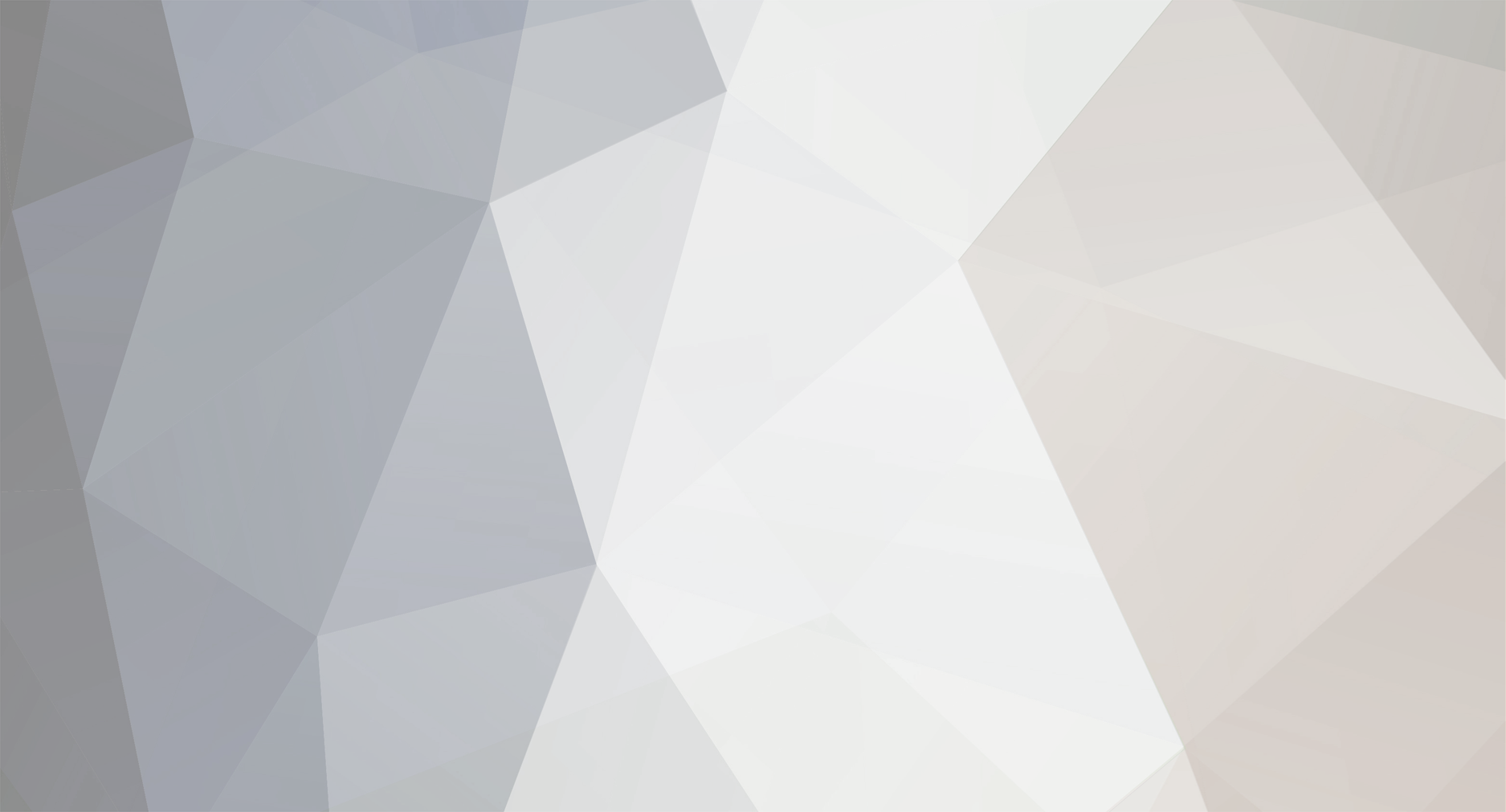
Simon54
-
Posts
11 -
Joined
-
Last visited
Posts posted by Simon54
-
-
Hello, I ran into a little problem while interpolating between GREEN and RED.
When the time value reached '500' it was suppose to start from GREEN and fade into RED until the time value reached '600'.
Problem is, it does not start at full GREEN because the time value is already '500' at the point in the function.I tried a bunch of math calculations to fix this but can't seem to figure it out. I'd appreciate any sort of help.
if (time >= 500 and time < 600) then -- local progress = (time/600) local progress = (time/100) -- time value at this point is '500' selection_color = tocolor(interpolateBetween( 0, 255, 0, 255, 0, 0, progress, "Linear")) end
-
Hello everyone! I'm trying to check if the player's account still exists after the player has logged out, due to the account being deleted.
What I did to check will only return the 'true' message even though the account was deleted.
Question is, how can I actually make this check work using the 'onPlayerLogout' event?addEventHandler( "onPlayerLogout", getRootElement( ), function( previousAccount, currentAccount ) local playerName = getPlayerName( source ) outputChatBox( playerName .. " logged out of account: '".. getAccountName( previousAccount ).."'", source ) -- info local does_previousAcc_still_exsist = getAccountName( previousAccount ) or "DELETED" if ( does_previousAcc_still_exsist ) then outputChatBox( "account: true: " .. does_previousAcc_still_exsist ) else outputChatBox( "account: false: " .. does_previousAcc_still_exsist ) -- trigger event here end end)
-
-
This is basically the idea or structure
========================================================== -- setting data theFistTable = {} -- {2, 4, 6, 8, 10} table.insert( theFistTable, 10 ) table.insert( theFistTable, 8 ) table.insert( theFistTable, 6 ) table.insert( theFistTable, 4 ) table.insert( theFistTable, 2 ) theSecondTable = {} -- {2, 4, 6, 8, 10} table.insert( theSecondTable, 2 ) table.insert( theSecondTable, 4 ) table.insert( theSecondTable, 6 ) table.insert( theSecondTable, 8 ) table.insert( theSecondTable, 10 ) setElementData( thePlayerElement, "data", theSecondTable ) ========================================================== -- matching data local data = getElementData( thePlayerElement, "data" ) for key, value in ipairs( theFirstTable ) do if data[1] == table.find( theFirstTable ) then outputChatBox("match found") end end
-
Hi, I can't seem to figure this out even after going over the Tables TUT. I have a number value that I need to match with a value inside a different table.
All is good, I'm just not sure how to loop through the other table (numberTable) to see if a value is present that does match data[1]I tried something like this:
for key, value in ipairs( numberTable ) do if data[1] == table.find( numberTable ) then outputChatBox("match found") end end
-
1 hour ago, Tekken said:
The "table way" is actually pretty straight forward, here is a simple example:
local guiStuff = {}; --create the table. guiStuff[ #guiStuff + 1 ] = guiCreateStaticImage("IMAGE1", x, y); --#guiStuff > gets the total number of items in the table and we add one so basically we do [0 + 1] then [1 + 1], [2 + 1] and so on.. guiStuff[ #guiStuff + 1 ] = guiCreateStaticImage("IMAGE2", x, y); guiStuff[ #guiStuff + 1 ] = guiCreateStaticImage("IMAGE3", x, y); guiStuff[ #guiStuff + 1 ] = guiCreateStaticImage("IMAGE4", x, y); --so on as many times you want.... for index = 1, #guiStuff do guiSetVisible(guiStuff[index], true); end --you can also acces any of the above like this guiSetVisible(guiStuff[3], false); --if you are sure of the index --imagine the table like this: local guiStuff = { [1] = guiCreateStaticImage("IMAGE1", x, y), [2] = guiCreateStaticImage("IMAGE2", x, y), [3] = guiCreateStaticImage("IMAGE3", x, y), [4] = guiCreateStaticImage("IMAGE4", x, y), };
Okay perfect, this is also a great example, easy to understand. Thanks Tekken I really appreciate the help!
-
Thanks IIYAMA, the dirty way does what I need it to do and it really saved a ton of lines in the code. I am however using it more than once in the same script, I think as long as it doesn't cause any major performance or security implications it should be okay until I figure out how to go about doing it the table way. Thanks again.
-
1
-
-
Hi, I have about 50 or more gui-images to make visible / invisible but im getting all errors - @'guiSetVisible' expected element at argument 1, got string "button_1_img"
I need to use a loop to concat and change button numbers 1-50. How to actually make this work?-- button elements button_1_img = guiCreateStaticImage ( 500, 500, 32, 32, "img/buttons/button_select.png", false, main_window ) button_2_img = guiCreateStaticImage ( 550, 500, 32, 32, "img/buttons/button_select.png", false, main_window ) button_3_img = guiCreateStaticImage ( 600, 500, 32, 32, "img/buttons/button_select.png", false, main_window ) button_4_img = guiCreateStaticImage ( 650, 500, 32, 32, "img/buttons/button_select.png", false, main_window ) button_5_img = guiCreateStaticImage ( 700, 500, 32, 32, "img/buttons/button_select.png", false, main_window ) -- + 45 for index=1,50 do guiSetVisible ( "button_"..index.."_img", true ) end
-
Thank you guys!
-
Hello Community. I need a bit of help with sorting some numbers inside a table starting from the lowest value to the highest.
The numbers inserted into the table will be math.random but for this exercise we're using numbers: 2, 4, 6, 8 and 10.
My question is, with the following example down below is there a simple way to make them output in the order of: 2, 4, 6, 8, 10 instead of: 10, 8, 2, 6, 4 ?
numTable = {} -- insert table.insert(numTable, 10) table.insert(numTable, 8) table.insert(numTable, 2) table.insert(numTable, 6) table.insert(numTable, 4) -- sort -- output outputChatBox(tostring(numTable[1])..", "..tostring(numTable[2])..", "..tostring(numTable[3])..", "..tostring(numTable[4])..", "..tostring(numTable[5]))
(Help) Interpolate / Progress
in Scripting
Posted
Thank you man, I appreciate