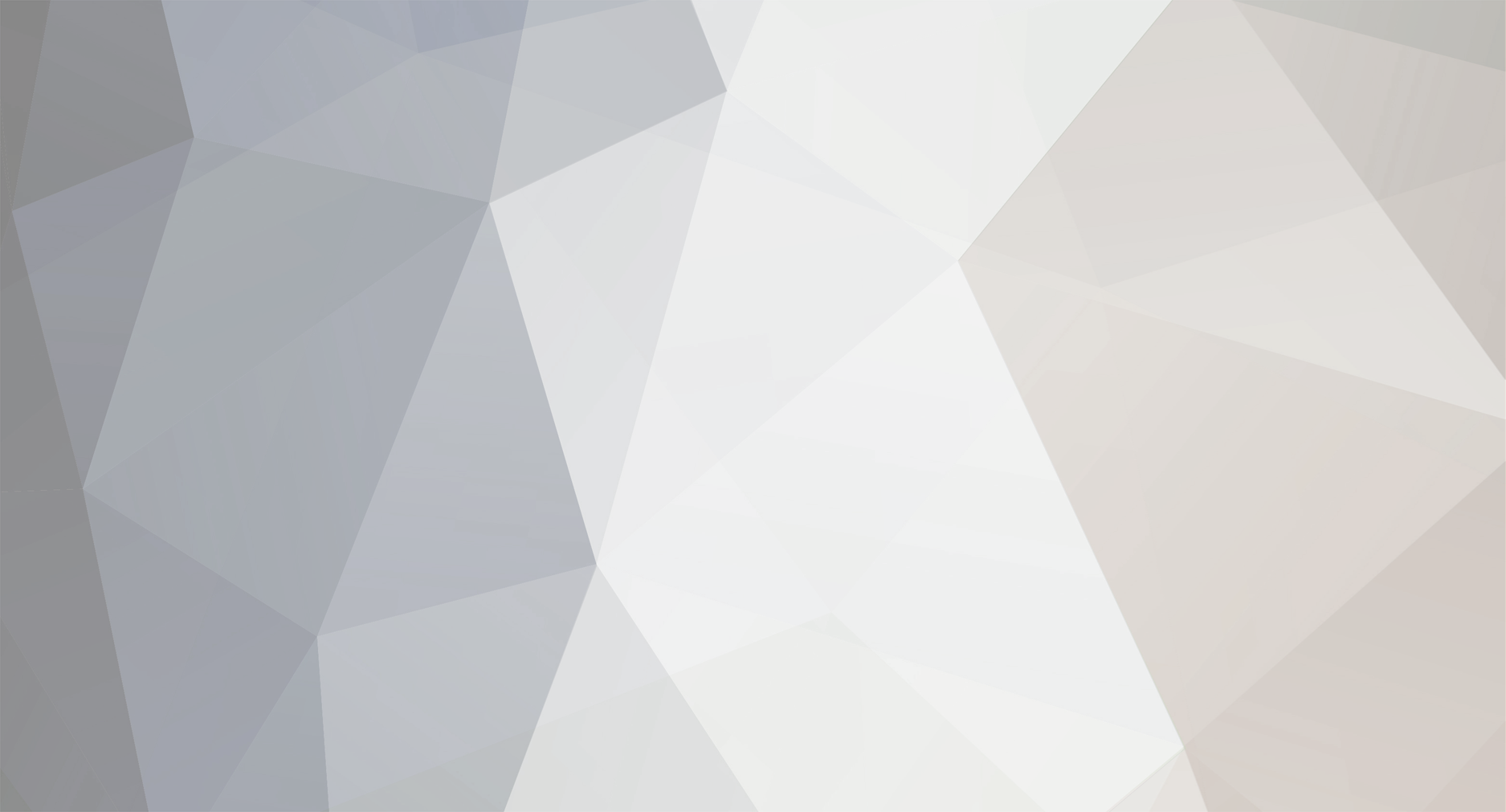
cheez3d
Members-
Posts
290 -
Joined
-
Last visited
Everything posted by cheez3d
-
Handler function parameters These are the parameters for the handler function that is called when the command is used. Server player playerSource, string commandName, [string arg1, string arg2, ...] playerSource: The player who triggered the command. If not triggered by a player (e.g. by admin), this will be false. commandName: The name of the command triggered. This is useful if multiple commands go through one function. arg1, arg2, ...: Each word after command name in the original command is passed here in a seperate variable. If there is no value for an argument, its variable will contain nil. You can deal with a variable number of arguments using the vararg expression, as shown in Server Example 2 below. Client string commandName, [string arg1, string arg2, ...] commandName: The name of the command triggered. This is useful if multiple commands go through one function. arg1, arg2, ...: Each word after command name in the original command is passed here in a seperate variable. If there is no value for an argument, its variable will contain nil. You can deal with a variable number of arguments using the vararg expression, as shown in Server Example 2 below. That is why Solidsnake used function(thePlayer,command,id) For example, if you use the command /goto 11 thePlayer will be the player element that requested the command, the command will be a string : "goto" and the id will be an argument specified by you, in this case 11.
-
Let's say that the mp3 is in %resourcedir%/audio/files <file src="audio/files/sound.mp3"/>
-
function ranks() local rankico = getElementData(localPlayer, "Rankicon") if rankico then setElementData(localPlayer, "Rank",":levelsystem/icons/"..rankico..".png") else setElementData(localPlayer, "Rank",":levelsystem/icons/private.png") end end setTimer(ranks, 500, 0)
-
Here is another example. It will display your FPS every second on the chat. local frames = 0 local time = false function startFrames() time = getTickCount() addEventHandler("onClientRender",root,countFrames) end function countFrames() if getTickCount()-time>=1000 then -- if a second passed from the last check outputChatBox(tostring(frames)) -- output FPS time = getTickCount() -- set the time to getTickCount() frames = 0 -- reset frames end frames = frames+1 -- increment frames every onClientRender until 1 second is reached end startFrames()
-
countPlayersInArena = function(arenaName) local playerCount = 0 for _,player in ipairs (getElementsByType("player")) do if getElementData(player,"Arena") == arenaName then playerCount = playerCount+1 end end return playerCount end setTimer(function() guiSetText(label_totalPlayers,#getElementsByType("player").." players online") guiSetText(label_freeroamPlayers,"Online: "..tostring(countPlayersInArena("Freeroam"))) guiSetText(label_currentArena,getElementData(localPlayer,"Arena")) guiSetText(label_playerName,getPlayerName(localPlayer)) guiSetText(label_playerMoney,"$"..convertNumber(getPlayerMoney(localPlayer))) end,5000,0) There was a typo.
-
I edited the code above. It should work now.
-
You're updating the labels too much. Every 5-10 seconds should be fine. contPlayersInArena = function(arenaName) local playerCount = 0 for _,player in ipairs (getElementsByType("player")) do if getElementData(player,"Arena") == arenaName then playerCount = playerCount+1 end end return playerCount end setTimer(function() guiSetText(label_totalPlayers,#getElementsByType("player").." players online") guiSetText(label_freeroamPlayers,"Online: "..tostring(countPlayersInArena("Freeroam"))) guiSetText(label_currentArena,getElementData(localPlayer,"Arena")) guiSetText(label_playerName,getPlayerName(localPlayer)) guiSetText(label_playerMoney,"$"..convertNumber(getPlayerMoney(localPlayer))) end,5000,0)
-
Can you post the rest of the code and the errors that you receive?
-
contPlayersInArena(arenaName) local pCount = 0 for _,player in ipairs (getElementsByType("player")) do if getElementData(player,"Arena") == arenaName then pCount = pCount+1 end return pCount end local freeroamCount = countPlayersInArena("Freeroam") outputChatBox(tostring(freeroamCount),root)
-
local pCount = 0 for _,player in ipairs (getElementsByType("player")) do if getElementData(player,"Arena") == "Freeroam" then pCount = pCount+1 end end Just a basic example.
-
showcaseObject = function(object) local rotationProgress = 0 local function rotateObject() rotationProgress = rotationProgress+0.01 local rotationValue = interpolateBetween(0,0,0,360,0,0,rotationProgress,"InOutQuad") local _,y,z = getElementRotation(object) setElementRotation(object,rotationValue,y,z) if rotationProgress>=1 then rotationProgress = 0 end end addEventHandler("onClientRender",root,rotateObject) end
-
This is not a request section. Use these functions: addCommandHandler() takePlayerMoney() setElementHealth()
-
local marker = createMarker(someX,someY,someZ) addEventHandler("onMarkerHit",marker,function(element,dimension) if getElementType(element) == "player" and getElementDimension(element) == dimension and not isPedInVehicle(element) then local x,y,z = getElementPosition(marker) local vehicle = createVehicle(432,x,y,z) warpPedIntoVehicle(element,vehicle,0) outputChatBox("You received a car!",element) end end)
-
SELECT * FROM `sometable` WHERE `somecolum` IS NOT NULL
-
You made a typo: health NUMBERIC instead of NUMERIC
-
addEventHandler("onVehicleExplode",root,function() destroyElement(source) end)
-
use stopSound() or destroyElement()
-
Did you read the page abut the function? I don't think so. https://wiki.multitheftauto.com/wiki/interpolateBetween
-
bindKey's third argument must be a function. If you use triggerServerEvent as the 3rd argument it will return true or false.
-
assingIDToPlayer = function() local connectedPlayers = getElementsByType("player") local assignedID = #connectedPlayers+1 local checkIDUniqueness = function() for _,player in ipairs (connectedPlayers) do if getElementData(player,"ID") == assignedID then assignedID = assignedID+1 checkIDUniqueness() break end setElementData(source,"ID",assignedID) end end checkIDUniqueness() end addEventHandler("onPlayerJoin",root,assingIDToPlayer) kickPlayerByID = function(player,command,id,...) local reason = {...} if id then for _,target in ipairs (getElementsByType("player")) do outputChatBox(id,player) outputChatBox(getElementData(player,"ID"),player) if tostring(getElementData(player,"ID")) == id then kickPlayer(target,player,(table.concat(reason," ") ~= "" and table.concat(reason," ") or "No reason specified!")) outputChatBox("INFO - "..player.." kicked "..target..", reason - "..(table.concat(reason," ") ~= "" and table.concat(reason," ") or "No reason specified!"),root,255,255,255) end end else outputChatBox("ERROR - No ID specified!",player,255,0,0) outputChatBox("SYNTAX: /ikick [reason]",player,0,255,0) end end addCommandHandler("ikick",kickPlayerByID)
-
fadeScoreboard = function(keyState) local fadeProgress = 0 if keyState == "down" then fadeProgress = fadeProgress+0.01 local alphaValue = interpolateBetween(0,0,0,255,0,0,fadeProgress,"InOutQuad") --dxDrawStuff + tocolor(r,g,b,alphaValue) if alphaValue>=1 then removeEventHandler("onClientRender",root,renderScoreboard) end elseif keyState == "up" then fadeProgress = fadeProgress-0.01 local alphaValue = interpolateBetween(0,0,0,255,0,0,fadeProgress,"InOutQuad") --dxDrawStuff + tocolor(r,g,b,alphaValue) if alphaValue<=0 then removeEventHandler("onClientRender",root,renderScoreboard) end end end addEventHandler("onPlayerJoin",root,function() bindKey(source,"tab","both",function(_,keyState) function renderScoreboard() fadeScoreboard(keyState) end addEventHandler("onClientRender",root,renderScoreboard) end) end)
-
Then you have to create your own ID system. getPlayerFromID - there is no such function I suggest setElementData and getElementData for the ID system
-
playSFX3D This function was added with a reason.