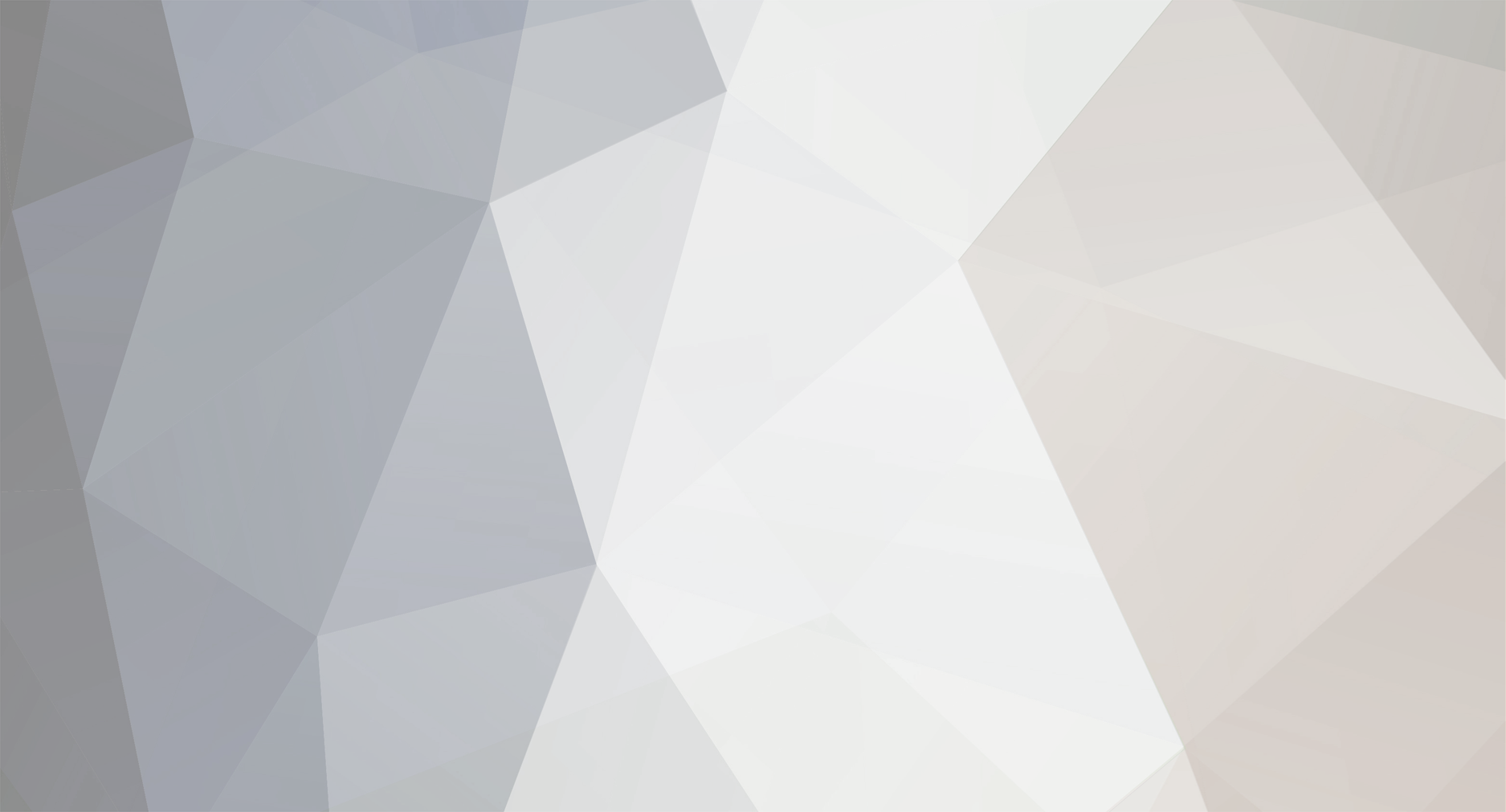
Dealman
Members-
Posts
1,421 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Dealman
-
You can read more about this on the Wiki page; Writing Gamemodes
-
For quite some time now, I've been experiencing issues with this forum. Even if I set it to log me in automatically and I choose to remember my password with my browser when prompted, I'm always logged out after a few minutes. Also to properly log in, I have to log in twice in a row. First attempt will just boot me back to index, while the second one will successfully log me in. Another minor issue with the website is that it always seem to want to send you back to the index, this can be particularly annoying if you want to reply to a thread - but need to log in, then you've gotta dig that thread up again. I'm using Google Chrome at the moment, but were experiencing this with Opera as well.
-
I'd suggest you read thoroughly what I said before, you seem to have misunderstood all of it. First of all, dxDrawRectangle returns a boolean - either true or false. If it was drawn successfully it returns true, if not - it returns false. Secondly, you can't create an element using a boolean. Always read about the function on the wiki, don't just assume it will magically work. Also, I would advice against you trying to create elements as it's a very time consuming task, and it will only do you good if you make an entire library. Thirdly, I would advice you to structure your code differently. Writing it like that has no benefit and just ends up being harder to read, I'd suggest you read my tutorial. Fourth, you're using createElement inside onClientRender. Always keep in mind that rendering will be done at anywhere between 30 to 60 times per second(I think 100 FPS is the limit). So at 60 FPS, you would be trying to create a new element every ~16ms. Fifth, you were checking if the width was greater than 532. You're interpolating it between 0 and 532, thus, it would stop at 532 - and your if statement would never be triggered. Instead, you'd want to use greater than or equal to. Sixth, personally, this is how I would've written it; local startTick = getTickCount() function DrawProgress() local nowTick = getTickCount() local progressWidth = interpolateBetween(0, 0, 0, 532, 0, 0, (nowTick - startTick) / ((startTick + 10000) - startTick), "Linear") if(progressWidth >= 532) then triggerServerEvent("payMoney", localPlayer) removeEventHandler("onClientRender", root, DrawProgress) -- Progress has reached 1.0, trigger the event, and then remove the render event to stop rendering. else dxDrawRectangle(142, 222, progressWidth, 41, tocolor(23, 23, 231, 255), false) end end addEventHandler("onClientRender", root, DrawProgress) Edit: You beat me to it
-
What he was trying to say is that all the gui-related functions only work with GUI elements. For example guiCreateWindow, guiCreateButton and the like. All of those return a GUI Element. DX drawings are drawings that are rendered each frame and as such does not return any elements. So this is why you can't use guiSetVisible to hide or show a DX drawing. Instead, you will have to use either addEventHandler or removeEventHandler. Or alternatively, you can simply use an if statement. Of course, you can write your own library to create custom elements using createElement. Thus, you can achieve the same behavior as you do with CEGUI but instead with DX Drawings. There are already a few of those out there, but I don't think either of them were ever finished. More so, if you read about dxDrawRectangle on the MTA Wiki, you'd see that it either returns true or false. So basically you would be doing this; guiSetVisible(true, false) When the correct usage would be; guiSetVisible(guiElement, false)
-
That's an error in your code, not mine. Do you even read the error? lua:4: attempt to concatnate local 'stunt'type (a nil value) This means the error occurred at Line 4 in your code. The variable stuntType is returning a nil value - which means it's empty or doesn't exist.
-
Because this code is never executed; function nothing () addEventHandler("onClientRender",root,lol) end Try this; local screenW,screenH = guiGetScreenSize () function lol(stuntType, stuntTime, distance) dxDrawText("You finished stunt: " .. stuntType ..", Time: " .. tostring( stuntTime ).. ", Distance: " .. tostring( distance ), screenW * 0.2219, screenH * 0.7935, screenW * 0.8839, screenH * 0.8963, tocolor(255, 255, 255, 255), 1.00, "bankgothic", "left", "top", false, false, false, false, false); end function nothing() addEventHandler("onClientRender", root, lol) end addEventHandler("onClientPlayerStuntFinish", root, nothing)
-
You forgot the parenthesis at the end of your function. I'd highly recommend you check out the Debugging section of the Wiki. I'd argue against writing your code like that, and instead write it like this; function ExampleCode(stuntType, stuntTime, distance) -- Code here end addEventHandler("onClientPlayerStuntFinish", root, ExampleCode) Personally I think it's much easier to read your own code when structured this way instead.
-
Ooh, very interesting! I'll definitely look into implementing this for my upcoming server. Have you done any performance tests with this? Also, please make the roadshine optional if you do implement it - as I recall the roadshine is far too shiny to even be remotely realistic.
-
You misplaced the e in Michael's name
-
There's a multitude of ways you can define what player to trigger the event for. You're absolutely right about onMarkerHit and hitElement, as the Wiki mentions; This can be either a player, ped, or a vehicle. So to verify that it is a player element, you can get getElementType. Or you can check what player is driving the vehicle in which hit the marker.
-
I think he misunderstood how to use the function triggerClientEvent(elementToSendTo, "eventNameToTrigger", sourceElement, Argument1, Argument2) The first one can either be a player of your choice, by using for example getPlayerFromName. Or you can leave it empty which defaults to root - meaning every player currently on the server. "eventNameToTrigger" - this is the event that you will be triggering. You create this event via addEvent and handle the event via addEventHandler. sourceElement - is the source of the element, what triggered it. Usually it's fine to just leave this at resourceRoot. Arguments - These are arguments you can pass on, such as variables or tables. -- Client: function ExampleFunction_Client(Text1, Text2) -- Make sure you retrieve any data, they will act as local variables, you can name these whatever you want -- Text1 = "Hello" -- Text2 = "World" end addEvent("exampleEvent", true) -- Create the event, "exampleEvent". true means that it can be remotely triggered addEventHandler("exampleEvent", root, ExampleFunction_Client) -- Of course we need an event handler to handle the event, attach it to the function(s) you want -- Server: function ExampleFunction_Server() triggerClientEvent(root, "exampleEvent", resourceRoot, "Hello", "World") -- This triggers for all players triggerClientEvent("exampleEvent", resourceRoot, "Hello", "World") -- Same as above ^ triggerClientEvent(getRandomPlayer(), "exampleEvent", resourceRoot, "Hello", "World") -- This triggers for a random player, and that player only end I've thought about maybe making a video tutorial explaining how to use these functions and communicate between Client & Server since it's a rather important aspect of scripting. It's really easy once you've done it a few times
-
Could always try to do it yourself Since you want it to revert to the old skin ID when you go off-duty, you'll need to define a variable where you would store this ID. getElementModel should return the current skin ID. So when someone goes on-duty, you would store their current skin before setting the new one. And when they later go off-duty, you set their skin ID to their old one again - which you have conveniently stored in a variable.
-
Ooh interesting, I was really confused as to why it wasn't working for me. Now that I look at that function I guess it makes sense.
-
guiSetVisible or guiSetEnabled will disable that GUI element. You'll have to dig through the code to find it first though.
-
Differences aren't too big as far as I'm aware. I'd argue that the CEGUI one is easier to work with, since mouse input and all that stuff is already working. Whereas with the DX Browser, you'll have to inject it manually(though this also gives a bit more control). It's also drawn using dxDrawImage so you can do fancy stuff with it. I've found that text inputs do not seem to work with the DX browser at all and I can't figure out why. Thus, I've been using the CEGUI version instead.
-
Use setWorldSoundEnabled to mute vehicle sounds. As for shadows, I don't think so.
-
You might wanna look into JR10's resource NPP. It should get you all the function and all that good stuff you need. I used this myself when I was writing my own MTA-Lua language definition for N++. I've always been loyal to N++ despite their poor support for Lua(at least custom versions of Lua). But I might check this one out some time. I've been too scared to try other editors since I love the Zenburn theme of N++ Edit: I saw there were a few zenburn based themes so I went ahead and gave it a go. It's actually pretty darn solid, very sleek interface design. And there's a whole lot of addons to be found. I would highly, highly recommend the "minimap" and the color-picker. I'd gladly help contribute to this, but I've barely used GitHub other than to host my own repositories. So I'd need to look up how contributions actually work so I don't break anything
-
Simply put the code you want to execute once the timer's done inside that timer. Otherwise, you can use getTimerDetailsto see if it's still running, stopped running or does not exist.
-
No that is not helpful as it's how many zombies will spawn per player. I mean the actual function that spawns the zombies. Look for createPed.
-
Try using a cloud service such as Kiwi6?
-
Replace the spaces with %20 inside of the Lua script as well? You can use string.gsub for this.
-
You'll need to find the function that actually spawns the zombies to begin with.
-
As for uploading the file somewhere you can use services such as Mediafire and Dropbox, I believe Mediafire lets you use direct links if you subscribe. Then you just use playSound like usual, but put in the URL instead of the filepath. Personally I have always used Kiwi6 to stream files. As for YouTube, you can do this by using the new CEF Browser introduced with MTA 1.5
-
The only way to accomplish this outside of editing the game files is to disable the default sound via setWorldSoundEnabled. You would then need to write your own engine simulation. I made this a while ago, however I abandoned it as it proved difficult to accomplish and didn't add that much to the game in my opinion.