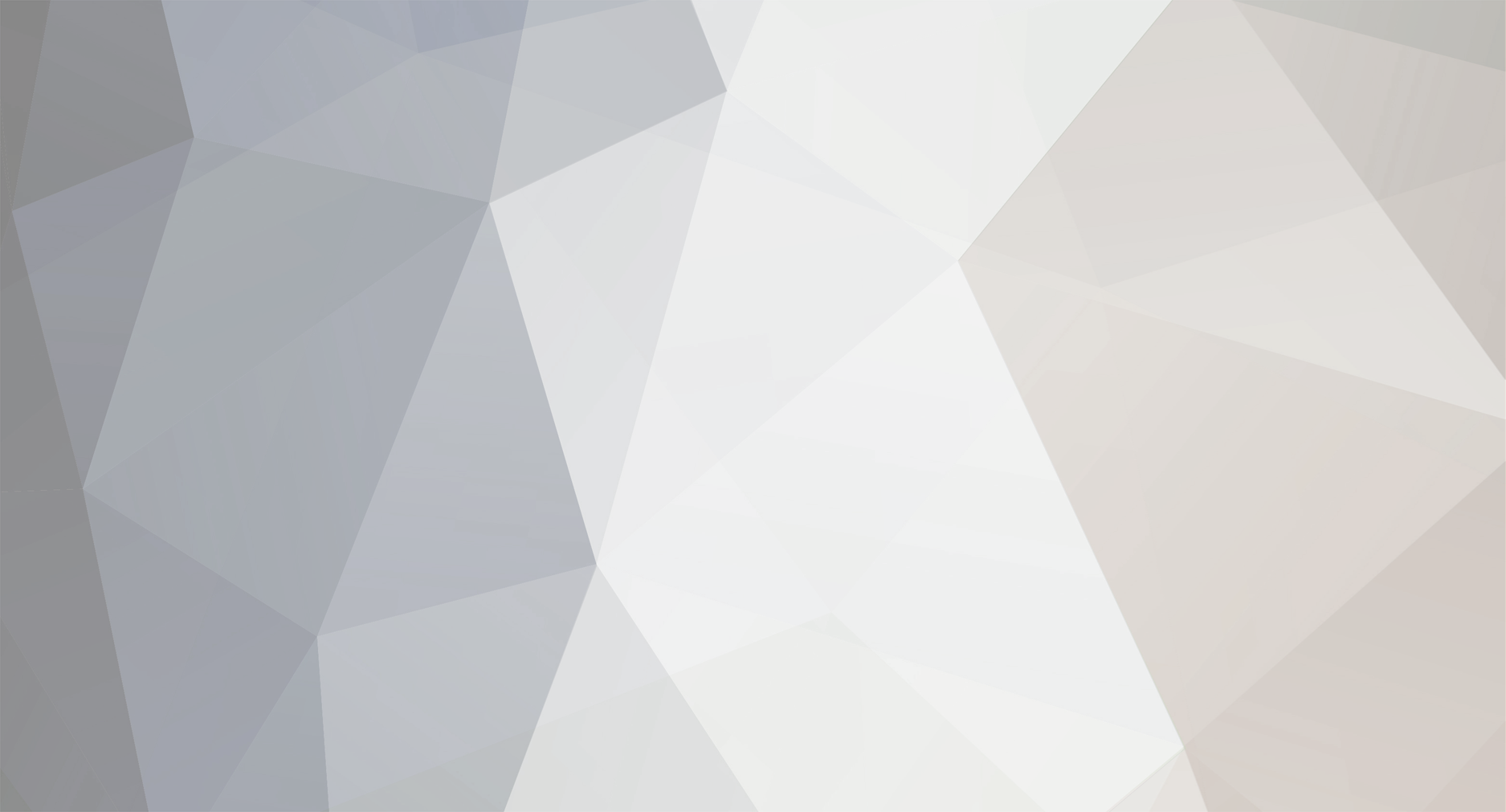
szlend
-
Posts
58 -
Joined
-
Last visited
Posts posted by szlend
-
-
Just made a complete list of San Andreas hidden interiors which contains the name, interior id and location.
You can get it here: http://pastebin.com/f4f45a8d1
I didn't test all the interiors but those that I did, work. I've gathered the locations from here. Would be nice if someone could make a wiki page for hidden interiors.
-
hm... the lines look like the light system in those singleplayer discos that's definitvely a nice feature =)
Offtopic:
ARGH look at the chat... I thought another funny superweapon would be a carpet bomb and started coding one but now i have to see that you already already the same idea...
lmao i had the same idea. wrong image btw ;P
-
I had similar problems too.
ERROR: Database query failed: near ""It's funny because i didn't even have that symbol in my SQL command.
-
It has nothing to do with the race gamemode. It happens in every gamemode and it pisses me because i have to tap F like mad so the player finally get's out of the car...
-
1) I wouldn't say Lua's syntax is very similar to C++.
Lua:
something = 1 if something == 1 then doSomething() else doSomethingElse() end
C++
int something = 1; if (something == 1) { doSomething(); } else { doSomethingElse(); }
2) Server-side scripts are executed on the server while client-side scripts are downloaded for the client and executed locally. Some functions like GUI stuff can be used client-side only.
3) You can use any script on any server as long as you have admin access to it.
4) I'm not completely sure what you mean but you can't execute a script if you don't have admin access at the server.
Edit: Dang, Ace_Gambit was faster ;P
-
-
http://development.mtasa.com/index.php?title=MoveObject
Look at examples at the bottom. You can restrict a command in acl.xml
-
As much as i know cancelEvent just cancels the original event so there's nothing wrong with that. And about my code, I just wrote an example of how to do it. I never tested it. So DSC-L|Gr00vE[Ger], just debug it dammit...
-
Also noticed this:
function Money( hitElement, matchingDimension) if ( getElementType ( hitElement ) == "player" ) then timers[ source ] = setTimer( givePlayerMoney, 1000, 0, hitElement, 10 ) end end
Should be:
function Money( hitElement, matchingDimension) if ( getElementType ( hitElement ) == "player" ) then timers[ hitElement ] = setTimer( givePlayerMoney, 1000, 0, hitElement, 10 ) end end
And read what 50p said. Why use a ColShape at all?
-
Read: http://development.mtasa.com/index.php? ... ShapeLeave
function money_stop () killTimer( timers[ source ] ) end
You are killing the timer for ColShape not the player which is wrong. It should look like this:
function money_stop (leaveElement, matchingDimension) if ( getElementType ( leaveElement ) == "player" ) then -- not really needed. just to prevent killing non-existing timers killTimer( timers[ leaveElement ] ) end end
-
the killTimer just kills the "timer" for the last player. I think the easiest way to work around this is to make the script client side.
-
well i couldn't find a function that is called giveMoney http://development.mtasa.com/index.php?search=giveMoney&fulltext=Search
and i always used this and it works fine http://development.mtasa.com/index.php?title=GivePlayerMoney
hm... and for the marker you're right the hitElement is the marker i have never checked it but someone here in this thread said that hitElement is the player^^
That's because he's using onColShapeHit event not OnPlayerMarkerHit
-
You can only give money to players. Maybe there's another element in that sphere. Try this:
function Money( hitElement, matchingDimension) if ( getElementType ( hitElement ) == "player" ) then timer = setTimer( givePlayerMoney, 1000, 0, hitElement, 10 ) end end
-
Well you should give credits to the real author.
-
Did you really make this?
-
Why the hell do you have 2x "end" in the function? And the variable "localvehicle" in "setElementAlpha ( localvehicle, tonumber ( level ))" isn't even defined....
Are you sure you've coded before?
I seriously doubt you would forget basic stuff as syntax ...
-
Frist, this code should execute when a player joins a server like this: http://development.mtasa.com/index.php? ... PlayerJoin
And second, You need to specify a player element when calling the spawnPlayer function. Like: spawnPlayer ( source, posX, posY, posZ )
-
Why not try debugging (debugscript 3) and post warnings, errors
-
Parameters for functions are:
- oldnick
- newnick
and source returned is the player who changed his nick.
This is not a function! This is an Event! The way function works is different to the way event works. Function gets attached to event.
With onClientChangeNick(source, oldnick, newnick), I wanted to show which parameters are used by "onClientChangeNick" event. I didn't say you should call function onClientChangeNick
-
Uhm you wont spawn just by making a map. You'll have to code a script that reads data from your map file ...
Here's a nice example:
<map> <spawnpoint id="spawnpoint1" posX="1959.5487060547" posY="-1714.4613037109" posZ="877.25219726563" rot="63.350006103516" model="0"/> <pickup id="Armor 1" posX="1911.083984375" posY="-1658.8798828125" posZ="885.40216064453" type="armor" health="50" respawn="60000"/> <flag posX="1959.5487060547" posY="-1714.4613037109" posZ="877.25219726563" team="blue" /> ... </map>
local flagElements = getElementsByType ( "flag" ) -- loop through them for key, value in pairs(flagElements) do -- get our info local posX = getElementData ( value, "posX" ) local posY = getElementData ( value, "posY" ) local posZ = getElementData ( value, "posZ" ) local team = getElementData ( value, "team" ) -- create an object according to the flag position createObject ( 1337, posX, posY, posZ ) -- output the team that we created a base for outputChatBox ( "Base for team " .. team .. " created" ) end
-
-
function setPlayerTextColor(source, commandName, playerName, colR, colG, colB) player = getPlayerFromNick(playerName) setElementData(player, "colR", colR) setElementData(player, "colG", colG) setElementData(player, "colB", colB) end function onPlayerChat(message, messageType) if messageType == 0 then local playerName = getClientName(source) local colR = getElementData(source, "colR") local colG = getElementData(source, "colG") local colB = getElementData(source, "colB") outputChatBox(playerName .. ": " .. message, getRootElement(), colR, colG, colB) cancelEvent() end end function setData() players = getElementsByType("player") setElementData(players, "colR", 0) setElementData(players, "colG", 0) setElementData(players, "colB", 0) end addEventHandler("onPlayerChat", getRootElement(), onPlayerChat) addEventHandler("onPlayerJoin", getRootElement(), setData) addEventHandler("onResourceStart", getRootElement(), setData) addCommandHandler("setwritecollor", setPlayerTextColor)
Something like that should work. Didn't test though. The command is /setwritecollor playerName r b g
-
Read this: http://development.mtasa.com/index.php? ... entHandler
You forgot one parameter in addEventHandler. Also make sure you use this client side since "onClientResourceStart" is a client-side event.
function keybind () bindKey ( "r", "down", clientToggleGui ) end addEventHandler( "onClientResourceStart", getRootElement(), keybind )
And btw, the client-side script doesn't need the source element. And type debugscript 3 in the console. It'll show all errors in your script
-
Whoops nevermind. The example i gave you above will bind the key when you type "/keybind"
Do this instead:
add bindKey ("r", "down", clientToggleGui) to the onClientResourceStart event function
San Andreas Online: Unique mode for MTA
in Scripting
Posted
Nice! XMLGui looks sweet and I like how it communicates in packets. Really looking forward for this.
PS. Sent ya a PM