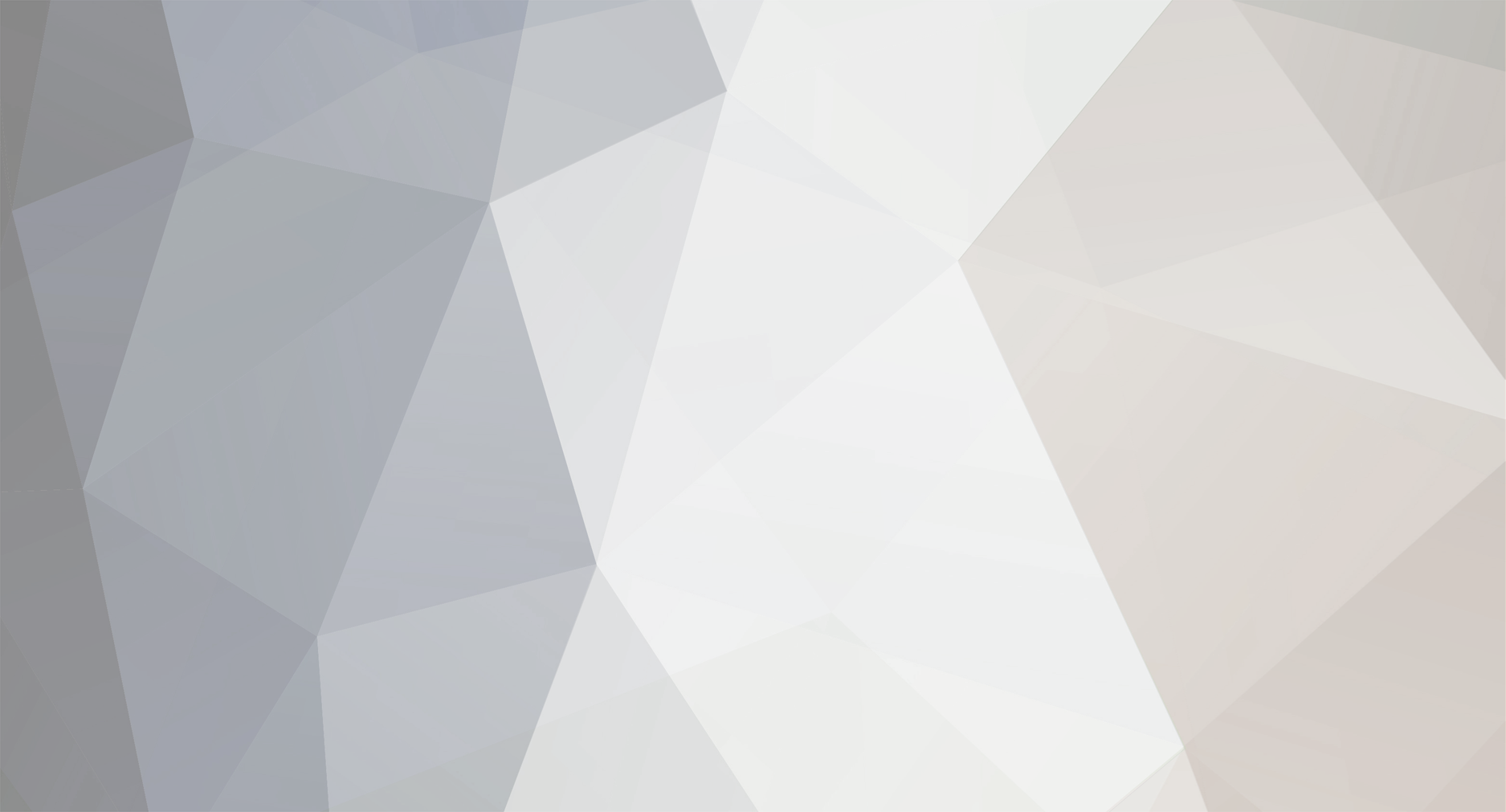
bartje01
-
Posts
208 -
Joined
-
Last visited
Posts posted by bartje01
-
-
Just asking me if this code is mine?/... Jheez.
We do not like the stealing other codes .
You clearly do not understand the meaning of stealing. It's not stealing if the author uploaded it..
-
Just asking me if this code is mine?/... Jheez.
-
Are you sure that this code is yours?
Nope it isn't. And if you need the server sided part:
local running = false -- if race is running addEvent("onClientRequestRespawn", true) addEventHandler("onClientRequestRespawn", getRootElement(), function(vehicleData) -- source is the player that requested respawn. -- spawn at the position where last saved. triggerClientEvent(source, 'onClientCall_race', source, "Spectate.stop", 'manual') triggerEvent('onClientRequestSpectate', source, false) spawnPlayer(source, vehicleData.posX, vehicleData.posY, vehicleData.posZ) local vehicle = exports.race:getPlayerVehicle(source) triggerClientEvent(source, 'onClientCall_race', source, "Spectate.stop", 'manual') setElementData(source, "race.spectating", true) setElementData(source, "status1", "dead") setElementData(source, "status2", "") --setElementData(source, "state", "training") setElementData(source, "race.finished", true) setCameraTarget(source, source) setElementData(vehicle, "race.collideworld", 1) setElementData(vehicle, "race.collideothers", 0) setElementData(source, "race.alpha", 255) setElementData(vehicle, "race.alpha", 255) setElementHealth(vehicle, vehicleData.health) setElementModel(vehicle, 481) -- fix motor sound. setElementModel(vehicle, tonumber(vehicleData.model)) setElementPosition(vehicle, vehicleData.posX, vehicleData.posY, vehicleData.posZ) setElementRotation(vehicle, vehicleData.rotX, vehicleData.rotY, vehicleData.rotZ) if(vehicleData.nitro ~= nil)then addVehicleUpgrade(vehicle, tonumber(vehicleData.nitro)) end setElementFrozen(vehicle, true) toggleAllControls(source, true) setVehicleLandingGearDown(vehicle, true) setTimer(delayedRespawn, 2000, 1, source, vehicle, vehicleData) end) function delayedRespawn(player, vehicle, vehicleData) triggerClientEvent(player, "clientUnfreezeOnReady", player, vehicle, vehicleData) end addEvent("onRaceStateChanging", true) addEventHandler("onRaceStateChanging", getRootElement(), function(newState, oldState) triggerClientEvent("onClientRaceStateChanging", getRootElement(), newState, oldState) if(newState == "Running")then running = true end if(newState == "PostFinish" or newState == "NoMap")then running = false local player = getElementsByType("player") for i = 1, #player do local replaying = getElementData(player[i], "respawn.playing") if(replaying)then setElementData(source, "race.spectating", false) setElementData(source, "status1", "dead") setElementData(source, "status2", "") setElementData(source, "race.finished", false) end end end end) -- Add training mode before player has played once addEventHandler("onElementDataChange", getRootElement(), function(theName, oldValue) if(getElementType(source) == "player")then if(tostring(getElementData(source, "state")) == "waiting" and running)then --triggerClientEvent(source, "onClientRaceStateChanging", source, "Running", "GridCountdown") end end end) -- Kill when respawned and gets hunter. addEvent("onPlayerPickUpRacePickup", true) addEventHandler("onPlayerPickUpRacePickup", getRootElement(), function(pickupID, pickupType, vehicleModel) if(pickupType == "vehiclechange" and vehicleModel == 425)then local state = getElementData(source, "state") or "dead" if(state == "dead")then setElementHealth(source, 0) end end end) addEventHandler("onClientRender", getRootElement(), function() local player = getElementsByType("player") for i = 1, #player do if(getElementData(player[i], "respawn.playing"))then local alpha = 0 if(player[i] == getLocalPlayer())then alpha = getElementData(player[i], "race.alpha") or 255 end setElementAlpha(player[i], alpha) local vehicle = getPedOccupiedVehicle(player[i]) if(vehicle ~= false)then end end end end)
-
anyone able to help me before sleeping time?
-
Hey all. How can I make this respawn script respawning people without a vehicle?
local screen_width, screen_height = guiGetScreenSize()
local RESPAWN_KEY = "F"
local showRespawn = false
local text = "#FF5500Press #FFFFFF"..RESPAWN_KEY.." #FF5500to enter to training mode"
local scale = 1.5
local font = "bankgothic"
local textWidth = dxGetTextWidth(text:gsub("#%x%x%x%x%x%x", ""), scale, font)
local fontHeight = dxGetFontHeight(scale, font)
local color = tocolor(255, 128, 0, 255)
setElementData(getLocalPlayer(), "respawn.playing", false, true)
local running = false
local vehicleData = {}
local SAVE_INTER = 22500
local saveTimer = nil
local reset = true -- reset timer
local lastSaved = 0
-- When element data for the player changes, bind or unbind key.
addEventHandler("onClientElementDataChange", getLocalPlayer(),
function(dataName, oldValue)
if(getElementType(source) ~= "player" or dataName ~= "state")then return end
local newValue = getElementData(source, dataName)
if(newValue == "dead")then
reset =true
setTimer(function()
if(#vehicleData > 0 and running)then
showRespawn = true
setElementData(getLocalPlayer(), "respawn.playing", false, true)
bindKey(RESPAWN_KEY, "up", respawn)
end
end,
3000, 1)
else
unbindKey(RESPAWN_KEY, "up", respawn)
showRespawn = false
setElementData(getLocalPlayer(), "respawn.playing", false, true)
end
if(newValue == "alive" and oldValue ~= "alive")then
reset = false
end
end)
-- respawn player, later add "checkpoints"
function respawn()
reset = false
unbindKey(RESPAWN_KEY, "up", respawn)
showRespawn = false
setElementData(getLocalPlayer(), "respawn.playing", true, true)
local index = #vehicleData
local index2 = #vehicleData
-- Save memory and go back to later saved positions if dying too fast.
local timeWasted = math.floor((getTickCount() - lastSaved)/1000)
if(timeWasted <= 7 and index > 1)then
table.remove(vehicleData, index)
index = #vehicleData
outputDebugString("Vehicle data num: "..index.." removed respawn.")
end
outputDebugString("Respawning with vehicle data index: "..index)
triggerServerEvent("onClientRequestRespawn", getLocalPlayer(), vehicleData[index])
if(index2 > 1 and index == index2)then
table.remove(vehicleData, index)
outputDebugString("Vehicle data num: "..index.." removed.")
end
end
addEventHandler("onClientRender", getRootElement(),
function()
if(reset)then
resetTimer(saveTimer)
end
-- Dont show text if player is not allowed to respawn.
if not showRespawn then return end
local x, y = screen_width/2 - textWidth/2, screen_height * 0.75 - fontHeight/2
dxDrawColoredText(text, x, y, screen_width, screen_height, color, scale, font)
end)
-- Save vehicle data, to later spawn with that data.
function saveVehicleData()
local vehicle = getPedOccupiedVehicle(getLocalPlayer())
if(not running or showRespawn or (not vehicle) or reset)then return end
local mode = getElementModel(vehicle)
if(mode == 425)then return end
local lastSaved = getTickCount()
local index = #vehicleData + 1
vehicleData[index] = {}
local posX, posY, posZ = getElementPosition(vehicle)
local rotX, rotY, rotZ = getElementRotation(vehicle)
local velX, velY, velZ = getElementVelocity(vehicle)
local turnVelX, turnVelY, turnVelZ = getVehicleTurnVelocity(vehicle)
local health = getElementHealth(vehicle)
local model = getElementModel(vehicle)
-- Later add worldSpecialProperty too.
vehicleData[index].posX = posX
vehicleData[index].posY = posY
vehicleData[index].posZ = posZ
vehicleData[index].rotX = rotX
vehicleData[index].rotY = rotY
vehicleData[index].rotZ = rotZ
vehicleData[index].velX = velX
vehicleData[index].velY = velY
vehicleData[index].velZ = velZ
vehicleData[index].turnVelX = turnVelX
vehicleData[index].turnVelY = turnVelY
vehicleData[index].turnVelZ = turnVelZ
vehicleData[index].health = health
vehicleData[index].model = model
vehicleData[index].dimension = getElementDimension(vehicle)
vehicleData[index].nitro = nil
local upgrades = getVehicleUpgrades(vehicle)
for upgradeKey, upgradeValue in ipairs(upgrades) do
if(tonumber(upgradeValue) >= 1008 and tonumber(upgradeValue) <= 1010)then
vehicleData[index].nitro = tonumber(upgradeValue)
end
end
outputDebugString("Vehicle data num: "..index.." saved.")
end
saveTimer = setTimer(saveVehicleData, SAVE_INTER, 0)
function saveRespawnToSpawnpoint(player)
local vehicle = getPedOccupiedVehicle(player)
local index = #vehicleData + 1
vehicleData[index] = {}
local spawn = getElementsByType("spawnpoint")
local posX, posY, posZ = getElementData(spawn[1], "posX"), getElementData(spawn[1], "posY"), getElementData(spawn[1], "posZ")
local rotX, rotY, rotZ = getElementData(spawn[1], "rotX"), getElementData(spawn[1], "rotY"), getElementData(spawn[1], "rotZ")
local velX, velY, velZ = 0, 0, 0
local turnVelX, turnVelY, turnVelZ = 0, 0, 0
local health = getElementHealth(vehicle)
local model = getElementData(spawn[1], "vehicle")
-- Later add worldSpecialProperty too.
vehicleData[index].posX = posX
vehicleData[index].posY = posY
vehicleData[index].posZ = posZ
vehicleData[index].rotX = rotX
vehicleData[index].rotY = rotY
vehicleData[index].rotZ = rotZ
vehicleData[index].velX = velX
vehicleData[index].velY = velY
vehicleData[index].velZ = velZ
vehicleData[index].turnVelX = turnVelX
vehicleData[index].turnVelY = turnVelY
vehicleData[index].turnVelZ = turnVelZ
vehicleData[index].health = health
vehicleData[index].model = model
vehicleData[index].dimension = getElementDimension(vehicle)
end
addEvent("onClientRaceStateChanging", true)
addEventHandler("onClientRaceStateChanging", getRootElement(),
function(newState, oldState)
if(newState == "Running")then
local state = tostring(getElementData(getLocalPlayer(), "state"))
if(state == "waiting")then
--[[
reset = false
running = true
vehicleData = {}
saveRespawnToSpawnpoint(getLocalPlayer())
showRespawn = true
setElementData(getLocalPlayer(), "respawn.playing", false, true)
bindKey(RESPAWN_KEY, "up", respawn)
--]]
elseif(state == "alive" or state == "not ready")then
reset = false
running = true
for i = 1, #vehicleData do
table.remove(vehicleData, i)
end
vehicleData = {}
saveVehicleData()
end
end
if(newState == "PostFinish" or newState == "NoMap")then
reset = true
running = false
unbindKey(RESPAWN_KEY, "up", respawn)
showRespawn = false
setElementData(getLocalPlayer(), "respawn.playing", false, true)
end
end)
addEventHandler("onClientPlayerWasted", getLocalPlayer(),
function()
if(source ~= getLocalPlayer())then return end
reset = true
setElementData(getLocalPlayer(), "respawn.playing", false, true)
setTimer(function()
--local state = getElementData(getLocalPlayer(), "respawn.playing") or true
if(#vehicleData > 0 and running)then
showRespawn = true
setElementData(getLocalPlayer(), "respawn.playing", false, true)
bindKey(RESPAWN_KEY, "up", respawn)
end
end,
3000, 1)
end)
local unfreeze = {}
addEvent("clientUnfreezeOnReady", true)
addEventHandler("clientUnfreezeOnReady", getRootElement(),
function(vehicle, vehData)
-- source is the vehicle to unfreeze
unfreeze.vehicle = vehicle
unfreeze.health = vehData.health
unfreeze.dim = vehData.dimension
unfreeze.x, unfreeze.y, unfreeze.z = vehData.posX, vehData.posY, vehData.posZ
unfreeze.vx, unfreeze.vy, unfreeze.vz = vehData.velX, vehData.velY, vehData.velZ
unfreeze.tx, unfreeze.ty, unfreeze.tz = vehData.turnVelX, vehData.turnVelY, vehData.turnVelZ
setElementFrozen(vehicle, false)
addEventHandler("onClientRender", getRootElement(), unfreezeOnReady)
end)
function unfreezeOnReady()
setElementHealth(unfreeze.vehicle, unfreeze.health)
setElementPosition(unfreeze.vehicle, unfreeze.x, unfreeze.y, unfreeze.z)
if(not isElementFrozen(unfreeze.vehicle))then
setElementDimension(unfreeze.vehicle, unfreeze.dim)
setElementVelocity(unfreeze.vehicle, unfreeze.vx, unfreeze.vy, unfreeze.vz)
setVehicleTurnVelocity(unfreeze.vehicle, unfreeze.tx, unfreeze.ty, unfreeze.tz)
removeEventHandler("onClientRender", getRootElement(), unfreezeOnReady)
end
end
function dxDrawColoredText(text, left, top, right, bottom, color, scale, font, alignX, alignY, clip, wordBreak, postGUI)
-- Making them optional.
right = right or screen_width
bottom = bottom or screen_height
while(left < 0) do
left = screen_width - math.abs(left)
end
while(top < 0) do
top = screen_height - math.abs(top)
end
while(right < 0) do
right = screen_width - math.abs(right)
end
-
Hmm how to do that? :l
-
Hmm I did it with the map related things. Didn't help. Just as I thought since they do show up with the admin panel
-
What should I replace? I can't just replace everything. That'll fuck up my server.
-
They do show in the admin panel. Mapmanager is running. It doesn't say anything about it in the debug. Still they don't show up.
-
Hey all. I've got a race server but I want to make a rest/afk cornor. How can I change the gamemode to play for just one person?
-
God dammit he did it again. How can I block this?
-
Alright, thanks. But now this certain person tells me my server is illegal because it has custom maps. I have a DM/DD race server.
Is that true?
-
Hey everyone. I wanted to know if MTA is legal. I'm home hosting a server and I'm getting DDoS attacks from a certain person lately. I'm not sure if I can do anything against it since I don't know if having a home host MTA server is legal.
-
How would I use that?
-
Hey all. How can I instantly remove respawns from every map? Just disable the respawn script. How to do it? Using a racing script btw.
-
Hey all. How can I make that I can drive trough any object?
-
Using this one:
https://community.multitheftauto.com/ind ... ls&id=5801
I also tried another one, same problem. maybe my path is wrong?
-
Hey everyone. I've got another problem. My mapshop doesn't show any maps. I save my maps in here:
J:\MTA\server\mods\deathmatch\resources\[gamemodes]\[race]\[maps]
What could be the problem?
-
Awesome, thanks anderl. It has a great effect.
-
Thanks, but now my buttons are behind the img. how can I get them infront of it?
-
So instead of the width and height I just put the .img name?
-
guiCreateStaticImage
Client-Sided
I thought I had to use that but. How will I use it here?
mainWindow = guiCreateWindow(screenWidth/2-mainWidth/2,screenHeight/2-mainHeight/2,mainWidth,mainHeight,"Login panel",false)
-
Hey everyone, just one question. How can I implend an image to a gui window?
-
Guys.. Pwease??
Respawn someone without vehicle
in Scripting
Posted
So.. could anyone please help me?