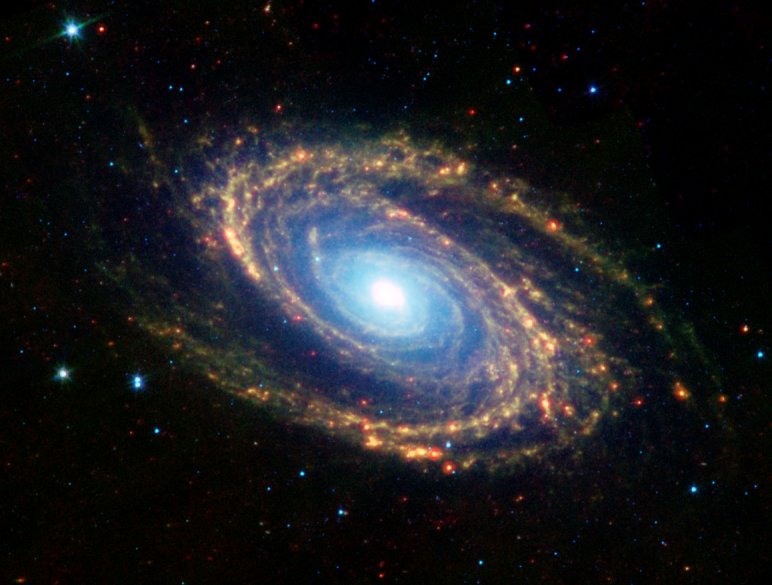
Craigster
-
Posts
5 -
Joined
-
Last visited
Posts posted by Craigster
-
-
Line 88, change the loop to ipairs.
-
1
-
-
local visible = false local stream = false local availableRadios = false local search = false local sound = false local ls, rs = false local x, y = guiGetScreenSize() local sx, sy = x/1440, y/900 local faded = true local user_settings = {radio_id = nil} ------------------------------------------------------------------------------------------------------------------------- local radio_categories = { [1] = { name = "Bulgaria", abbreviation = "BG" }, [2] = { name = "Russia", abbreviation = "RU" }, [3] = { name = "Czech Republic", abbreviation = "CZ" }, [4] = { name = "Slovakia", abbreviation = ""}, [5] = { name = "Poland", abbreviation = "PL"} } ------------------------------------------------------------------------------------------------------------------------- local radios = { [1] = { { name = "Radio Fresh", url = "http://193.108.24.21:8000/fresh_low"}, { name = "Radio The Voice", url = "http://31.13.223.148:8000/thevoice.mp3"}, { name = "Radio City", url = "http://149.13.0.81:80/city64" }, { name = "Radio FM+", url = "http://193.108.24.21:8000/fmplus"}, { name = "Radio N-Joy", url = "http://live.btvradio.bg/njoy.mp3" }, { name = "Radio Melody", url = "http://live.btvradio.bg/melody.mp3"}, { name = "Radio NRJ", url = "http://149.13.0.81:80/nrj128" }, { name = "Radio Star FM", url = "http://pulsar.atlantis.bg:8000/starfm.m3u"}, { name = "Z-Rock Radio", url = "http://live.btvradio.bg/z-rock.mp3"}, { name = "BG Radio", url = "http://149.13.0.81:80/bgradio128"}, { name = "Darik Radio", url = "http://darikradio.by.host.bg:8000/S2-128.m3u" }, { name = "Radio Focus", url = "http://online.focus-radio.net:8100/sofia"}, { name = "Radio Magic FM", url = "http://31.13.223.148:8000/magicfm.mp3"}, { name = "Radio Jazz FM", url = "http://46.10.150.123:80/jazz-fm.mp3"}, { name = "Radio BNR Horizont", url = "http://stream.bnr.bg:8002/horizont.mp3"}, }, [2] = { { name = "Radio Europa Plus", url = "http://ep128server.streamr.ru:8030/ep12" }, { name = "Radio Record", url = ""}, { name = "Trancemission", url = ""}, { name = "Record EDM", url = ""}, { name = "Pirate Station", url = ""}, { name = "VIP House", url = ""}, { name = "Record Hardstyle", url = ""}, { name = "Record Dancecore", url = ""}, { name = "Record Breaks", url = ""}, { name = "Record Chill-Out", url = ""}, { name = "Record Dubstep", url = ""}, { name = "Super Disco 90-х", url = ""}, { name = "Radio Pump", url = ""}, { name = "Radio Medliak FM", url = ""}, { name = "Radio Gop FM", url = ""}, { name = "Radio Russian Mix", url = ""}, { name = "Radio Yo! FM", url = ""}, }, [3] = { {name = "Radio Evropa 2", url = "http://icecast4.play.cz:80/evropa2-64.mp3" }, }, [4] = { {name = "Radio Expres", url = "http://85.248.7.162:8000/64.mp3" }, }, [5] = { {name = "Radio Party", url = "http://s3.radioparty.pl:8000/rp.mp3" }, } } local radio_indexing = {} ------------------------------------------------------------------------------------------------------------------------- function createGUI() local radioname = false local radiolocation = false radioPanel = guiCreateWindow(x/2-345/2, y/2-410/2, 345, 410, "Online Radio (R)", false) availableRadios = guiCreateGridList(20, 50, 300, 300, false, radioPanel) guiGridListSetSortingEnabled(availableRadios, false) local column1 = guiGridListAddColumn(availableRadios, "Radio name:", 0.6) local column2 = guiGridListAddColumn(availableRadios, "Radio location:", 0.3) local playbtn = guiCreateButton(19, 355, 150, 50, "Play Radio", false, radioPanel) local stopbtn = guiCreateButton(171, 355, 150, 50, "Stop Radio", false, radioPanel) search = guiCreateEdit(22, 21, 299, 26, "", false, radioPanel) -- Load the radios from the main table(temp) and reference them from the index. for category_id, category in pairs(radios) do local category_name = getRadioCategoryNameById(category_id) for radio_id, radio in ipairs(category) do local radio_name = "N/A" if (radio.name) then radio_name = radio.name; end -- Permanent id local id = #radio_indexing + 1 radio_indexing[id] = {__index = radios[category_id][radio_id], __newindex = radios[category_id][radio_id]} setmetatable(radio_indexing[id], radio_indexing[id]) radios[category_id][radio_id].id = id; radios[category_id][radio_id].cat_id = category_id; local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, radio_name, false, false) guiGridListSetItemText(availableRadios, row, column2, category_name, false, false) guiGridListSetItemData(availableRadios, row, column1, id) end end --[[ for id = 1, #BG do local radio = BG[id] local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, radio, false, false) guiGridListSetItemText(availableRadios, row, column2, "Bulgaria", false, false) end local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, "", false, false) guiGridListSetItemText(availableRadios, row, column2, "", false, false) for id = 1, #RU do local radio = RU[id] local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, radio, false, false) guiGridListSetItemText(availableRadios, row, column2, "Russia", false, false) end local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, "", false, false) guiGridListSetItemText(availableRadios, row, column2, "", false, false) for id = 1, #CZ do local radio = CZ[id] local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, radio, false, false) guiGridListSetItemText(availableRadios, row, column2, "Czech Republic", false, false) end local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, "", false, false) guiGridListSetItemText(availableRadios, row, column2, "", false, false) for id = 1, #SK do local radio = SK[id] local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, radio, false, false) guiGridListSetItemText(availableRadios, row, column2, "Slovakia", false, false) end local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, "", false, false) guiGridListSetItemText(availableRadios, row, column2, "", false, false) for id = 1, #PL do local radio = PL[id] local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, column1, radio, false, false) guiGridListSetItemText(availableRadios, row, column2, "Poland", false, false) end ---]] if (visible) then showCursor(true) end guiSetVisible(radioPanel, visible) addEventHandler("onClientGUIClick", stopbtn, radioList, false) addEventHandler("onClientGUIClick", playbtn, radioList, false) addEventHandler("onClientGUIDoubleClick", availableRadios, radioList, false) addEventHandler("onClientGUIChanged", search, onSearchBarChanged) addEventHandler("onClientGUIClick", stopbtn, stopRadio, false) addEventHandler("onClientGUIClick", playbtn, playRadio, false) addEventHandler("onClientGUIDoubleClick", availableRadios, playRadio, false) if isEventHandlerAdded("onClientRender", root, drawNoSoundText) == false then addEventHandler("onClientRender", root, drawNoSoundText) end end addEventHandler("onClientResourceStart", resourceRoot, createGUI) function retrieveAllRadiosFromIndex(r_index) guiGridListClear(availableRadios) local r_index = r_index or radio_indexing for k, v in ipairs(r_index) do if (r_index[k].id) then local id = r_index[k].id local radio = r_index[k] local category_name = getRadioCategoryNameById(radio.cat_id) local radio_name = "N/A" if (radio.name) then radio_name = radio.name; end local row = guiGridListAddRow(availableRadios) guiGridListSetItemText(availableRadios, row, 1, radio_name, false, false) guiGridListSetItemText(availableRadios, row, 2, category_name, false, false) guiGridListSetItemData(availableRadios, row, 1, id) end end end function searchForRadioNameFromIndex(name) if not (name) then return end local radio_indexes = {} for k, v in pairs(radio_indexing) do local radio = radio_indexing[k] if (radio.name) then local radio_name = string.lower(radio.name) local name = string.lower(name) if string.find(radio_name, name, 1) then table.insert(radio_indexes, radio); end end end return radio_indexes end function getRadioCategoryNameById(id) if (id) then if (radio_categories[id] and radio_categories[id].name) then return radio_categories[id].name; end end return "N/A"; end function getRadioByPermId(perm_id) if not (perm_id) then return end return radio_indexing[perm_id]; end function getRadioURLByPermId(perm_id) if (perm_id) then local radio = getRadioByPermId(perm_id) if (radio) and (radio.url) then return radio.url, true end end return "N/A", nil end ------------------------------------------------------------------------------------------------------------------------- function stopRadio() if sound then stopSound(sound) sound = nil end removeEventHandler("onClientRender", root, drawSoundBars) removeEventHandler("onClientRender", root, drawRadioName) if isEventHandlerAdded("onClientRender", root, drawNoSoundText) == false then addEventHandler("onClientRender", root, drawNoSoundText) end end ------------------------------------------------------------------------------------------------------------------------- function playRadio() stopRadio() refreshRadio() removeEventHandler("onClientRender", root, drawNoSoundText) if isEventHandlerAdded("onClientRender", root, drawSoundBars) == false then addEventHandler("onClientRender", root, drawSoundBars) end if isEventHandlerAdded("onClientRender", root, drawRadioName) == false then addEventHandler("onClientRender", root, drawRadioName) end end ------------------------------------------------------------------------------------------------------------------------- function refreshRadio() if sound then stopSound(sound) sound = nil end local id = user_settings.radio_id if (id) then local url, bool = getRadioURLByPermId(id) if (url and bool) then sound = playSound(url) if isElement(sound) then setSoundVolume(sound, 0.5) end end end end ------------------------------------------------------------------------------------------------------------------------- function openRadioGUI() if (guiGetVisible(radioPanel) == false) then guiSetVisible(radioPanel, true) showCursor(true) else guiSetVisible(radioPanel, false) showCursor(false) end end bindKey( "R", "down", openRadioGUI) ------------------------------------------------------------------------------------------------------------------------- function isEventHandlerAdded(sEventName, pElementAttachedTo, func) if type(sEventName) == 'string' and isElement(pElementAttachedTo) and type(func) == 'function' then local aAttachedFunctions = getEventHandlers(sEventName, pElementAttachedTo) if type(aAttachedFunctions) == 'table' and #aAttachedFunctions > 0 then for i, v in ipairs(aAttachedFunctions) do if v == func then return true end end end end return false end ------------------------------------------------------------------------------------------------------------------------- function drawRadioName() local radioName = guiGridListGetItemText(availableRadios, guiGridListGetSelectedItem(availableRadios), 1) if not faded then return end if isPlayerMapVisible() then return end dxDrawText(radioName, x*0.01302083+1, y*0.9713+1, x*0.2546875+1, y*1+1, tocolor(0, 0, 0, 255), 0.9*sy, "bankgothic", "center", "center", false, false, false, false, false) dxDrawText(radioName, x*0.01302083, y*0.9713, x*0.2546875, y*1, tocolor(255, 255, 255, 255), 0.9*sy, "bankgothic", "center", "center", false, false, false, false, false) end ------------------------------------------------------------------------------------------------------------------------- function drawNoSoundText() if not faded then return end if isPlayerMapVisible() then return end dxDrawText("Radio Off", x*0.01302083+1, y*0.9713+1, x*0.2546875+1, y*1+1, tocolor(0, 0, 0, 255), 0.9*sy, "bankgothic", "center", "center", false, false, false, false, false) dxDrawText("Radio Off", x*0.01302083, y*0.9713, x*0.2546875, y*1, tocolor(255, 255, 255, 255), 0.9*sy, "bankgothic", "center", "center", false, false, false, false, false) end ------------------------------------------------------------------------------------------------------------------------- function drawSoundBars() if sound then ls, rs = getSoundLevelData(sound) end if (ls ~= false) then if not faded then return end if isPlayerMapVisible() then return end dxDrawRectangle(x*0, y*1, x*0.004583, y*0.11852*(ls/-230768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.00583, y*1, x*0.004583, y*0.11852*(ls/-150768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.01167, y*1, x*0.004583, y*0.11852*(ls/-190768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.0175, y*1, x*0.004583, y*0.11852*(ls/-90768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.023, y*1, x*0.004583, y*0.11852*(ls/-120768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.029167, y*1, x*0.004583, y*0.11852*(ls/-60768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.035, y*1, x*0.004583, y*0.11852*(ls/-90768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.04083, y*1, x*0.004583, y*0.11852*(rs/-75768), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.0471875, y*1, x*0.004583, y*0.11852*(ls/-132571), tocolor(0, 128, 255, 255)) dxDrawRectangle(x*0.0525, y*1, x*0.004583, y*0.11852*(ls/-182571), tocolor(0, 128, 255, 255)) end end ------------------------------------------------------------------------------------------------------------------------- addEventHandler('onClientScreenFadedIn', root, function() faded = true end) addEventHandler('onClientScreenFadedOut', root, function() faded = false end) ------------------------------------------------------------------------------------------------------------------------- function onSearchBarChanged() if not isElement(source) then return end local newText = guiGetText(source) if (newText ~= "") then return retrieveAllRadiosFromIndex(searchForRadioNameFromIndex(newText)); else retrieveAllRadiosFromIndex() end end ------------------------------------------------------------------------------------------------------------------------- function radioList(btn, state) if (state ~= "up") then return end local row, col = guiGridListGetSelectedItem(availableRadios) local perm_id = guiGridListGetItemData(availableRadios, row, 1) perm_id = tonumber(perm_id) if (perm_id) then local url = getRadioURLByPermId(perm_id) if (url) then user_settings["radio_id"] = perm_id else user_settings["radio_id"] = nil end end end
Here you go, I've re-organized your 'table'. You can now add the rest of the URLs there. Line 211-229, also added the search functionality for you, rest is the same as usual.
Happy holidays buddy, enjoy!
-
1
-
-
Yup.
-
Same has happened to me previously, I can surely say for experience that the exports function inflicts when you input a function value. One of the reasons can be because everytime you send the function, it won't make a reference to the original function, acting like a sandbox within resources, but also indeed, create a new one hence making it excessively usage to the memory.
[Question] cancell antiafk to a zone
in Scripting
Posted
I've quickly and rapidly created a base script for you to start to work on, untested. But assuming that the array has the correct values, and assuming you have a seperate AFK script. You will need to integrate it within the script. Which you will need to do so by exporting the function: isElementWithinAFKZone.
I would also suggest to have the 'no damage' client-sided, but is not necessary, as it reduces the server-stress.