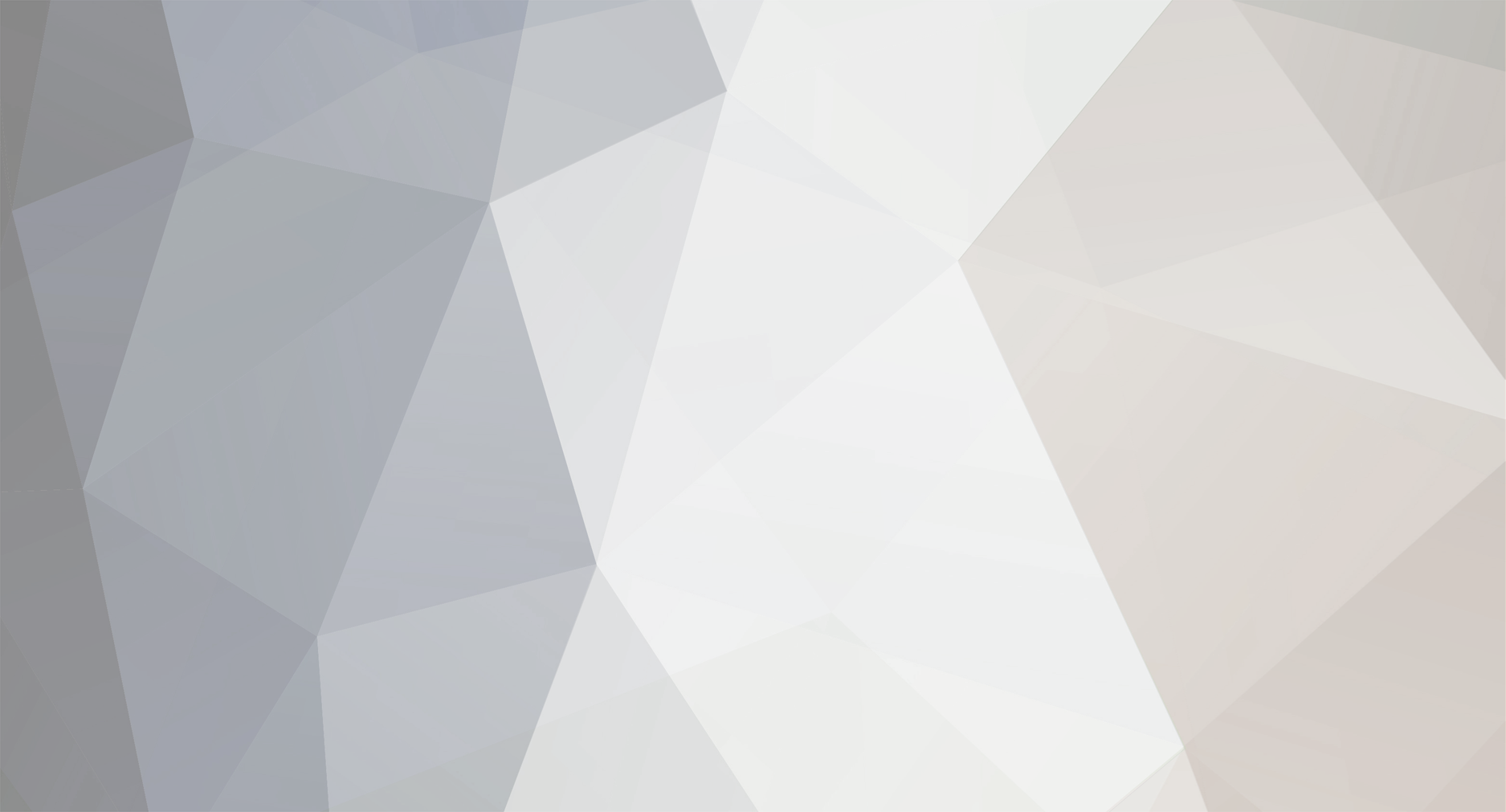
pa3ck
Members-
Posts
1,141 -
Joined
-
Last visited
-
Days Won
7
Everything posted by pa3ck
-
It's only couple of lines of code, the loop is pretty small as well (probably between 10 and 50, don't think there would be more), couple of ms and it's done... But if you are that concerned about performance, you can try this: local teams = {"Team1", "Team2", "Team3", "Team4"} local allowedPlayers = {} addEventHandler("onPlayerQuit", root, function() if allowedPlayers[source] then allowedPlayers[source] = nil end end) function RadioChat(thePlayer, _, ...) if not allowedPlayers[thePlayer] or not isPlayerInAllowedTeam(thePlayer) then outputChatBox("You are not in the list of allowed teams", thePlayer) return end local message = table.concat ( { ... }, " " ) for i=1, #teams do local team = getTeamFromName(teams[i]) -- teams[i] means, teams[1], teams[2], teams[3], teams[4] (returns the value for each) if team then local name = getPlayerName(thePlayer) local players = getPlayersInTeam ( theTeam ) for playerKey, playerValue in ipairs ( players ) do outputChatBox("(*RADIO*) " .. getPlayerName ( thePlayer ) .. " #ffffff"..message, playerValue, 0, 0, 255, true) end end end end function isPlayerInAllowedTeam(player) local isAllowed = false for k, v in ipairs(teams) do if getPlayerTeam(player) == getTeamFromName(v) then isAllowed = true if not allowedPlayers[player] then allowedPlayers[player] = true end break end end return isAllowed end
-
local teams = {"Team1", "Team2", "Team3", "Team4"} function RadioChat(thePlayer, _, ...) if not isPlayerInAllowedTeam(thePlayer) then outputChatBox("You are not in the list of allowed teams", thePlayer) return end local message = table.concat ( { ... }, " " ) for i=1, #teams do local team = getTeamFromName(teams[i]) -- teams[i] means, teams[1], teams[2], teams[3], teams[4] (returns the value for each) if team then local name = getPlayerName(thePlayer) local players = getPlayersInTeam ( theTeam ) for playerKey, playerValue in ipairs ( players ) do outputChatBox("(*RADIO*) " .. getPlayerName ( thePlayer ) .. " #ffffff"..message, playerValue, 0, 0, 255, true) end end end end function isPlayerInAllowedTeam(player) local isAllowed = false for k, v in ipairs(teams) do if getPlayerTeam(player) == getTeamFromName(v) then isAllowed = true break end end return isAllowed end That should solve it
-
I'm not too good with shaders, but it's worth a try... try to remove the if statement that checks if it's the localPlayer. Because you will only change the shader for the local player, the code will never run for others. If you use getPedOccupiedVehicle(v), you will still only change the localPlayer's vehicle, but others should see it as well, the big if here is, can MTA restrict it to a single vehicle... -- server function setNitroColor(r, g, b, player) triggerClientEvent(root , "Nitrochange", root, r, g, b, player) end -- client function nitroColor(r, g, b, v) local shader = dxCreateShader(":CCS/models/nitro.fx") dxSetShaderValue (shader, "gNitroColor", r/255, g/255, b/255 ) engineApplyShaderToWorldTexture (shader,"smoke", getPedOccupiedVehicle(v)) end addEvent( "Nitrochange", true) addEventHandler("Nitrochange", root, nitroColor)
-
'#' gets the length (number of data entries) in the return table. It is recommended that you use callback functions when working with dbQuery (you will need to use dbQuery, dbExec is for inserts/updates/deletes etc). dbQuery( function (qh) local result = dbPoll( qh, 0 ) -- Timeout doesn't matter here because the result will always be ready if #result > 0 then -- you need to check the length of the table because even if there is nothing found, result will always be true for index, rows in ipairs(result) do -- loop through the returned rows outputChatBox(rows["YOUR_DB_COLUMN_NAME_1"]) outputChatBox(rows["YOUR_DB_COLUMN_NAME_2"]) outputChatBox(rows["YOUR_DB_COLUMN_NAME_3"]) end else outputChatBox("0 results found") end end, connection, "SELECT * FROM YOUR_DB_TABLE_NAME" ) The structure of the returned data will have nested tables. Example: local returnedData = { { ["username"] = "my_username_1", ["password"] = "my_pass_1", ["serial"] = "my_serial_1"}, }, { ["username"] = "my_username_2", ["password"] = "my_pass_2", ["serial"] = "my_serial_2"}, }, { ["username"] = "my_username_3", ["password"] = "my_pass_3", ["serial"] = "my_serial_3"}, } } --[[ This is the reason why you need to loop through the table and use the value variable like if it was a table returnedData[1][1] --> "my_username_1" returnedData[1][2] --> "my_pass_1" returnedData[1]["username"] --> "my_username_1" returnedData[2]["username"] --> "my_username_2" for k, rows in ipairs(returnedData) do outputChatBox(rows["username"]) end Output: my_username_1 my_username_2 my_username_3 for k, rows in ipairs(returnedData) do outputChatBox(rows["username"] .. " : " .. rows["password"]) end Output: my_username_1 : my_password_1 my_username_2 : my_password_2 my_username_3 : my_password_3 ]]
-
First make sure you keep the sound element in a variable (sound = playSound("...") ) then there is more than likely an event in your code which triggers when the user logs in. If you use the default login system, then there's a server-side event "onPlayerLogin" which you can use to turn off the sound on client-side with a trigger
-
No, labels created with guiCreateLabel don't have that option. You can use a DX framework which lets you do that or you can use dxDrawText with colorCoded parameter set to true, but note that clipping/word breaking will be disabled.
-
That's not a bug, that is how the website was built, it's using POST method to conduct a search, so when you try to navigate back the browser no longer has the data, but it even tells you that. This could be solved by using GET method in the queryString (eg. community.multitheftauto.com/index.php?search=resourceName) but I don't think it will be changed, since it's not that big of a deal. What you can do is, don't open the link just by clicking on it, right click -> open in new tab or click with the middle mouse button on the link and if you wanted to go back, you simply close the tab.
-
The smoothest animation you will ever get is using interpolateBetween, what's wrong with it? Why is that not smooth enough?
-
function ss() if source and getElementType(source) == "player" then -- check if it's been called by onPlayerJoin not onResourceStart bindKey (source, "F5", "down", "öv") else for k,player in ipairs(getElementsByType("player")) do bindKey (player, "F5", "down", "öv") end end end addEventHandler("onResourceStart",resourceRoot,ss) addEventHandler("onPlayerJoin",root,ss)
-
Why would you re-bind the command when somebody joins for every single player?
-
If you mean put it inside the "slothbot" resource, then no, you don't have to put it in there, since it's using exported functions.You can put it into any resource as long as you are using the correct resource name and the resource is running. I sent you the code to do an outputChatBox when the player dies and the 'attacker' is a bot, you can do the same logic to do an outputChatBox when you kill a bot.
-
Put what to the ACL? You don't have to put anything in the ACL
-
It says slothbot is not running.. start the resource, it cannot be renamed, make sure the name of the resource is "slothbot". You can use the function isPedBot to check if the killer is a bot @ event onPlayerWasted. addEventHandler ( "onPlayerWasted", getRootElement(), function(ammo, attacker) if attacker and getElementType(attacker) == "ped" and exports [ "slothbot" ]:isPedbot(attacker) then local name = getPlayerName(source) outputChatBox("Player " .. name .. " was killed by a bot") end end)
-
function boss (player,comando,weaponid,skinid, ...) if not hasObjectPermissionTo (player, "command.ban") then -- you might want to add your message here return end local teamName = table.concat({...}," ") local team = getTeamFromName(teamName) if not team then team = createTeam(teamName) end local x,y,z = getElementPosition(player) slothbot1 = exports [ "slothbot" ]:spawnBot ( x,y,z , 90, tonumber(weaponid), 0, 0, team, tonumber(skinid), "hunting", true ) end addCommandHandler("spawnbot",boss) That should work
-
Yea, I didn't think about that.. try this: function boss (player,comando,weaponid,skinid, ...) local teamName = table.concat({...}," ") local team = getTeamFromName(teamName) if not team then team = createTeam(teamName) end local x,y,z = getElementPosition(player) slothbot1 = exports [ "slothbot" ]:spawnBot ( x,y,z , 90, tonumber(weaponid), 0, 0, team, tonumber(skinid), "hunting", true ) end addCommandHandler("spawnbot",boss)
-
Sorry, I didn't see your reply. Looks like the problem happens when I do a manual tab in the code editor. If you look at the code editor below and the code I will insert, you will see what I mean. I'm using Google Chrome Version 56.0.2924.87 (from work, not able to update) but I sometimes use IE as well, that is when I have the problem with copying code, but that never happens in Chrome (don't blame me for using IE, needed for production development). But strangely enough it can be fixed by removing the 'tabs' completely, clicking on insert, editing the code again and adding the exact same tabs. function() -> manual tabs if true then if true then myFunction() myFunction() end end end function() -> auto tabs if true then if true then myFunction() myFunction() end end end function() -> manual tabs if true then if true then myFunction() myFunction() end end end
-
function boss (player,comando,weaponid,skinid, teamName) local team = getTeamFromName(teamName) if not team then team = createTeam(teamName) end local x,y,z = getElementPosition(player) slothbot1 = exports [ "slothbot" ]:spawnBot ( x,y,z , 90, tonumber(weaponid), 0, 0, team, tonumber(skinid), "hunting", true ) end addCommandHandler("spawnbot",boss) Try this, I added the last argument 'teamName'. If you add a teamName eg. You type: /spawnbot 22, 10, Criminals -> it will look for the team Criminals and if it doesn't exist, it will create that team and spawn the bot assigned to it.
-
I'm not trying to be a smartass myself, but @DriFtyZ did the right thing. If you look at the new guidelines, you can see, that posts, that include problematic code (so not just a question about how to do x and y ), should be labeled as [BUG] so I'm pretty sure that is why loads of people do that. It's a bit confusing actually, I agree with you, it shouldn't be called [BUG].
-
That's not hooked to a command but to a function.. --client function smt() outputChatbox("smt") end addCommandHandler("smt_command", smt) bindKey("o", "up", "smt_command") On server: --server addEventHandler("onResourceStart", resourceRoot, function() -- in case you restart or start the resource while the server is running for k, v in ipairs(getElementsByType("player")) do bindKey(v, "o", "up", "smt_command") end end) addEventHandler("onPlayerJoin", root, function() -- when somebody new joins and missed the "onResourceStart" event, bind the command to the source only bindKey(source, "o", "up", "smt_command") end) function smt(p) outputChatbox("smt", p) end addCommandHandler("smt_command", smt)
-
Sorting out the zip and stuff should be handled by your website. From lua you can then read in the map data, save it then load it in.
-
fetchRemote that's basically the only thing you need, you read in the file, save it as a map file and load it.