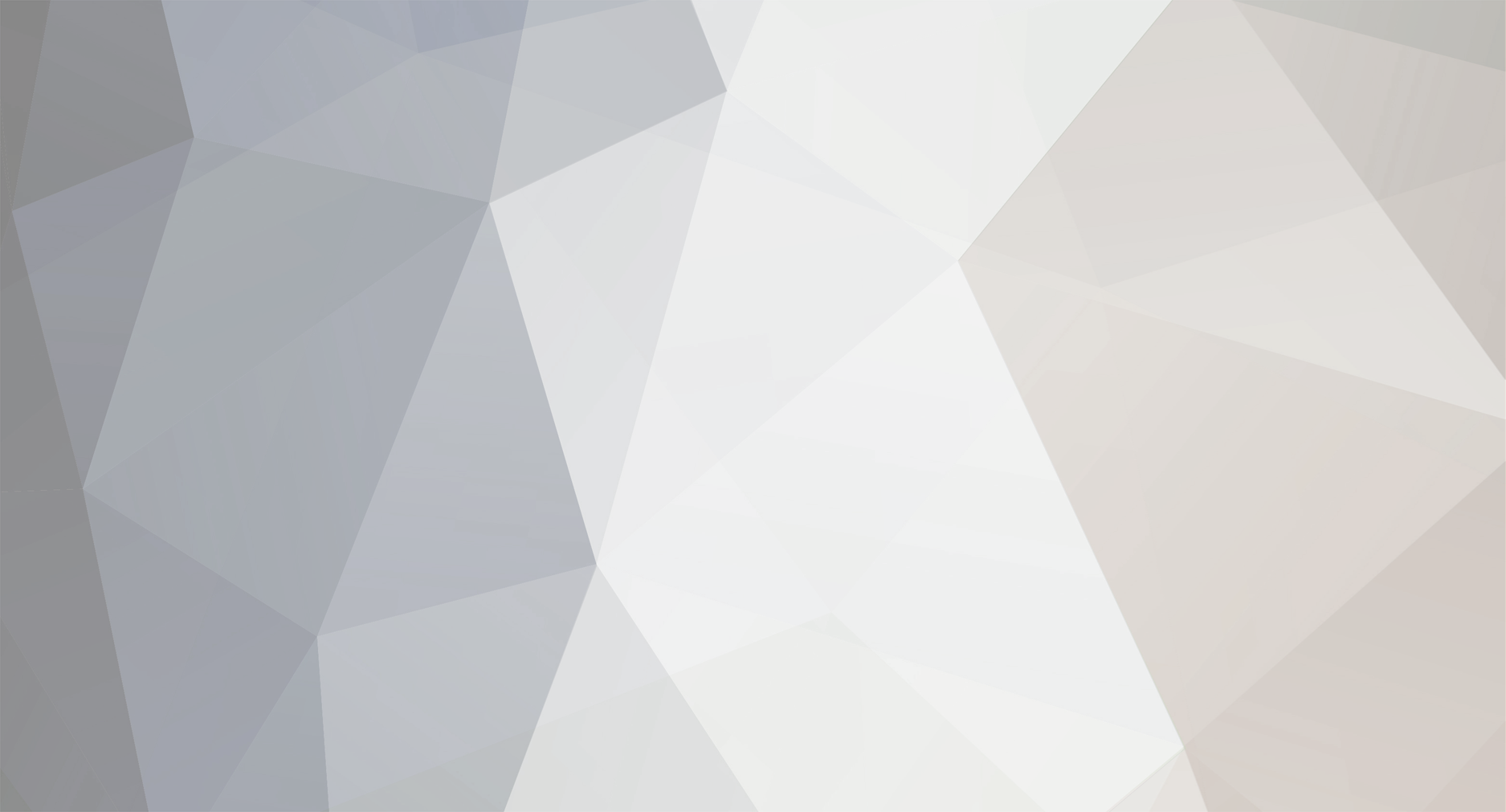
3aGl3
Members-
Posts
128 -
Joined
-
Last visited
Everything posted by 3aGl3
-
Yes. The function guiGetScreenSize will return the players game resolution, thus screenW will be the width of his screen (window if he plays in windowed mode). So all you have to di is decide which elements should snap to the right of the screen and which to the left. Of course you can also use screenH to make something align with the bottom of the screen in a similar fashion.
-
You will have to get the screens size with something like this: screenW, screenH = guiGetScreenSize() After that you can offset everything from that, for example to place something on the right side of the screen: local width = 128 local height = 32 local x = screenW - width - 10 -- 10 so it has some space to the right local y = 50 dxDrawRectangle( x, y, width, height )
-
If they have the key for it, sure. Another option would be to have the file on the server side and only cache it on the client side. Although I don't think clients should have any sensitive data at all saved to their disk, their password (encrypted ofc) would be the only sensitive data I'd let a client save...so you might want to think of another way to do this.
-
You need to trigger the client event onto the same element. For example on the server side: triggerClientEvent( client, "onClientNeedsThingsDone", resourceRoot, some_variable ) and on the client side you will in turn need: addEvent( "onClientNeedsThingsDone", true ) -- true makes sure you can trigger it from the server side addEventHandler( "onClientNeedsThingsDone", resourceRoot, getThingsDone ) Note that I attached the handler to the resourceRoot and triggered the event on the resourceRoot. To make sure only the right player gets the event there is the first variable in the triggerClientEvent, which can either be an element or a table.
-
Don't forget to also edit the .col and remove the gate there as well.
-
The getAllElementData function returns only the data set with setElementData. Given that the wiki states width, depth and height as the attributes used in map files I'd say that are the names of the values you are looking for.
-
You'll either have to make a custom model or get one somewhere. As this has literally nothing to do with scripting I'd say this is the wrong forum for it...
-
"not nil" will be true, so the script will start. A couple lines later the variable is set, so not true will be false and the content of the if won't execute again unless the variable is set to false or nil again...
-
The entire way you do it is the error. You shouldn't create a new table for every player that registers... Also you should never use .. to create SQL querys...like this your database is very open to SQL injections. The proper way to do it would be a table with vehicles and a column with the account name to identify the vehicle owner.
-
As you are never removing the unnamed function from onClientRender it will start everythign all over again once it's done. You can either name the function that starts the rendering and remove it as well or you should not clear the Debounce variable... Comment line 19 in your original script and it should work.
-
The wiki states there are "only" 65535 dimensions. I never tried to go even that far but what is correct now?
-
It seems like you have a SQL error rather than a script error.
-
Maybe I'm missing it but what exactly is your question?
-
If you want some serious tool to edit your MySQL database your Navicat Lite. But yes, generally you can edit your database while it's running, even with phpmyadmin.
-
As you're not giving more infos on your database structure here is how I would do it: local top_players = databaseQueryRaw( "SELECT * FROM `player` ORDER BY `money` ASC;" ) That will give you a table with all players data ordered by their money value.
-
First of all let's start with some very basic things about a login system. Please for the love of your users, do NOT save passwords in cleartext. MTA provides the sha256 as well as hash functions, make use of them. Also get a random salt when the user registers and also store that in your database. Every other way of storing passwords is completely insecure. So let's get to your question now that we talked about that. 1. When a player logs in he will select a character, at that point you want to load the characters data from the table 2. When a player logs out you will want to save the data to the database again, also it's probably a good idea to do it when he changes dimensions, ints etc. just in case something unexpected happens Here is an example: After selecting a character to spawn the player function playerSpawn( player ) -- check if the player is actually logged in if not player.account or player.account.guest then outputDebugString( "Player is not logged in when trying to spawn.", 1 ) return end -- get a list of characters related to the account local pdata = databaseQueryRaw( "SELECT * FROM `player` WHERE `account`=? ORDER BY `player_id` ASC;", player.account.name ) if pdata then -- get the selected character local c = player.account:getData( "character" ) -- pick the correct character or fall back if not c or not pdata[tonumber(c)] then pdata = pdata[1] else pdata = pdata[c] end -- get the players team local team = getTeamFromName( LOC_team_player ) if isObjectInACLGroup( "user.".. player.account.name, aclGetGroup( "Admin" ) ) then -- ToDo: We might want a better way to find teammember team = getTeamFromName( LOC_team_admin ) end -- set the players object ID player.id = PLAYER_ID_PREFIX .. "-" .. pdata.player_id -- get the skin based on the character and gender local skin = getCharacterSkin( pdata.gender, pdata.char ) if pdata.pos_x and pdata.pos_y and pdata.pos_z and pdata.pos_r and pdata.int and pdata.dim then -- spawn the player at his last know position spawnPlayer( player, pdata.pos_x, pdata.pos_y, pdata.pos_z, pdata.pos_r, skin, pdata.int, pdata.dim, team ) else local spawnpoints = getElementsByType( "spawnpoint" ) if spawnpoints and #spawnpoints > 0 then -- if we found spawnpoints -- select a random spawnpoint local spawnpoint = spawnpoints[math.random(#spawnpoints)] local pos_x, pos_y, pos_z = getElementPosition( spawnpoint ) local pos_r = getElementRotation(spawnpoint) or 0 local int = getElementInterior( spawnpoint ) or 0 local dim = getElementDimension( spawnpoint ) or 0 -- spawn the player at that position spawnPlayer( player, pos_x, pos_y, pos_z, pos_r, skin, int, dim, team ) else outputDebugString( "No spawnpoint found, falling back.", 2 ) spawnPlayer( player, fallback.x, fallback.y, fallback.z, 0, skin, pdata.int, pdata.dim, team ) end end else -- open the character creation clientCall( player, "openCharacterCreation" ) return end -- focus the clients camera and show his HUD setCameraTarget( player, player ) setPlayerHudComponentVisible( player, "all", true ) end Note that in the example I was storing the character in an account data previously. To save the location: function playerLocationSave( player, account ) account = account or player.account -- make sure the account is valid if not account or account.guest then outputDebugString( "'playerLocationSave' called on player with an invalid account.", 1 ) return end -- gather all the info about the player that has to be saved local pos_x, pos_y, pos_z = getElementPosition( player ) local rot_x, rot_y, rot_z = getElementRotation( player ) local dim = getElementDimension( player ) local int = getElementInterior( player ) -- get a list of characters related to the account local chars = databaseQueryRaw( "SELECT * FROM `player` WHERE `account`=? ORDER BY `player_id` ASC;", player.account.name ) if chars then -- try to get the selected character local c = player.account:getData( "character" ) -- pick the correct character or fall back if not c or not chars[tonumber(c)] then chars = chars[1] else chars = chars[c] end -- save the data to the database databaseQueryRaw( "UPDATE `player` SET `pos_x`=?, `pos_y`=?, `pos_z`=?, `pos_r`=?, `dim`=?, `int`=? WHERE `player_id`=?", pos_x, pos_y, pos_z, rot_z, dim, int, chars.player_id ) end end
-
Oh yes, indeed. I just quickly looked it up in the wiki and missed the weapon-skill
-
I think I will use the very last dimension as a sort of "trashcan".
-
Thanks for the reply. I guess I'll just use a different dimension then.
-
The wiki states: So I would assume that setWeaponProperty( "minigun", "anim_loop_stop", 0.5 ) setWeaponProperty( "minigun", "anim2_loop_stop", 0.5 ) will change the fire rate for aimed and hip fire.
-
Quote from the Wiki: Yet you are using state == "down"